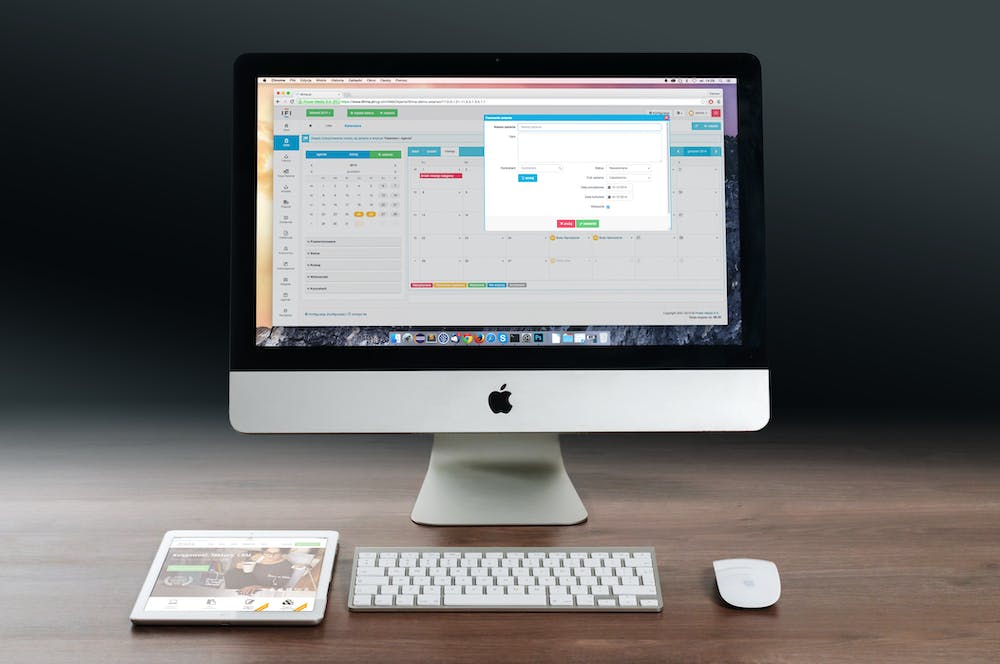
Introduction
Ajax, short for Asynchronous JavaScript and XML, is a powerful tool that allows web developers to create dynamic and interactive websites. When combined with Django, a popular web framework for Python, Ajax brings a whole new level of functionality to your Website. In this article, we will explore how to master Ajax in Django and build dynamic websites like a pro.
What is Ajax?
Ajax is a technology that allows web pages to be updated asynchronously by exchanging data with a web server in the background. This means that instead of reloading the entire page whenever a user interacts with IT, only certain parts of the page can be updated. This significantly enhances user experience by providing seamless and responsive interactions.
Using Ajax in Django
Django provides a convenient way to work with Ajax through its built-in features and third-party libraries. To get started, you need to include the necessary JavaScript libraries in your HTML templates:
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/sweetalert2.min.js"></script>
These libraries will allow us to make Ajax requests and display messages to the user.
Ajax Requests in Django
To make an Ajax request in Django, we can use the jQuery library as follows:
$(document).ready(function() {
// Code goes here
});
Within this function, we can define event handlers for different interactions. For example, to handle a form submission via Ajax, we can use the following code:
$('#myForm').on('submit', function(event) {
event.preventDefault();
$.ajax({
url: '/my-url/',
type: 'POST',
data: $('#myForm').serialize(),
success: function(response) {
// Code to handle the response
}
});
});
This code intercepts the form submission event, prevents IT from executing the default action, and sends an Ajax request to the server with the form data. The `url` argument specifies the URL to which the request is sent, the `type` argument indicates the HTTP method (in this case, POST), and the `data` argument contains the serialized form data.
Handling Ajax Requests in Django
In Django, we can handle Ajax requests by defining corresponding views. For example, to handle the previous form submission, we can create the following view:
from django.http import JsonResponse
def my_view(request):
if request.method == 'POST' and request.is_ajax():
# Process the form data
return JsonResponse({'success': True})
else:
return JsonResponse({'success': False})
This view checks if the request method is POST and if IT is an Ajax request. IT then processes the form data and returns a JSON response indicating whether the operation was successful or not.
Conclusion
Ajax is a powerful tool that allows web developers to create dynamic and interactive websites. By mastering Ajax in Django, you can enhance the user experience and build websites with seamless and responsive interactions. With the techniques covered in this article, you are now equipped to start building dynamic websites with Ajax in Django. Happy coding!
FAQs
1. Can Ajax be used with Django?
Yes, Django provides built-in features and third-party libraries that allow you to work with Ajax seamlessly.
2. Is Ajax necessary for building dynamic websites?
No, Ajax is not necessary for building dynamic websites, but IT greatly enhances the user experience by providing seamless and responsive interactions.
3. Are there any alternative libraries to jQuery for making Ajax requests in Django?
Yes, besides jQuery, you can use libraries like Axios or Fetch API to make Ajax requests in Django.
4. Can Ajax requests be used for authentication in Django?
Yes, Ajax requests can be used for handling user authentication in Django. You can send login or registration data via Ajax and process IT on the server side.
5. Are there any security considerations when using Ajax in Django?
Yes, when working with Ajax in Django, IT is important to protect against common web security vulnerabilities, such as Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF). Django provides built-in mechanisms to handle these security concerns, such as CSRF tokens.