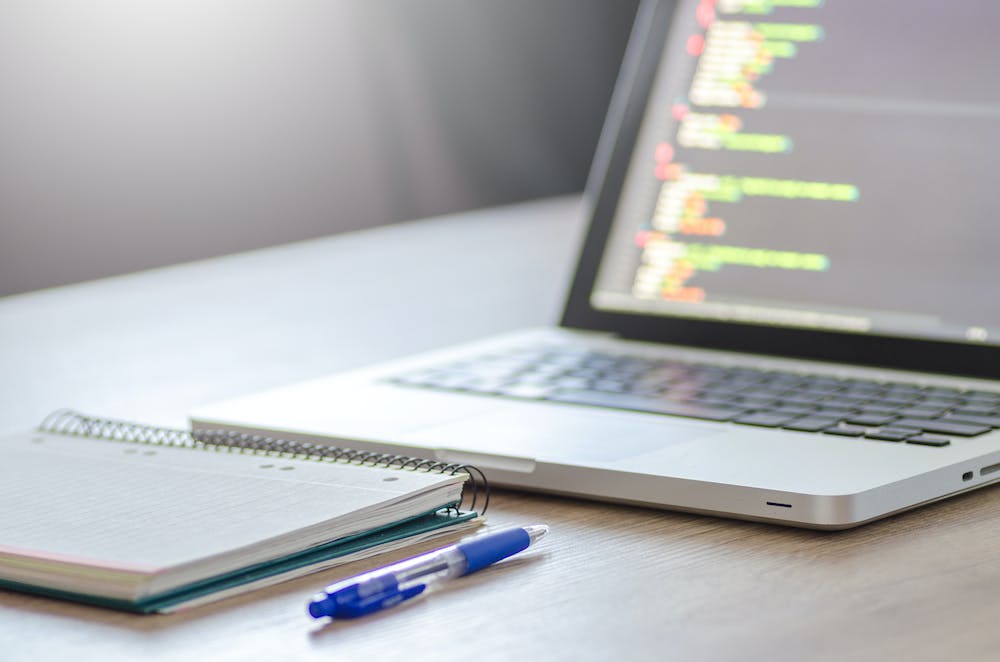
JavaScript is a crucial component of web development, but IT can also be a performance bottleneck if not optimized properly. One way to streamline JavaScript loading and execution is by using RequireJS. In this article, we will explore how RequireJS can improve JavaScript performance and how to implement it in your web projects.
What is RequireJS?
RequireJS is a JavaScript file and module loader that helps improve code organization, maintainability, and performance. It allows you to define and load dependencies for your JavaScript modules in a structured manner, ensuring that the right code is loaded at the right time without cluttering the global namespace.
Improving Performance with RequireJS
One of the key benefits of using RequireJS is its ability to optimize JavaScript performance. By modularizing your code and loading only the necessary dependencies when they are needed, RequireJS helps reduce the initial page load time and improve the overall responsiveness of your web application.
When you use RequireJS, it automatically handles the asynchronous loading of JavaScript files, which means that the browser can continue rendering the page while JavaScript files are being loaded in the background. This can significantly improve the perceived performance of your web application, especially on slower network connections.
Implementing RequireJS in Your Project
Implementing RequireJS in your project is straightforward. First, you need to include the RequireJS library in your HTML file using a script tag:
<script src="path/to/require.js"></script>
Next, you define your JavaScript modules using the define function provided by RequireJS. This function takes an array of dependencies and a callback function that defines the module:
define(['dependency1', 'dependency2'], function(dependency1, dependency2) {
// Module code goes here
});
Finally, you use the require function to load and execute your main application module:
require(['app'], function(app) {
// Your main application code goes here
});
By following these steps, you can modularize your JavaScript code and take advantage of RequireJS’s performance optimizations.
RequireJS and SEO
One concern with using RequireJS is its potential impact on search engine optimization (SEO). Since RequireJS loads JavaScript dynamically, it may not be immediately apparent to search engine crawlers what content is being loaded and when. However, this issue can be mitigated by using server-side rendering for initial page content and ensuring that critical JavaScript is loaded synchronously.
Conclusion
RequireJS is a powerful tool for optimizing JavaScript performance in web applications. By modularizing your code and managing dependencies more effectively, you can improve the loading and execution of JavaScript, leading to a faster and more responsive user experience. Consider using RequireJS in your next web project to take advantage of its performance benefits.
FAQs
Is RequireJS the only option for optimizing JavaScript performance?
No, there are other tools and techniques available for optimizing JavaScript performance, such as minification, bundling, and lazy loading. RequireJS is just one option that offers specific benefits for modularizing code and managing dependencies.
Can RequireJS be used with front-end frameworks like React or Angular?
Yes, RequireJS can be used with front-end frameworks to manage and load dependencies for individual components or modules. However, some frameworks may have their own built-in solutions for managing dependencies, so it’s important to check compatibility and best practices for each framework.
Does RequireJS have any performance drawbacks?
While RequireJS can improve performance by optimizing JavaScript loading and execution, it may introduce some overhead in terms of network requests and file size. However, these drawbacks can be mitigated by using proper configuration and optimization techniques.