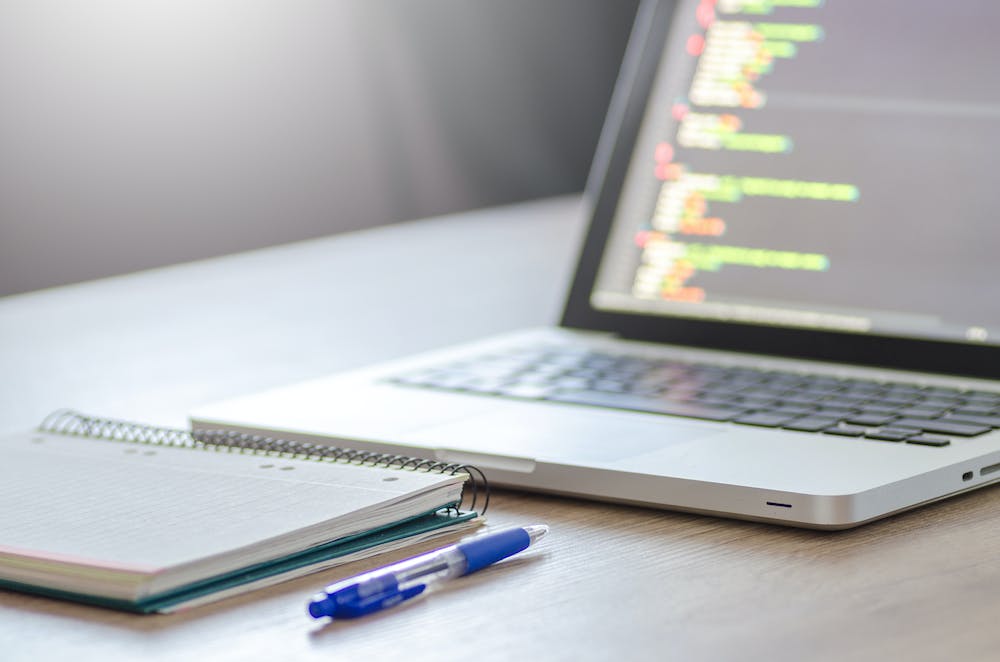
Python is a popular and versatile programming language that has gained immense popularity in recent years. Known for its simplicity and readability, Python is an excellent choice for beginners who want to learn programming. Whether you want to build your career as a software developer, data scientist, or simply enhance your problem-solving skills, Python is a great language to start with.
In this article, we will unveil the top 10 basic Python programs that are perfect for beginners who are starting their coding journey. These programs cover a range of fundamental concepts and can help you understand the basic syntax and logic used in Python. So, let’s dive in and explore the exciting world of Python programming!
1. Hello, World!
The classic “Hello, World!” program is the most basic program you can write in any programming language. IT simply prints the phrase “Hello, World!” on the output screen. This program is often the first step for beginners in understanding how to write and run a program in Python.
print("Hello, World!")
Running this program will display “Hello, World!” on the output screen. IT may seem trivial, but this program lays the foundation for more complex coding tasks.
2. Simple Calculator
A simple calculator program can perform basic arithmetic operations such as addition, subtraction, multiplication, and division. IT takes input from the user and produces the result based on the chosen operation. This program helps beginners understand how to work with user input and perform elementary calculations.
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
sum = num1 + num2
difference = num1 - num2
product = num1 * num2
quotient = num1 / num2
print("Sum:", sum)
print("Difference:", difference)
print("Product:", product)
print("Quotient:", quotient)
The above program prompts the user to enter two numbers and calculates the sum, difference, product, and quotient of those numbers. The results are then printed on the output screen.
3. Check if a Number is Even or Odd
A program that determines if a given number is even or odd is another basic exercise that helps beginners learn about conditional statements and the modulo operator. IT is a good way to understand how to check for divisibility and perform different actions based on the result.
num = int(input("Enter a number: "))
if num % 2 == 0:
print(num, "is even")
else:
print(num, "is odd")
This program takes a number as input and checks whether IT is even or odd. IT uses the modulo operator (%), which returns the remainder of division, to determine if the number is divisible by 2.
4. Fibonacci Series
The Fibonacci series is a famous sequence of numbers in which each number is the sum of the two preceding ones. A program to generate the Fibonacci series is an interesting exercise that helps beginners understand loops and recursion.
n1, n2 = 0, 1
count = 0
if n <= 0:
print("Please enter a positive integer")
elif n == 1:
print("Fibonacci sequence up to", n, ":")
print(n1)
else:
print("Fibonacci sequence:")
while count < n:
print(n1)
nth = n1 + n2
n1 = n2
n2 = nth
count += 1
In this program, the user enters the number of terms they want to generate in the Fibonacci series. The program then uses a while loop to calculate and print the series.
