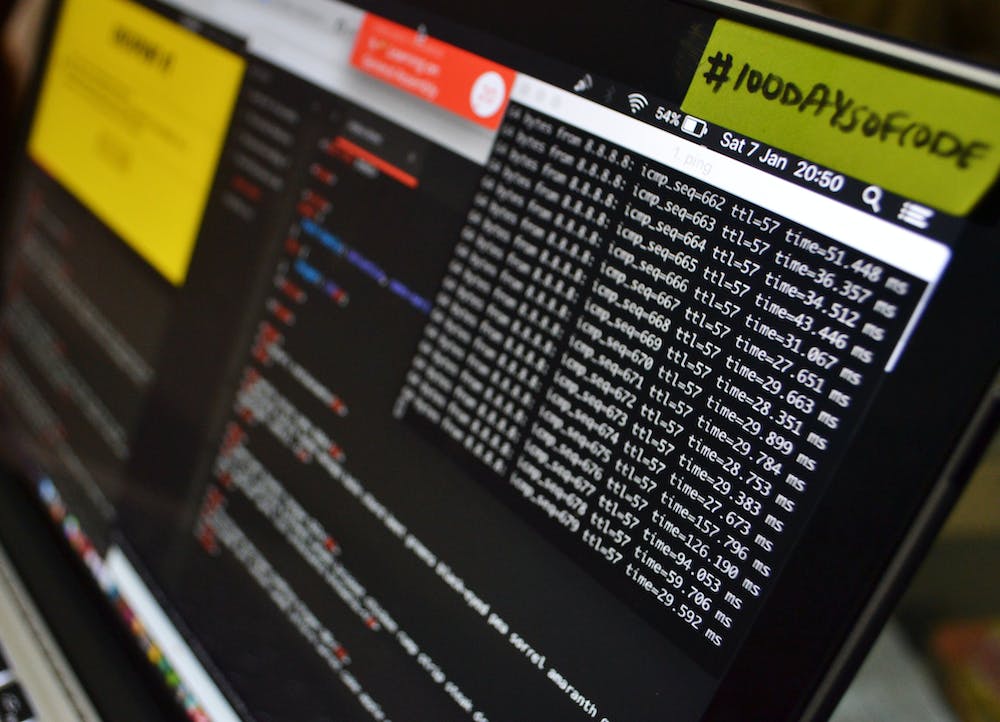
In this tutorial, we will learn how to use the Laravel DB Select method to fetch data from a database in a Laravel application. Laravel is a popular PHP framework that provides a variety of tools for building web applications. One of the most important components of a web application is its ability to interact with a database. Laravel makes this process easy with its elegant and expressive ORM (Object-Relational Mapping) called Eloquent.
Getting Started with Laravel
If you haven’t already installed Laravel, you can do so by following the official installation guide on the Laravel Website. Once you have Laravel installed, you can create a new project by running the following command in your terminal:
composer create-project --prefer-dist laravel/laravel my-project
Replace ‘my-project’ with the name of your project. After creating the project, navigate to its directory and start the development server by running the following command:
php artisan serve
You can now access your Laravel application by visiting http://localhost:8000 in your web browser.
Setting Up the Database
Before we can start using the DB Select method, we need to set up a database for our Laravel application. Open the .env
file in the root of your Laravel project and configure the database connection settings:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database
DB_USERNAME=your_username
DB_PASSWORD=your_password
Replace your_database
, your_username
, and your_password
with your actual database information. Once the database connection is configured, run the following command to create a migration for a new table in your database:
php artisan make:migration create_users_table --create=users
This will create a new migration file in the database/migrations
directory. Open the migration file and define the schema for the users
table:
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->remember_token();
$table->timestamps();
});
After defining the schema, run the migration to create the users
table in your database:
php artisan migrate
With the database set up, we can now start using the Laravel DB Select method to fetch data from the users
table.
Using Laravel DB Select
The DB facade in Laravel provides a variety of methods for interacting with the database. One of the most commonly used methods is Select, which allows you to retrieve data from a database table. Let’s take a look at how we can use the Select method to fetch all users from the users
table.
$users = DB::table('users')->select('id', 'name', 'email')->get();
In the above example, we are using the DB facade to select the id
, name
, and email
columns from the users
table. The get
method returns a collection of user records that match the select criteria.
Filtering Results with Where
In addition to selecting specific columns, you can also filter the results using the Where method. For example, let’s say we want to fetch only the users whose email address is verified:
$verifiedUsers = DB::table('users')->select('id', 'name', 'email')
->whereNotNull('email_verified_at')->get();
In this example, we are using the WhereNotNull method to filter the results based on the email_verified_at
column. This will return only the users whose email address has been verified.
Ordering Results with OrderBy
You can also order the results using the OrderBy method. For example, let’s say we want to fetch the users in alphabetical order by their names:
$orderedUsers = DB::table('users')->select('id', 'name', 'email')
->orderBy('name', 'asc')->get();
In this example, we are using the OrderBy method to order the results in ascending order based on the name
column.
Limited Results with Take
If you only want to fetch a limited number of results, you can use the Take method. For example, let’s say we want to fetch the first 10 users from the users
table:
$limitedUsers = DB::table('users')->select('id', 'name', 'email')->take(10)->get();
In this example, we are using the Take method to limit the results to the first 10 users from the users
table.
Conclusion
The Laravel DB Select method provides a powerful and flexible way to fetch data from a database in a Laravel application. By using the Select method along with other methods like Where, OrderBy, and Take, you can easily retrieve the data you need for your application. This tutorial has provided an introduction to using the Select method, and there are many more features and capabilities to explore in the Laravel documentation.
FAQs
What is the DB facade in Laravel?
The DB facade in Laravel provides a variety of methods for interacting with the database, including Select, Insert, Update, and Delete. IT offers an expressive and fluent syntax for building database queries in your Laravel application.
Can I use the Select method with Eloquent models?
Yes, you can use the Select method with Eloquent models by calling the Select method on the model class. For example, User::select('id', 'name', 'email')->get();
will retrieve only the specified columns from the users table using the User model.
Is the DB facade the only way to interact with the database in Laravel?
No, the DB facade is not the only way to interact with the database in Laravel. Laravel also provides an elegant ORM called Eloquent, which allows you to work with database records as objects. Eloquent provides a variety of methods for querying and manipulating data in your application.