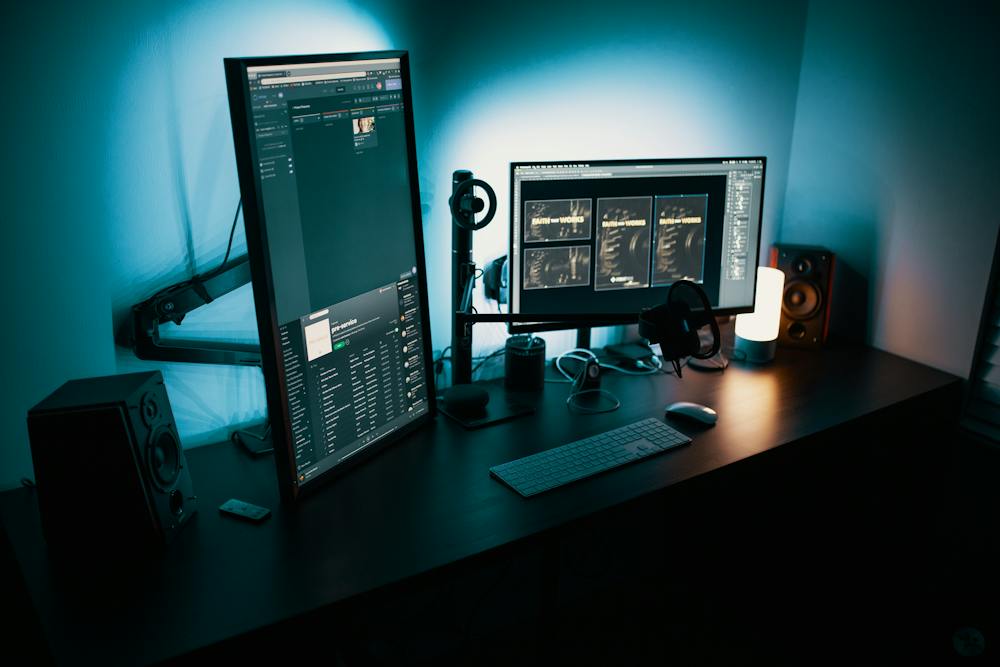
PHP is a popular server-side scripting language that is used to develop dynamic web pages and web applications. One of the key features of PHP is its string manipulation capabilities, which allow developers to work with text data in various ways. In this article, we will explore some of the essential string functions in PHP, along with examples to demonstrate their usage.
Understanding PHP Strings
In PHP, a string is a sequence of characters, which can be letters, numbers, special symbols, or whitespace. Strings are used to store and manipulate text data, such as usernames, passwords, and other textual information. PHP provides a wide range of functions for working with strings, including searching, manipulating, and formatting operations.
Essential PHP String Functions
strlen()
The strlen() function is used to get the length of a string. IT returns the number of characters in the given string. Here’s an example:
“`php
$str = “Hello, world!”;
echo strlen($str); // Output: 13
?>
“`
strpos() and strrpos()
The strpos() function is used to find the position of the first occurrence of a substring within a string. If the substring is not found, it returns false. On the other hand, strrpos() is used to find the position of the last occurrence of a substring within a string. Here are some examples:
“`php
$str = “Hello, world!”;
echo strpos($str, “world”); // Output: 7
echo strrpos($str, “o”); // Output: 8
?>
“`
substr()
The substr() function is used to extract a part of a string. It takes a string, a start index, and an optional length parameter, and returns the extracted substring. Here’s an example:
“`php
$str = “Hello, world!”;
echo substr($str, 7); // Output: world!
echo substr($str, 7, 3); // Output: wor
?>
“`
str_replace()
The str_replace() function is used to replace all occurrences of a substring within a string with another substring. It takes the string to be replaced, the replacement string, and the input string, and returns the modified string. Here’s an example:
“`php
$str = “Hello, world!”;
echo str_replace(“world”, “backlink works“, $str); // Output: Hello, Backlink Works!
?>
“`
strtolower() and strtoupper()
The strtolower() function is used to convert a string to lowercase, while strtoupper() is used to convert a string to uppercase. Here are some examples:
“`php
$str = “Hello, world!”;
echo strtolower($str); // Output: hello, world!
echo strtoupper($str); // Output: HELLO, WORLD!
?>
“`
trim()
The trim() function is used to remove whitespace (or other specified characters) from the beginning and end of a string. Here’s an example:
“`php
$str = ” Hello, world! “;
echo trim($str); // Output: Hello, world!
?>
“`
Conclusion
Working with PHP strings is an essential part of web development, and PHP provides a rich set of functions for manipulating and formatting text data. By understanding and using these string functions effectively, developers can create more dynamic and interactive web applications.
FAQs
What is the difference between strpos() and strrpos()?
The strpos() function finds the position of the first occurrence of a substring within a string, while strrpos() finds the position of the last occurrence of a substring.
Can I use string functions to manipulate non-English characters?
Yes, PHP string functions are capable of working with multi-byte characters, including non-English characters.
Are there any performance considerations when working with string functions?
While PHP string functions are generally efficient, it’s important to avoid unnecessary or excessive use of string manipulation operations, especially in large-scale applications, to maintain optimal performance.
Where can I find more information about PHP string functions?
The official PHP documentation provides comprehensive information and examples for all the built-in string functions. Additionally, there are many online resources and tutorials available for learning about PHP string manipulation.