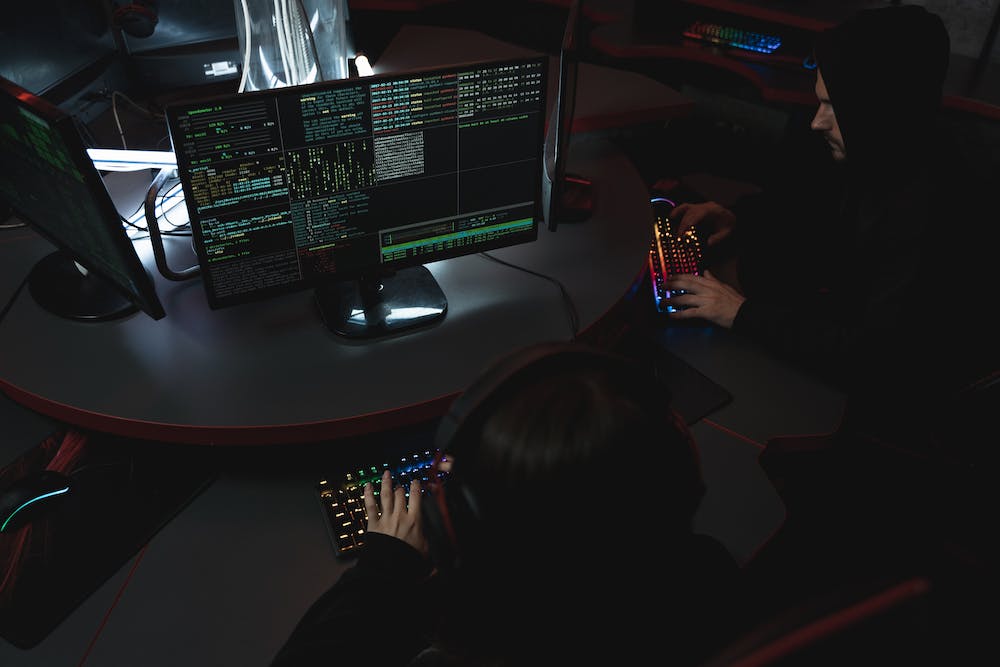
Welcome to our article on JavaScript SHA256 encryption, where we will explore the secrets of this powerful encryption algorithm and learn how to use IT effectively to secure your data. In this article, we will provide you with a comprehensive understanding of SHA256, its implementation in JavaScript, and demonstrate how you can encrypt data using this technique like a pro.
The Basics of SHA256 Encryption:
SHA256, which stands for Secure Hash Algorithm 256-bit, is a cryptographic algorithm used primarily for data integrity and security purposes. IT operates on a binary input and produces a fixed-size 256-bit hash value representing that input. This hash value is unique to the provided input and is practically impossible to reverse engineer or retrieve the original data from IT.
One of the key advantages of SHA256 over other encryption algorithms is its resistance to cryptographic vulnerabilities, such as collision attacks. This ensures that even a small change in the input results in a drastically different hash value. The SHA256 algorithm has become widely adopted and is commonly used for various purposes, including password storage, digital signatures, and blockchain technology.
Implementing SHA256 in JavaScript:
To use the SHA256 algorithm in JavaScript, we have several libraries and APIs available that simplify the implementation process. One popular library is CryptoJS, which provides a bunch of cryptographic functions, including SHA256 encryption.
First, you need to include the CryptoJS library in your HTML file. You can download the library from the official CryptoJS Website or include IT using a content delivery network (CDN). Here is an example of how to import the CryptoJS library into your HTML file:
<script src="https://cdnjs.cloudflare.com/ajax/libs/crypto-js/4.1.1/crypto-js.min.js"></script>
Once you have included the library, you can start using the SHA256 function provided by CryptoJS to encrypt your data. Here is an example:
<script>
var hash = CryptoJS.SHA256("Hello, World!");
console.log(hash.toString());
</script>
In this example, we use the CryptoJS.SHA256() function to calculate the hash value of the provided string “Hello, World!”. The resulting hash, represented as an object, is converted to its string representation using the toString() method and logged to the console.