5. Check if a Number is Prime
A program to check whether a given number is a prime number is an essential exercise for beginners to learn about loops and break statements. IT helps in understanding how to determine if a number has only two factors: 1 and itself.
num = int(input("Enter a number: "))
if num > 1:
for i in range(2, num):
if (num % i) == 0:
print(num, "is not a prime number")
break
else:
print(num, "is a prime number")
else:
print(num, "is not a prime number")
This program checks whether the input number is greater than 1 and then iterates through a range of numbers from 2 to the input number. If the input number is divisible by any number in this range, IT is not prime. If no factors are found, the number is prime.
6. Reverse a String
A program that reverses a given string is a fun exercise that helps beginners understand string manipulation. IT involves iterating through the string in reverse order and appending each character to a new string to produce the reversed version.
string = input("Enter a string: ")
reversed_string = ''
for char in string:
reversed_string = char + reversed_string
print("Reversed string:", reversed_string)
This program prompts the user to enter a string and then reverses IT character by character using a for loop.
7. Count the Number of Words in a Sentence
A program to count the number of words in a given sentence or string is an excellent exercise for beginners to understand loops, conditional statements, and string manipulation. IT involves iterating through each character in the sentence and counting the spaces to determine the number of words.
sentence = input("Enter a sentence: ")
word_count = 1
for char in sentence:
if char == ' ':
word_count += 1
print("Number of words in the sentence:", word_count)
This program takes a sentence as input and counts the number of words by incrementing a counter every time IT encounters a space character.
8. Generate a Multiplication Table
A program to generate a multiplication table for a given number helps beginners understand nested loops. IT is a useful exercise that involves iterating through rows and columns to multiply corresponding values.
num = int(input("Enter a number: "))
for i in range(1, 11):
print(num, 'x', i, '=', num * i)
This program takes a number as input and generates the multiplication table for that number from 1 to 10. IT uses a nested loop to generate each row.
9. Find the Largest Number in a List
A program to find the largest number in a given list is an essential exercise for beginners to understand loops and conditional statements. IT involves iterating through each element in the list and comparing IT with the current largest number.
numbers = [10, 25, 8, 67, 45, 2]
largest = numbers[0]
for num in numbers:
if num > largest:
largest = num
print("The largest number in the list is:", largest)
This program initializes the largest variable with the first element in the list and then iterates through the remaining elements to find the largest number.
10. Check if a String is a Palindrome
A program that checks whether a given string is a palindrome helps beginners understand loops and string manipulation. IT involves comparing the characters from both ends of the string to determine if they form the same string when reversed.
if string == string[::-1]:
print("The string is a palindrome")
else:
print("The string is not a palindrome")
This program checks whether the input string is equal to its reverse. If they match, the string is a palindrome.
Conclusion
These top 10 basic Python programs for beginners are a great way to start your coding journey. They cover essential concepts such as input/output, conditional statements, loops, and string manipulation. By implementing these programs, you will gain a solid foundation in Python programming that will enable you to tackle more complex coding tasks.
Remember, practice is vital for mastering any programming language. Try modifying these programs, experiment with different inputs, and explore additional functionalities to enhance your skills. As you progress, you will be ready to take on more advanced Python programming challenges.
FAQs
Q: Is Python a suitable language for beginners?
A: Yes! Python is known for its simplicity and readability, making IT an excellent choice for beginners who are starting their coding journey.
Q: What are the benefits of learning Python?
A: Learning Python opens up various career opportunities in software development, data analysis, artificial intelligence, web development, and more. IT is widely used in industry and academia due to its ease of use and extensive libraries.
Q: Are these programs suitable for absolute beginners?
A: Yes, these programs are designed specifically for beginners. They cover fundamental concepts and gradually introduce more advanced topics.
Q: Can I use an Integrated Development Environment (IDE) for writing and running Python programs?
A: Yes, you can choose from various popular IDEs, such as PyCharm, Visual Studio Code, or Jupyter Notebook, to write and run your Python programs. These IDEs provide helpful features like syntax highlighting, code suggestions, debugging tools, and more.
Q: Where can I find more resources to learn Python?
A: There are plenty of online resources available for learning Python, including tutorials, documentation, video courses, and interactive coding platforms like Codecademy, Coursera, and Udemy. Utilize these resources in conjunction with practice to enhance your Python skills.