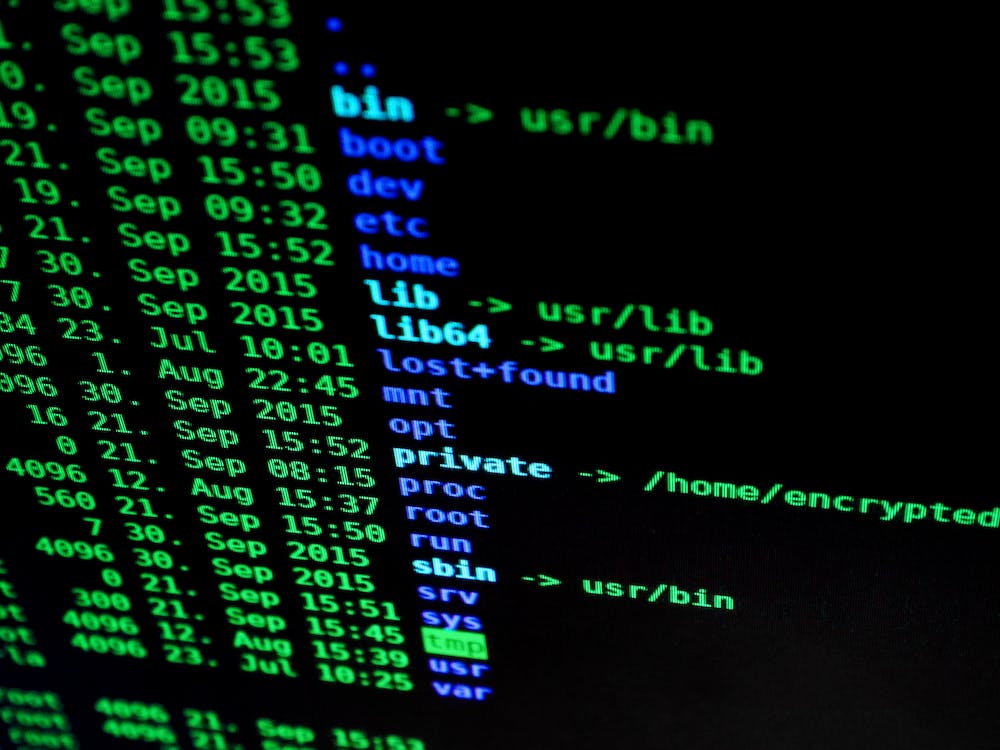
PHP is a widely used scripting language known for its flexibility and power in web development. One of the core features
of PHP is its ability to handle variables and manipulate strings effortlessly. In this article, we will uncover the shocking
secrets that occur when PHP variables meet strings, and how they can be leveraged to enhance your web development projects.
Introduction to PHP Variables
Before diving into the fascinating world of PHP variables and strings, let’s briefly understand what they are. In PHP, variables
are containers that store data, such as numbers, text, or even complex structures like arrays or objects. They are essential
for storing and managing information within a web application.
Concatenation: The Magic Uniting PHP Variables and Strings
Concatenation is the process of combining or joining multiple strings together. In PHP, concatenation plays a vital role in
working with variables and strings. IT allows us to merge PHP variables with strings to create dynamic and personalized content.
Let’s see an example to understand IT better:
<?php
$name = "John";
$message = "Hello, " . $name . "!"; // Concatenating $name variable with a string
echo $message;
?>
In this example, we have a variable named $name, which contains the value “John.” By concatenating this variable with a string,
we can create a personalized message, such as “Hello, John!” The dot (.) operator is used for concatenation in PHP.
String Interpolation: A Shortcut to Complex Concatenation
While concatenation is a powerful tool, PHP also provides a shortcut called string interpolation. IT allows us to embed variables
directly into double-quoted strings without using the dot (.) operator. Take a look at this example:
<?php
$name = "Jane";
$message = "Hello, $name!"; // Using string interpolation
echo $message;
?>
By utilizing string interpolation, we achieved the same output as before. The variable $name is directly inserted into the string,
resulting in “Hello, Jane!” This approach can simplify concatenation, especially when dealing with long strings or multiple variables.
Manipulating Strings Using PHP Functions
PHP offers a plethora of built-in functions to manipulate strings further. These functions allow developers to perform tasks like
finding the length of a string, converting cases, replacing and extracting substrings, and much more. Let’s explore a few examples:
strlen(): Finding the Length of a String
<?php
$text = "Lorem ipsum dolor sit amet";
$length = strlen($text);
echo "The length of the string is: " . $length;
?>
The strlen() function returns the length of a given string. In this example, the length of the string “Lorem ipsum dolor sit amet”
is calculated and displayed as output.
strtolower() and strtoupper(): Changing Cases
<?php
$text = "Hello, World!";
$lowercase = strtolower($text);
$uppercase = strtoupper($text);
echo "Lowercase: " . $lowercase . "<br>";
echo "Uppercase: " . $uppercase;
?>
The strtolower() and strtoupper() functions are used to convert a string to lowercase and uppercase, respectively. In this example,
the string “Hello, World!” is transformed into “hello, world!” and “HELLO, WORLD!” correspondingly.
Conclusion
PHP variables and string manipulation go hand in hand, providing developers with a wide range of possibilities to enhance their web
projects. Whether IT‘s concatenating variables and strings, utilizing string interpolation, or utilizing built-in PHP functions,
mastering these techniques can greatly improve the interactivity and user experience of your web applications.
FAQs
Q: What is the difference between single quotes and double quotes in PHP?
A: In PHP, single quotes (”) and double quotes (“”) have different behavior. Single quotes are treated literally, meaning variables
are not interpolated and special characters like backslashes (\) or single quotes (”) are displayed as is. On the other hand,
double quotes allow variable interpolation and the interpretation of certain escape sequences (\n for a new line, \t for a tab, etc.).
Q: Can I concatenate different data types with strings in PHP?
A: Yes, PHP provides automatic type conversion when concatenating different data types with strings. For example, if you concatenate
a numeric variable with a string, PHP will convert the number to a string representation and merge IT with the existing string.
Q: Are there any specific security concerns when dealing with user input in PHP variables?
A: Yes, when handling user input stored in PHP variables, IT is crucial to sanitize and validate the data to prevent security vulnerabilities
like SQL injection or cross-site scripting (XSS). Using functions like filter_var() or prepared statements can help mitigate these risks.