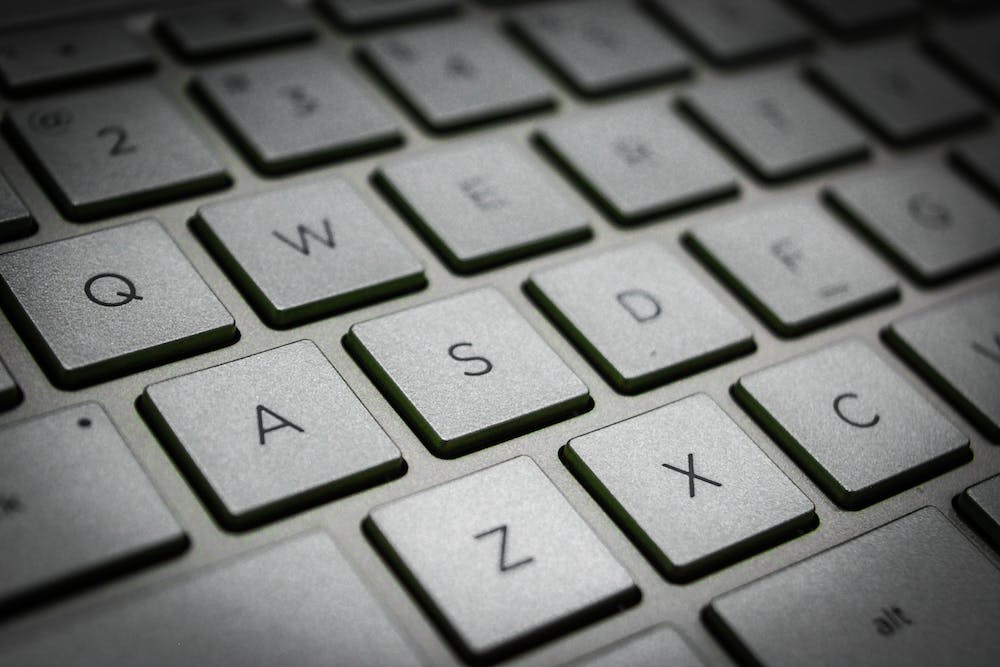
Python is a versatile programming language that is widely used for developing all kinds of applications, including graphical user interface (GUI) calculators. Building a user-friendly GUI calculator in Python can be a rewarding experience, but IT also comes with its own set of challenges. In this article, we’ll explore some proven tricks and tips to help you build a user-friendly GUI calculator in Python.
Understanding the Basics of GUI Programming in Python
Before we dive into the specifics of building a user-friendly GUI calculator in Python, it’s important to have a good understanding of the basics of GUI programming in Python. Python offers several libraries and frameworks for building GUI applications, such as Tkinter, PyQt, and wxPython. For the purpose of this article, we’ll focus on using Tkinter, which is a popular and easy-to-use library for building GUI applications in Python.
Using Tkinter to Build a User-Friendly GUI Calculator
Tkinter provides a simple and intuitive way to create GUI applications in Python. To build a user-friendly GUI calculator, you can start by designing the layout of the calculator using Tkinter’s widgets, such as labels, buttons, and entry fields. You can then define the functionality of the calculator by writing event handlers for the various buttons and input fields.
Here’s a simple example of a basic calculator GUI using Tkinter:
“`python
import tkinter as tk
def calculate():
result = eval(entry.get())
output_label.config(text=”Result: ” + str(result))
root = tk.Tk()
root.title(“Simple Calculator”)
entry = tk.Entry(root)
entry.pack()
calculate_button = tk.Button(root, text=”Calculate”, command=calculate)
calculate_button.pack()
output_label = tk.Label(root, text=””)
output_label.pack()
root.mainloop()
“`
In this example, we create a simple calculator GUI with an entry field for input, a “Calculate” button, and a label to display the result. When the “Calculate” button is clicked, the calculate function is called, which evaluates the expression entered in the entry field and displays the result in the output label.
Adding User-Friendly Features to the GUI Calculator
While the basic calculator GUI serves its purpose, adding some user-friendly features can greatly enhance the overall user experience. Here are some proven tricks and tips to make your GUI calculator more user-friendly:
1. Adding Clear and Backspace Buttons
Adding clear and backspace buttons can make it easier for users to correct any mistakes in their input. You can create clear and backspace buttons using Tkinter’s Button widget and define event handlers to clear the input field or remove the last character, respectively.
“`python
clear_button = tk.Button(root, text=”Clear”, command=lambda: entry.delete(0, tk.END))
clear_button.pack()
backspace_button = tk.Button(root, text=”Backspace”, command=lambda: entry.delete(len(entry.get())-1, tk.END))
backspace_button.pack()
“`
2. Improving the Layout and Design
Aesthetics play a crucial role in the user-friendliness of any GUI application. You can improve the layout and design of your calculator by using Tkinter’s grid, pack, or place managers to arrange the widgets in a visually appealing manner. You can also use custom fonts, colors, and styles to make your calculator more visually appealing.
“`python
entry.config(font=(“Arial”, 12))
calculate_button.config(font=(“Arial”, 12))
clear_button.config(font=(“Arial”, 12))
backspace_button.config(font=(“Arial”, 12))
output_label.config(font=(“Arial”, 12))
“`
3. Handling Errors Gracefully
It’s important to handle errors gracefully to provide a more seamless user experience. You can use try-except blocks to catch and handle any errors that may occur during the calculation process, and display helpful error messages to the user.
“`python
try:
result = eval(entry.get())
output_label.config(text=”Result: ” + str(result))
except Exception as e:
output_label.config(text=”Error: ” + str(e))
“`
Conclusion
Building a user-friendly GUI calculator in Python can be a fun and rewarding experience, especially when you incorporate proven tricks and tips to enhance the overall user experience. By using Tkinter to create the GUI and adding user-friendly features such as clear and backspace buttons, improving the layout and design, and handling errors gracefully, you can create a calculator that is both easy to use and visually appealing. With the right approach and attention to detail, you can build a user-friendly GUI calculator that users will love to use.
FAQs
Q: Can I use other libraries or frameworks to build a GUI calculator in Python?
A: Yes, you can use other libraries and frameworks such as PyQt or wxPython to build a GUI calculator in Python. The choice of library or framework depends on your specific requirements and preferences.
Q: How can I test the user-friendliness of my GUI calculator?
A: You can test the user-friendliness of your GUI calculator by conducting usability testing with real users. Observe how users interact with the calculator and gather feedback to identify any areas for improvement.
Q: Are there any resources or tutorials available for building GUI calculators in Python?
A: Yes, there are plenty of resources and tutorials available online that can help you learn how to build GUI calculators in Python. You can find step-by-step guides, code examples, and video tutorials to help you get started.