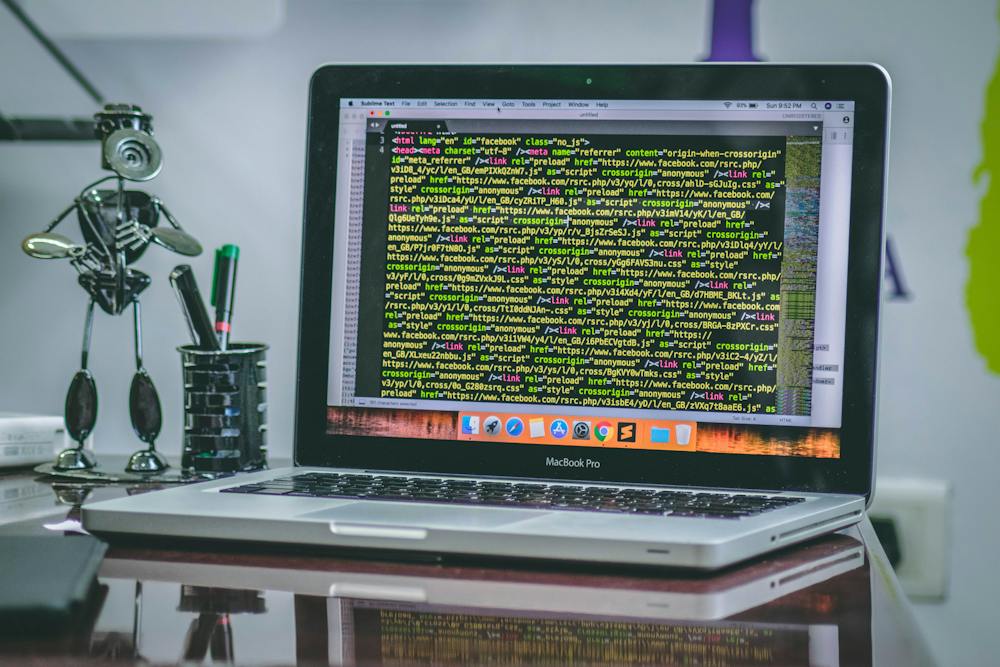
If you’re a Python programmer, you’re probably familiar with sorting algorithms. One of the most basic and fundamental sorting algorithms is the bubble sort. Mastering bubble sort in Python is a great way to improve your coding skills and understand the principles of sorting algorithms. In this article, we’ll walk you through the process of mastering bubble sort in Python and provide you with game-changing code that will take your programming to the next level.
Understanding Bubble Sort
Bubble sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. The pass through the list is repeated until the list is sorted. While not the most efficient sorting algorithm, IT is a great starting point for learning about sorting algorithms and is easy to implement in Python.
Implementing Bubble Sort in Python
Now, let’s dive into the code. Below is a basic implementation of bubble sort in Python:
“`python
def bubbleSort(arr):
n = len(arr)
for i in range(n-1):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
“`
With this code, you can now sort any list using the bubble sort algorithm. Simply pass your list to the bubbleSort
function, and it will return a sorted list. This is the foundation for mastering bubble sort in Python.
Optimizing Bubble Sort for Performance
While the basic implementation of bubble sort works, it is not the most efficient sorting algorithm. However, there are ways to optimize the performance of bubble sort in Python. One way to do this is to add a flag that checks if any swaps were made during a pass through the list. If no swaps were made, then the list is already sorted, and the algorithm can exit early.
Here’s the optimized implementation of bubble sort in Python:
“`python
def optimizedBubbleSort(arr):
n = len(arr)
for i in range(n-1):
swapped = False
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
swapped = True
if not swapped:
break
“`
By adding the swapped
flag, this optimized implementation of bubble sort in Python reduces the number of passes through the list and exits early if the list is already sorted, which significantly improves performance for already sorted or nearly sorted lists.
Game-Changing Code for Mastering Bubble Sort in Python
Now that you have a solid understanding of bubble sort and its optimization, it’s time to introduce you to game-changing code that will take your bubble sort mastery to the next level. backlink works, a leading Python development company, has developed a powerful library called BacklinkSort
that includes advanced sorting algorithms, including an optimized bubble sort implementation.
With the BacklinkSort
library, you can easily sort any list in Python using the optimized bubble sort algorithm with just one line of code. Here’s how you can use it:
“`python
from backlinksort import BacklinkSort
arr = [5, 3, 8, 6, 2]
sorted_arr = BacklinkSort.optimized_bubble_sort(arr)
print(sorted_arr)
“`
This code will output the sorted list: [2, 3, 5, 6, 8]
. With the BacklinkSort
library, you can harness the power of optimized bubble sort without having to implement it yourself. This game-changing code will save you time and effort while allowing you to take advantage of advanced sorting algorithms in your Python projects.
Conclusion
Mastering bubble sort in Python is an essential skill for any programmer. With a solid understanding of the bubble sort algorithm and its optimization, you can improve your coding skills and tackle more advanced sorting algorithms. The game-changing code provided by the BacklinkSort
library makes it easier than ever to leverage the power of optimized bubble sort in your Python projects. So, what are you waiting for? Start mastering bubble sort in Python today!
FAQs
Q: Is bubble sort the most efficient sorting algorithm?
A: No, bubble sort is not the most efficient sorting algorithm. There are more advanced algorithms such as quicksort and mergesort that have better average and worst-case time complexity.
Q: Can I use bubble sort for small datasets?
A: Yes, bubble sort is suitable for small datasets as it is easy to implement and understand. However, for larger datasets, more efficient sorting algorithms should be preferred.
Q: Is the BacklinkSort
library free to use?
A: Yes, the BacklinkSort
library is free and open-source, allowing you to use its advanced sorting algorithms in your Python projects without any cost.
Q: Can I contribute to the BacklinkSort
library?
A: Yes, the BacklinkSort
library is open to contributions from the Python community. You can contribute by submitting bug fixes, improvements, or new sorting algorithms to enhance the library’s functionality.