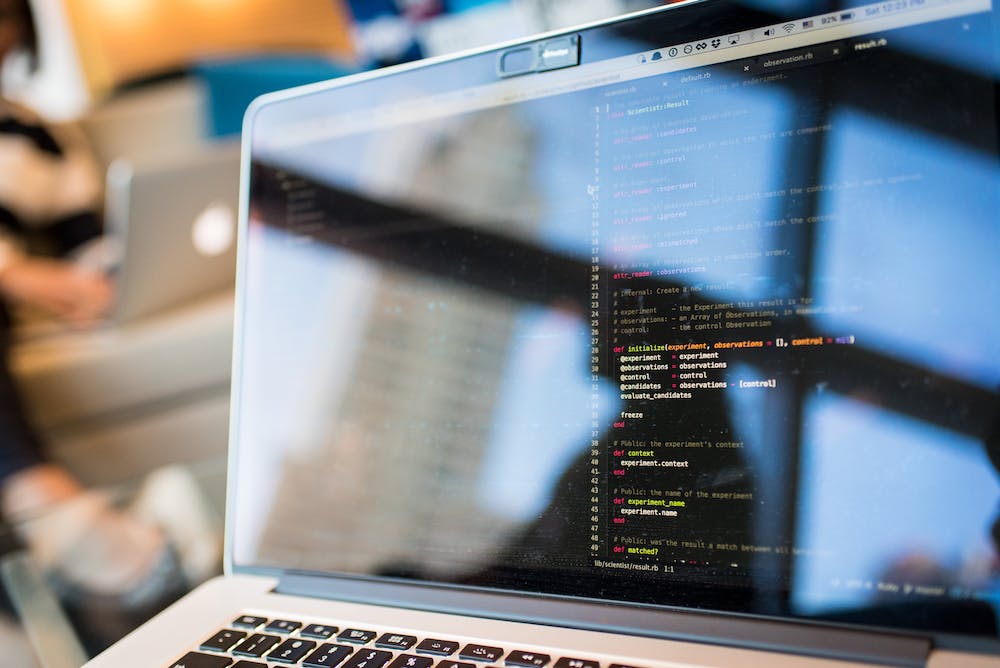
File uploading is a common feature in web development, and PHP provides a simple and efficient way to handle file uploads. Whether you want to create a file upload form for user submissions or store files on a server, PHP makes the process straightforward. In this step-by-step guide, we will explore the process of uploading files with PHP and walk you through the necessary steps to implement IT in your web application.
Step 1: Create the HTML Form
The first step in the file upload process is to create an HTML form that allows users to select a file for upload. The form should have an input field of type “file” that enables users to browse their local file system and select the file they want to upload. Here’s an example of an HTML form for file upload:
“`html
“`
In this example, the form’s action attribute specifies the PHP script that will process the file upload, while the enctype attribute ensures that the form data is encoded as “multipart/form-data” to support file uploads.
Step 2: Process the File Upload in PHP
Once the user selects a file and submits the form, the file upload needs to be processed in a PHP script. The script will receive the uploaded file and handle it according to the application’s requirements. Here’s a basic example of a PHP script to handle file uploads:
“`php
$targetDirectory = “uploads/”;
$targetFile = $targetDirectory . basename($_FILES[“file”][“name”]);
if (move_uploaded_file($_FILES[“file”][“tmp_name”], $targetFile)) {
echo “The file “. htmlspecialchars( basename( $_FILES[“file”][“name”])). ” has been uploaded.”;
} else {
echo “Sorry, there was an error uploading your file.”;
}
?>
“`
In this PHP script, we specify a target directory for the uploaded files and use the move_uploaded_file() function to move the uploaded file to the target directory. If the file upload is successful, a success message is displayed; otherwise, an error message is shown to the user.
