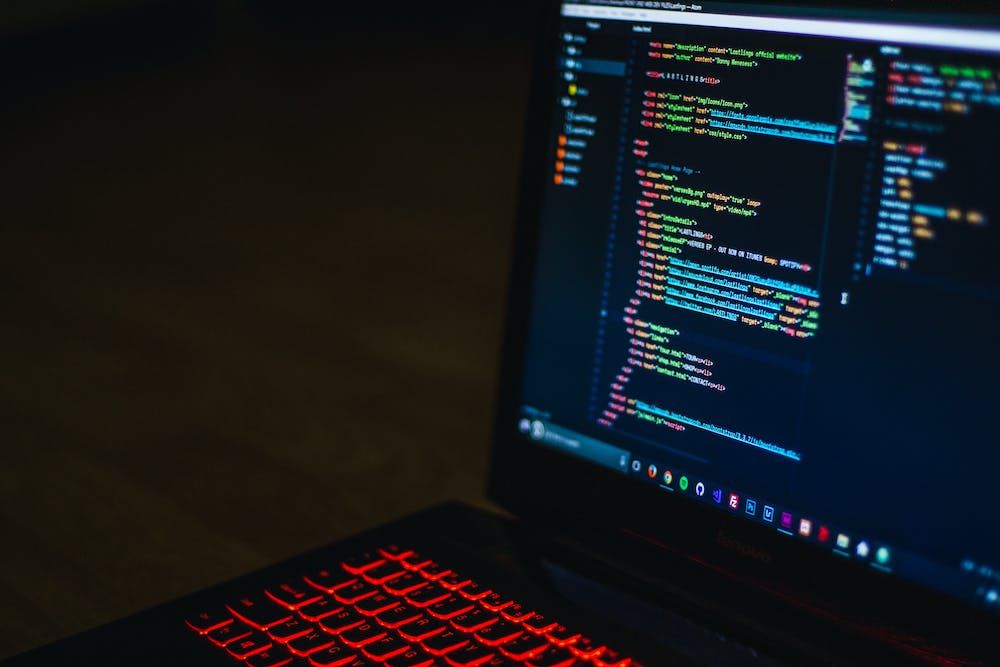
Using cURL in PHP: A Comprehensive Guide
cURL is a powerful tool that allows you to transfer data to and from servers using various protocols. In the context of PHP, cURL is a library that provides functionality to make HTTP requests, send and receive data, and handle responses. This comprehensive guide will walk you through the process of using cURL in PHP, from installation to advanced usage and troubleshooting. So let’s dive in!
Installation and Setup
Before you can start using cURL in PHP, you need to ensure that IT is installed and enabled on your system. Most modern PHP installations come with cURL preinstalled, but you can verify its presence by running the following code:
<?php
if (in_array('curl', get_loaded_extensions())) {
echo 'cURL is installed and enabled';
} else {
echo 'cURL is not installed or enabled';
}
?>
If cURL is not installed, you can install IT by referring to the official PHP documentation or checking your platform-specific instructions. Once you have IT installed, you can enable the cURL extension by modifying your php.ini file, typically located in your PHP installation directory. Search for the line that starts with “extension=…” and add the following line below IT:
extension=curl
Basic Usage
With cURL installed and enabled, you are now ready to start using IT in your PHP projects. The most common use case is making HTTP GET requests to retrieve data from a server. Here’s a basic example:
<?php
// Create a new cURL resource
$curl = curl_init();
// Set the URL and other options
curl_setopt($curl, CURLOPT_URL, 'https://example.com/api/data');
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
// Send the request and get the response
$response = curl_exec($curl);
// Close cURL resource
curl_close($curl);
// Process the response
if ($response !== false) {
// Handle the response
echo $response;
} else {
// Handle the error
echo 'An error occurred: ' . curl_error($curl);
}
?>
In this example, we first initialize a new cURL resource using the curl_init() function. We then set the URL we want to fetch with curl_setopt(), along with other options such as CURLOPT_RETURNTRANSFER which instructs cURL to return the response instead of outputting IT directly.
After sending the request using curl_exec(), we retrieve the response, process IT, and close the cURL resource using curl_close(). IT is important to always close the resource after you are done to free up system resources.
Advanced Usage
While the basic usage covers most common scenarios, cURL provides a wide range of additional options and functionalities for more advanced use cases. Let’s explore some of them:
- Setting request headers: You can customize the HTTP headers of your request using the CURLOPT_HTTPHEADER option.
- Handling POST requests: You can send POST data with cURL by using CURLOPT_POST and CURLOPT_POSTFIELDS options.
- Authentication: If your API requires authentication, you can set the username and password using the CURLOPT_USERPWD option.
- Working with cookies: cURL allows you to handle cookies and maintain session state with the CURLOPT_COOKIE and CURLOPT_COOKIEFILE options.
- Handling SSL/TLS: If you need to make requests to servers with self-signed certificates or specific SSL/TLS configurations, you can specify additional options such as CURLOPT_SSL_VERIFYPEER and CURLOPT_SSL_VERIFYHOST.
These are just a few examples of the advanced capabilities of cURL in PHP. The official PHP documentation and the cURL documentation provide in-depth information on all available options and functionalities.
FAQs (Frequently Asked Questions)
Q: Can I make asynchronous requests with cURL in PHP?
A: No, by default, cURL operates in a synchronous manner. However, you can make multiple requests in parallel using techniques such as multi-handle requests or asynchronous libraries like Guzzle.
Q: Is cURL the only option for making HTTP requests in PHP?
A: No, cURL is not the only option. PHP also provides built-in functions like file_get_contents() and stream_context_create() that can be used to make HTTP requests. However, cURL offers more flexibility and advanced features compared to these alternatives.
Q: How can I debug cURL requests and handle errors?
A: You can enable verbose mode by setting the CURLOPT_VERBOSE option to true. This will output detailed information about the request and response, including any errors that occurred.
Q: Can cURL handle file uploads?
A: Yes, cURL can handle file uploads by using the CURLOPT_POSTFIELDS option along with the ‘@’ symbol followed by the file path.
Q: Is IT necessary to close the cURL resource after each request?
A: Yes, IT is good practice to close the cURL resource using curl_close() after you are done with each request. Failing to do so may result in resource leaks and potential performance issues.