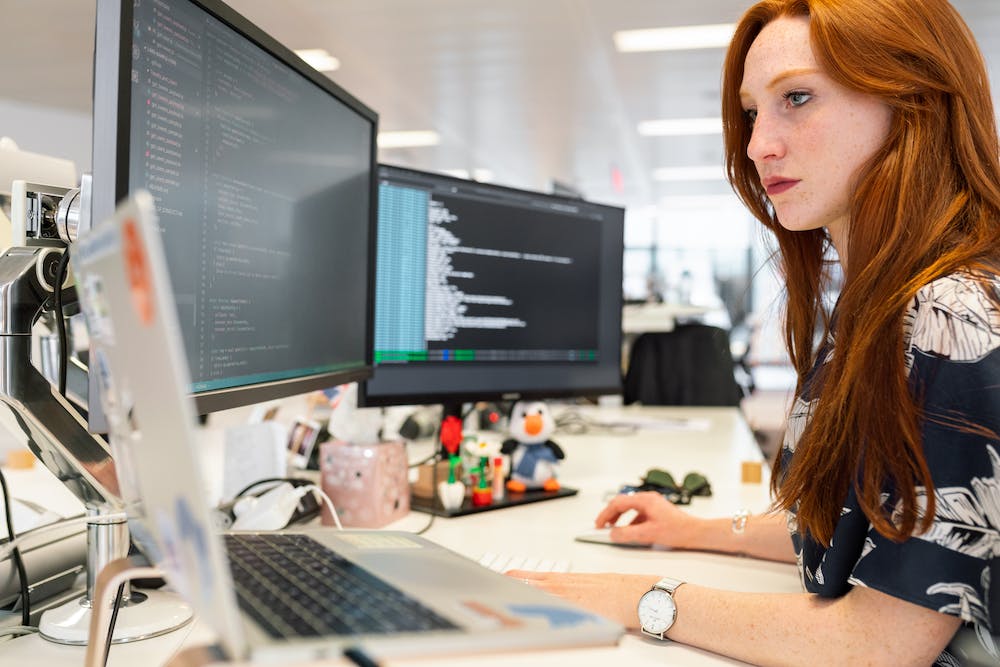
If you’re interested in computer vision and machine learning, you’ve probably heard of YOLO (You Only Look Once) object detection. YOLO is a state-of-the-art, real-time object detection system that can detect multiple objects in an image with high accuracy. In this tutorial, we’ll show you just how easy IT is to implement YOLO object detection in Python.
What is YOLO Object Detection?
YOLO is an object detection system that uses a single neural network to recognize multiple objects in an image. It can process images at an incredibly fast rate, making it suitable for real-time applications such as self-driving cars, security cameras, and augmented reality. YOLO is known for its high accuracy and efficiency, making it one of the most popular object detection systems in the field of computer vision.
Implementing YOLO in Python
Implementing YOLO in Python is surprisingly easy, thanks to the availability of pre-trained models and the user-friendly YOLOv3 implementation. In this tutorial, we’ll use the popular OpenCV library to perform YOLO object detection in Python.
Before we begin, make sure you have Python and OpenCV installed on your system. If not, you can easily install them using pip:
pip install opencv-python
Once you have OpenCV installed, you can download the pre-trained YOLOv3 weights and configuration files from the official Website. These files are necessary for performing object detection using YOLO. After downloading the files, you can load them into your Python script using OpenCV’s cv2.dnn.readNet
function:
net = cv2.dnn.readNet('yolov3.weights', 'yolov3.cfg')
Now that you have the pre-trained model loaded, you can use it to perform object detection on an image. Here’s a simple example of how to detect objects in an image using YOLO and OpenCV:
image = cv2.imread('image.jpg')
height, width = image.shape[:2]
# Create a 4D blob from the image
blob = cv2.dnn.blobFromImage(image, 1/255.0, (416, 416), swapRB=True, crop=False)
# Set the input to the network
net.setInput(blob)
# Get the output layer names
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# Run the forward pass
outs = net.forward(output_layers)
# Post-process the outputs
# ...
# Display the results
# ...
Customizing YOLO Object Detection
One of the great features of YOLO is its flexibility and ease of customization. You can easily fine-tune the YOLOv3 model to detect specific objects or classes of interest. This is done by training the model on a custom dataset using the Darknet framework. With some basic knowledge of machine learning and deep learning, you can create your own custom YOLO model to detect just about anything you can imagine.
There are also pre-trained models available for specific types of objects, such as people, cars, animals, and more. These pre-trained models can be used as a starting point for detecting specific objects in your own applications.
Optimizing YOLO for Performance
While YOLO is known for its speed and efficiency, there are still ways to optimize it for even better performance. This includes techniques such as model quantization, layer fusion, and model pruning. By applying these optimization techniques, you can make YOLO even faster and more lightweight, making it suitable for deployment on edge devices with limited computing resources.
Conclusion
Implementing YOLO object detection in Python is easier than you might think. With just a few lines of code, you can perform real-time object detection on images and videos, and even customize the model to detect specific objects. YOLO’s speed and accuracy make it a great choice for a wide range of computer vision applications, from security and surveillance to augmented reality and robotics. So why not give it a try and see for yourself how easy it is to implement YOLO in Python?
FAQs
Q: Can I use YOLO for real-time object detection?
A: Yes, YOLO is designed for real-time object detection and can process images at incredibly fast rates, making it suitable for real-time applications such as self-driving cars, security cameras, and augmented reality.
Q: Is YOLO easy to implement in Python?
A: Yes, implementing YOLO in Python is surprisingly easy, thanks to the availability of pre-trained models and user-friendly implementations such as OpenCV.
Q: Can I customize YOLO to detect specific objects?
A: Absolutely! YOLO is flexible and can be customized to detect specific objects or classes of interest. You can even create your own custom YOLO model to detect just about anything you can imagine.
Q: Is YOLO suitable for edge devices with limited computing resources?
A: Yes, by applying optimization techniques such as model quantization, layer fusion, and model pruning, you can make YOLO even faster and more lightweight, making it suitable for deployment on edge devices with limited computing resources.