Encrypt Data like a Pro!
Now that we have covered the basics of SHA256 encryption and its implementation in JavaScript, let’s dive into how you can encrypt your data like a pro using this powerful technique.
1. Secure Password Storage: One of the most common use cases for SHA256 encryption is secure password storage. By applying the SHA256 algorithm to a user’s password, you can store only the resulting hash value in your database instead of the actual password. This ensures that even if your database is compromised, the original passwords cannot be retrieved.
Here’s an example in JavaScript:
var password = "mySecretPassword";
var hash = CryptoJS.SHA256(password);
var encryptedPassword = hash.toString();
// Store the encrypted password in your database
2. Digital Signatures: Digital signatures play a crucial role in verifying the authenticity of electronic documents or messages. By using SHA256 encryption in combination with asymmetric key algorithms, you can create a digital signature that ensures the integrity and non-repudiation of the data.
Here’s an example in JavaScript:
var data = "Hello, Bob!";
var privateKey = "yourPrivateKey";
var hash = CryptoJS.SHA256(data);
var signature = encryptWithPrivateKey(hash, privateKey);
// Send the data and the signature to Bob
function encryptWithPrivateKey(hash, privateKey) {
// Implementation of asymmetric encryption using private key
}
3. Blockchain technology: SHA256 is the hash function at the core of many blockchain technologies, including Bitcoin. IT is used to secure and validate the integrity of blocks within the chain. By encrypting each block’s data with SHA256, the blockchain network ensures transparency and immutability, making IT practically impossible to alter the stored data.
These are just a few examples of how SHA256 encryption can be used in JavaScript to enhance data security. The possibilities and applications are vast, and IT is crucial to implement the encryption techniques correctly to ensure maximum protection.
Conclusion:
In conclusion, JavaScript SHA256 encryption is a powerful tool for securing data and ensuring its integrity. By implementing SHA256 encryption techniques correctly, we can protect sensitive information, verify the authenticity of data, and ensure the security of blockchain technologies.
Throughout this article, we have explored the basics of SHA256 encryption, provided an example of its implementation in JavaScript using the popular CryptoJS library, and demonstrated how to encrypt data like a pro using SHA256. IT is essential to stay updated with the latest cryptographic advancements and best practices to adapt to evolving security challenges effectively.
FAQs:
1. Is JavaScript SHA256 encryption secure?
Yes, JavaScript SHA256 encryption is secure as long as implemented correctly. The SHA256 algorithm itself is highly secure and widely adopted in various applications, ensuring data integrity and resistance to cryptographic vulnerabilities.
2. Are there any alternatives to CryptoJS for SHA256 encryption in JavaScript?
Yes, besides CryptoJS, there are other libraries available for SHA256 encryption in JavaScript, such as Forge, sjcl (Stanford JavaScript Crypto Library), and more. IT is crucial to choose a library that is actively maintained and has a good reputation in the cryptographic community.
3. Can SHA256 encrypted data be decrypted?
No, SHA256 encryption is a one-way function, meaning IT is practically impossible to retrieve the original data from its hash value. Its primary purpose is to provide data integrity and secure password storage rather than encryption and decryption of data.
4. Are there any limitations or downsides to using SHA256 encryption?
While SHA256 encryption is highly secure, IT is important to note that IT generates fixed-size hash values. Therefore, there is a possibility of collision attacks, where different inputs produce the same hash value. However, the probability of such collisions is negligible, and SHA256 remains a robust encryption algorithm for most use cases.
References:
[1] SHA-2, Wikipedia. Available at: https://en.wikipedia.org/wiki/SHA-2
[2] CryptoJS, GitHub. Available at: https://github.com/brix/crypto-js
[3] SHA-256, MDN Web Docs. Available at: https://developer.mozilla.org/en-US/docs/Web/API/SubtleCrypto/digest#SHA-256