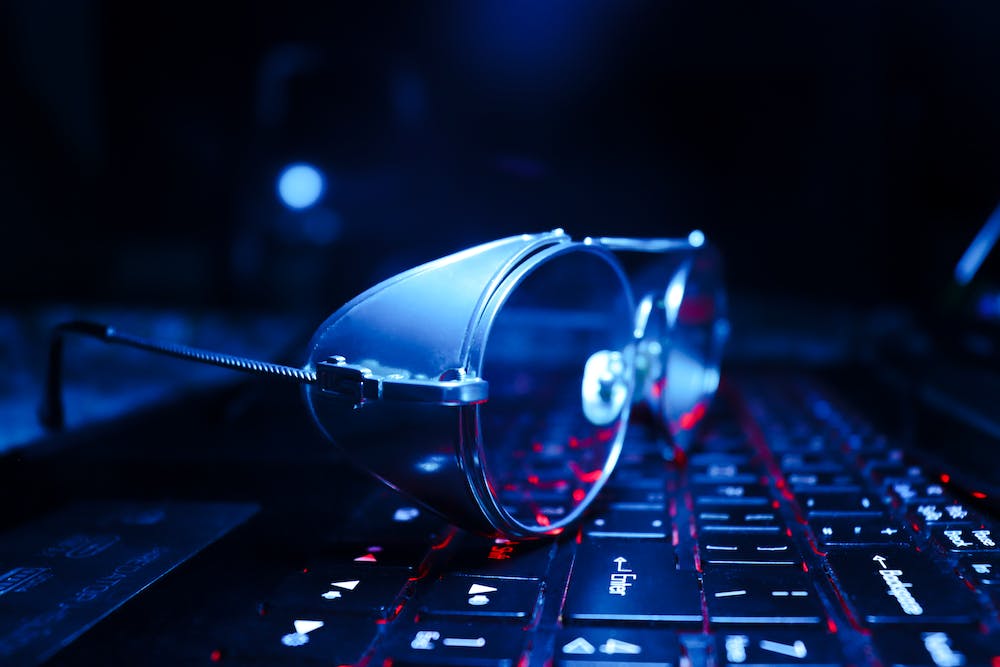
PHP, which stands for Hypertext Preprocessor, is a popular scripting language widely used for web development. Known for its simplicity and versatility, PHP has become the go-to choice for developers all around the world. In this article, we will delve into the hidden secrets of PHP that will broaden your understanding and take your coding skills to the next level.
Let’s begin by exploring some lesser-known features of PHP that can enhance your development process:
1. Namespaces
Namespaces allow you to organize your code into logical containers, preventing naming conflicts and improving code maintainability. By encapsulating your functions, classes, and variables within a namespace, you can ensure that your code remains modular and easy to understand. Consider the following example:
namespace MyProject;
class MyClass {
// Class methods and properties here
}
In the above code snippet, the MyClass
class is encapsulated within the MyProject
namespace. This allows other developers to use the class without worrying about naming conflicts with their own codebase.
2. Traits
Traits are a powerful feature in PHP that enable you to reuse code across multiple classes. They provide a mechanism for horizontal code sharing, allowing you to define methods that can be used by different classes without the need for inheritance. Let’s consider an example:
trait Logger {
public function log($message) {
// Log the message
}
}
class MyClass {
use Logger;
// Class methods and properties here
}
In the above code, the Logger
trait is defined separately and then used within the MyClass
class using the use
keyword. This way, the log
method defined in the trait becomes available in the MyClass
class.
3. Generators
Generators provide an efficient way to iterate over large sets of data without loading them all into memory. They allow you to create functions that can produce sequences of values, making them ideal for working with large datasets or when memory usage is a concern. Here’s an example:
function generateNumbers($start, $end) {
for ($i = $start; $i <= $end; $i++) {
yield $i;
}
}
foreach (generateNumbers(1, 1000000) as $number) {
// Process each number
}
In the above code, the generateNumbers
function is a generator that produces numbers from a specified range. By iterating over the generator using a foreach
loop, you can process each number one at a time, without having to load the entire range into memory.
Now that we have uncovered some hidden gems of PHP, IT‘s time to wrap things up.
Conclusion
PHP’s popularity stems from its flexibility, ease of use, and extensive community support. By utilizing advanced features like namespaces, traits, and generators, you can write more modular, reusable, and efficient code. Remember to explore the PHP documentation and experiment with these concepts in your own projects to fully grasp their potential.
FAQs
1. Is PHP suitable for large-scale projects?
Yes, PHP is capable of handling large-scale projects given its scalability and robust ecosystem of frameworks and libraries. Many popular websites, such as Facebook and Wikipedia, rely on PHP for their backend.
2. Can I use PHP for mobile app development?
While PHP is primarily used for web development, frameworks like Laravel and Phalcon allow you to build APIs for mobile app development. You can use PHP in combination with technologies like React Native or Flutter to create mobile applications.
3. Are there any disadvantages of using PHP?
Some developers argue that PHP’s syntax can be inconsistent at times, leading to potential bugs. Moreover, PHP’s shared-nothing architecture, where each request starts from scratch, can make IT less suitable for high-performance applications compared to languages like Node.js or Golang.
4. Is PHP suitable for beginners?
PHP is an ideal language for beginners due to its simple and readable syntax. Its extensive documentation and vibrant community make IT easy to find support and resources when starting out.
5. Can PHP be used for server-side scripting?
Yes, PHP was originally designed for server-side scripting, allowing you to generate dynamic web pages and interact with databases. IT excels at handling form submissions, processing data, and interacting with external APIs.
Now armed with these insights and answers to common questions, you are ready to explore the depth of PHP and unlock its true potential!