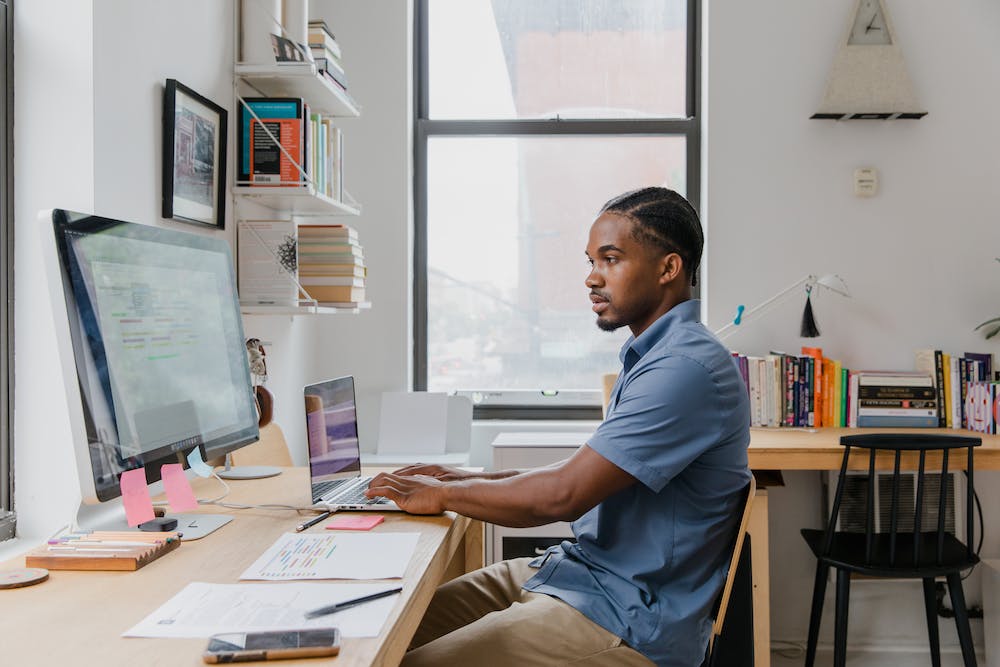
In today’s fast-paced world, handling large volumes of data efficiently is crucial for any web application. This is where Redis, an open-source in-memory data structure store, comes in. With its support for various data structures such as strings, hashes, lists, sets, and sorted sets, Redis is a popular choice for caching, session management, real-time analytics, and more. In this article, we will explore how to work with Redis in PHP, focusing specifically on key-value storage and retrieval.
Setting up Redis in PHP
Before diving into working with Redis in PHP, you’ll need to ensure that Redis is installed and running on your server. You can follow the official Redis installation guide for your specific operating system. Once Redis is up and running, you can use the php-redis
extension to interact with Redis from your PHP code.
If the php-redis
extension is not already installed, you can install IT using the following command:
sudo apt-get install php-redis # For Ubuntu/Debian
sudo yum install php-redis # For CentOS/RHEL
After installing the extension, you’ll need to restart your web server for the changes to take effect. Once the extension is successfully installed, you can start using Redis in your PHP applications.
Connecting to Redis
To connect to Redis from your PHP code, you can use the following snippet:
<?php
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
echo "Connection to server successfully";
echo "Server is running: " .$redis->ping();
?>
In this example, we create a new instance of the Redis
class and connect to the Redis server running on 127.0.0.1
on port 6379
. Running the ping()
method checks if the server is alive and returns PONG
if everything is working as expected.
Storing and Retrieving Key-Value Pairs
Once connected to the Redis server, you can start storing and retrieving key-value pairs using the set()
and get()
methods, respectively:
<?php
$redis->set('name', 'John');
$name = $redis->get('name');
echo 'Name: ' . $name;
?>
In this example, we store the key-value pair 'name'
and 'John'
using the set()
method. We then retrieve the value associated with the key 'name'
using the get()
method and print it to the screen. This simplistic example demonstrates the fundamental concept of key-value storage in Redis.
Working with Expire Times
Redis allows you to set an expiration time for key-value pairs using the setex()
method, which takes an additional parameter for the expiration time in seconds:
<?php
$redis->setex('token', 3600, 'abcd1234');
?>
In this example, we set the key 'token'
with a value of 'abcd1234'
and an expiration time of 3600 seconds (1 hour). After the specified time has elapsed, the key-value pair will be automatically removed from Redis, making it ideal for implementing short-lived cache entries or temporary data.
Using Hashes for Complex Data Structures
In addition to simple key-value pairs, Redis also supports complex data structures such as hashes. You can use the hSet()
, hGet()
, and hMGet()
methods to work with hash data structures:
<?php
$redis->hSet('user:123', 'name', 'Alice');
$redis->hSet('user:123', 'email', '[email protected]');
$name = $redis->hGet('user:123', 'name');
$email = $redis->hGet('user:123', 'email');
$userData = $redis->hMGet('user:123', ['name', 'email']);
?>
In this example, we use the hSet()
method to store the name and email of a user with the ID 123
hGet() method or retrieve multiple fields at once using the hMGet()
method. This demonstrates the flexibility and power of Redis in handling complex data structures.
Conclusion
In conclusion, working with Redis in PHP for key-value storage and retrieval is a powerful and efficient way to manage data in your web applications. By leveraging the in-memory nature of Redis, you can achieve high performance and low latency for various use cases such as caching, session management, real-time analytics, and more. With the php-redis
extension, connecting to Redis from your PHP code is straightforward, and its rich set of methods allows for easy manipulation of key-value and complex data structures. Whether you’re building a small-scale application or a large-scale system, Redis provides the tools to handle your data with ease and efficiency.
FAQs
What is Redis?
Redis is an open-source, in-memory data structure store used as a database, cache, and message broker. It supports various data structures such as strings, hashes, lists, sets, and sorted sets.
How can I install the php-redis extension?
You can install the php-redis
extension using package managers such as apt
for Ubuntu/Debian or yum
for CentOS/RHEL. After installation, you’ll need to restart your web server for the changes to take effect.
Can Redis be used for caching in PHP applications?
Yes, Redis is commonly used for caching in PHP applications due to its in-memory nature and support for various data structures. It provides high-performance caching with low latency, making it a popular choice for speeding up web applications.
Overall, Redis offers a powerful and efficient way to handle key-value storage and retrieval in PHP applications. By leveraging the features of Redis such as in-memory storage, expiration times, and complex data structures, you can enhance the performance and scalability of your web applications. With the php-redis
extension, connecting to and interacting with Redis from your PHP code is straightforward, enabling you to take full advantage of Redis’s capabilities.