Step 3: Handle File Upload Errors
When working with file uploads, it’s essential to handle potential errors that may occur during the upload process. PHP provides various error codes to indicate different types of upload errors, such as file size exceeded, file type not allowed, or server error. Here’s an example of how to handle file upload errors in PHP:
“`php
if ($_FILES[“file”][“error”] != 0) {
switch ($_FILES[“file”][“error”]) {
case 1:
echo “The uploaded file exceeds the upload_max_filesize directive in php.ini.”;
break;
case 2:
echo “The uploaded file exceeds the MAX_FILE_SIZE directive that was specified in the HTML form.”;
break;
case 3:
echo “The uploaded file was only partially uploaded.”;
break;
case 4:
echo “No file was uploaded.”;
break;
case 6:
echo “Missing a temporary folder.”;
break;
case 7:
echo “Failed to write file to disk.”;
break;
case 8:
echo “A PHP extension stopped the file upload.”;
break;
default:
echo “Sorry, there was an error uploading your file.”;
}
}
?>
“`
In this example, we use the $_FILES[“file”][“error”] variable to check for different error codes and display corresponding error messages to the user.
Step 4: Limit File Type and Size
It’s essential to limit the types of files that users can upload to prevent malicious uploads and ensure security. You can use PHP to validate the file type and size before allowing the upload to proceed. Here’s an example of how to limit file type and size in PHP:
“`php
$allowedFileTypes = array(“jpg”, “jpeg”, “png”);
$maxFileSize = 5242880; // 5MB
$fileExtension = pathinfo($_FILES[“file”][“name”], PATHINFO_EXTENSION);
if (!in_array($fileExtension, $allowedFileTypes)) {
echo “Sorry, only JPG, JPEG, and PNG files are allowed.”;
} elseif ($_FILES[“file”][“size”] > $maxFileSize) {
echo “Sorry, your file is too large. Please upload a file of up to 5MB.”;
} else {
// Process the file upload
}
?>
“`
In this example, we define an array of allowed file types and a maximum file size limit. We then use the pathinfo() function to retrieve the file extension and check if it’s allowed. We also compare the file size with the maximum allowable size and display appropriate messages to the user.
Step 5: Secure File Uploads
File uploads can pose security risks, and it’s crucial to implement measures to secure the file upload process. Here are some best practices to secure file uploads in PHP:
- Use a secure target directory to store uploaded files.
- Ensure that uploaded files cannot be executed as scripts on the server.
- Implement file name sanitization to prevent directory traversal attacks.
- Restrict file permissions to prevent unauthorized access.
- Use server-side validation in addition to client-side validation.
By following these best practices, you can enhance the security of file uploads in your PHP application and protect against potential vulnerabilities.
Step 6: Displaying Uploaded Files
Once the file upload is successful, you may want to display the uploaded file to the user or store its details in a database. Here’s an example of how to display an uploaded image using PHP:
“`php
$uploadedFile = “uploads/” . $_FILES[“file”][“name”];
echo ““;
?>
“`
In this example, we use the img tag to display the uploaded image to the user. You can also store the file details, such as file name, location, and upload timestamp, in a database for future reference.
Conclusion
Uploading files with PHP is a common requirement in web development, and PHP provides a robust set of functions to facilitate the file upload process. By following this step-by-step guide, you can effectively handle file uploads in your PHP application, implement security measures, and provide a seamless user experience for file uploads.
FAQs
Q: Can I upload multiple files at once using PHP?
A: Yes, you can create an HTML form with multiple file input fields to allow users to upload multiple files simultaneously. In the PHP script, you can handle multiple file uploads using a loop to process each uploaded file individually.
Q: Is it possible to resize and manipulate uploaded images with PHP?
A: Yes, PHP provides image manipulation functions, such as imagecreatefromjpeg(), imagecreatefrompng(), and imagecopyresized(), to resize, crop, and manipulate uploaded images. You can use these functions to perform various image processing tasks based on your requirements.
Q: How can I improve the performance of file uploads in PHP?
A: To enhance the performance of file uploads, you can optimize server settings, such as increasing the upload_max_filesize and post_max_size directives in php.ini, implementing file compression techniques, and utilizing caching mechanisms to reduce server load and improve upload speed.
By following these tips and best practices, you can ensure that file uploads in your PHP application are efficient and secure, providing a seamless experience for users.