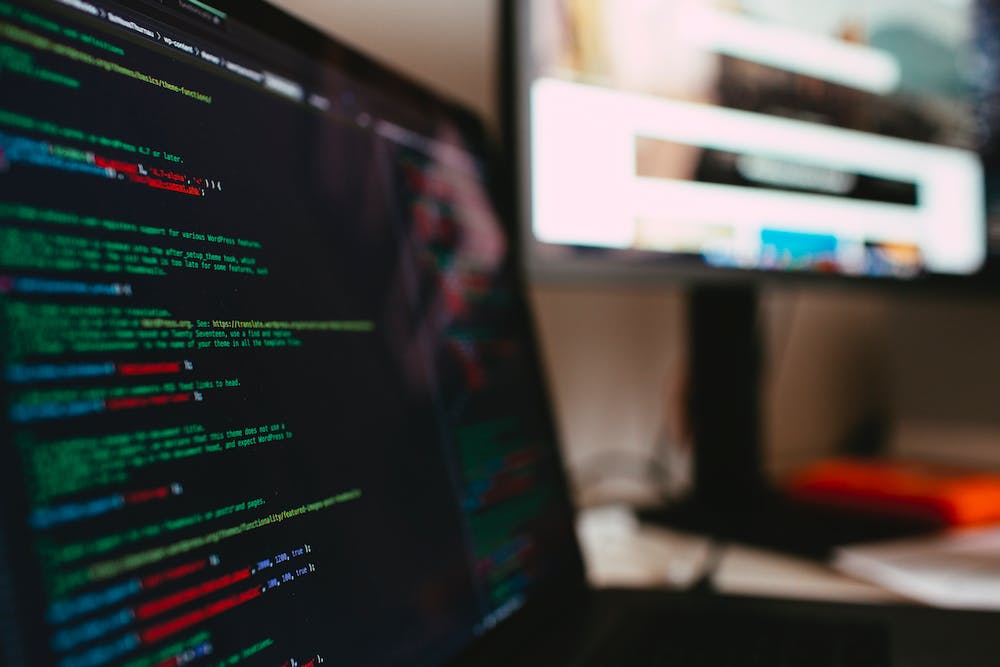
As a developer, you are always on the hunt for tools and frameworks that can make your code more efficient, readable, and maintainable. Laravel, one of the most popular PHP frameworks, has gained immense popularity among developers. One of its standout features is its array manipulation capabilities, which can truly revolutionize your code. In this article, we will explore how Laravel’s array feature can benefit your development process and why developers can’t stop raving about IT.
The Power of Laravel’s Array Feature
Laravel provides a robust set of array manipulation methods that can save you both time and effort. Let’s delve into some of the most notable features:
1. Array Filtering
Laravel offers various helper functions and methods for filtering arrays. The array_filter()
method, for example, allows you to filter an array based on a given callback. This enables you to easily remove unwanted elements from your array without using complex loops or conditional statements.
$array = [2, 4, 6, 8, 10];
$result = array_filter($array, function($value) {
return $value % 2 === 0;
});
// Result: [2, 4, 6, 8, 10]
This example demonstrates how you can effortlessly filter out odd numbers from an array using a concise callback function.
2. Array Mapping
Mapping arrays is another common task in programming. Laravel simplifies this process by providing the array_map()
method, which applies a given callback function to each element of an array and returns a new array containing the results.
$array = [1, 2, 3, 4];
$result = array_map(function($value) {
return $value * 2;
}, $array);
// Result: [2, 4, 6, 8]
The above example demonstrates how you can double each element in an array using array mapping, saving you from writing complex loops.
3. Array Sorting
Sorting arrays is a common task, and Laravel provides various methods to make this process a breeze. The array_sort()
function, for instance, allows you to sort an array by its values while maintaining the key-value associations.
$array = ['John' => 25, 'Alice' => 30, 'Bob' => 20];
$result = array_sort($array);
// Result: ['Bob' => 20, 'John' => 25, 'Alice' => 30]
The array is sorted in ascending order based on the values, but the keys remain intact. This feature is incredibly useful when dealing with associative arrays.
4. Array Access
Accessing specific elements in an array can sometimes be cumbersome, especially when nested arrays are involved. Laravel’s array_get()
method simplifies this process by allowing you to access array elements using dot notation.
$array = [
'users' => [
'John' => ['age' => 25, 'email' => '[email protected]'],
'Alice' => ['age' => 30, 'email' => '[email protected]']
]
];
$email = array_get($array, 'users.John.email');
// Result: '[email protected]'
The array_get()
method enables you to access deep array elements in a concise and readable manner, eliminating the need for multiple nested array accesses.
Developers Can’t Stop Raving About Laravel’s Array Feature
Since its introduction, Laravel’s array feature has revolutionized the way developers work with arrays and has garnered immense praise from the developer community. Here are some reasons why developers can’t stop raving about IT:
1. Enhanced Productivity
The array manipulation methods provided by Laravel significantly reduce the boilerplate code required for common array operations. This not only improves code readability but also enhances developer productivity by allowing them to focus on the core logic of their application instead of dealing with repetitive array tasks.
2. Simplified Codebase
Laravel’s array feature simplifies complex array manipulations into a few concise and expressive methods. This leads to cleaner and more maintainable code, as IT eliminates the need for lengthy loops, conditionals, and multiple nested array accesses. The resulting codebase becomes easier to understand, debug, and modify, making collaboration among developers more seamless.
3. Improved Performance
Efficient array manipulation is crucial for the performance of web applications. Laravel’s array functions are optimized for speed and memory usage, ensuring your code performs well even when dealing with large datasets. These methods are designed to minimize resource consumption and maximize execution speed, resulting in faster and more efficient code.
Conclusion
Laravel’s array feature is a game-changer for developers, offering a wide range of powerful array manipulation methods that simplify complex tasks into a few elegant lines of code. With its array filtering, mapping, sorting, and access capabilities, Laravel enables developers to write more efficient, readable, and maintainable code. By leveraging these features, you can enhance your productivity, simplify your codebase, and improve the performance of your applications.
Frequently Asked Questions
Q: Is Laravel’s array feature limited to PHP arrays?
No, Laravel’s array methods can also be used to work with collections, which are an additional feature provided by Laravel. Collections extend the functionality of arrays, allowing you to perform advanced operations such as filtering, mapping, and sorting in a more concise and object-oriented manner.
Q: Can I combine multiple array methods in Laravel?
Absolutely! Laravel’s array methods are designed to be chained together, enabling you to build complex array manipulations with ease. By chaining methods, you can create a fluent and readable syntax that performs multiple array operations in one go.
Q: Are there any performance considerations when using Laravel’s array feature?
Laravel’s array methods are highly optimized for performance. However, IT is important to note that certain operations, such as sorting large arrays, can have higher time complexity depending on the algorithm used. IT‘s recommended to benchmark your code and consider performance trade-offs when dealing with extremely large datasets.
Q: Can I use Laravel’s array methods in non-Laravel projects?
While Laravel’s array methods are primarily designed for use within Laravel projects, many of them are implemented as standalone functions and can be used in any PHP project. You can simply include the necessary Laravel framework files to access these methods independently.