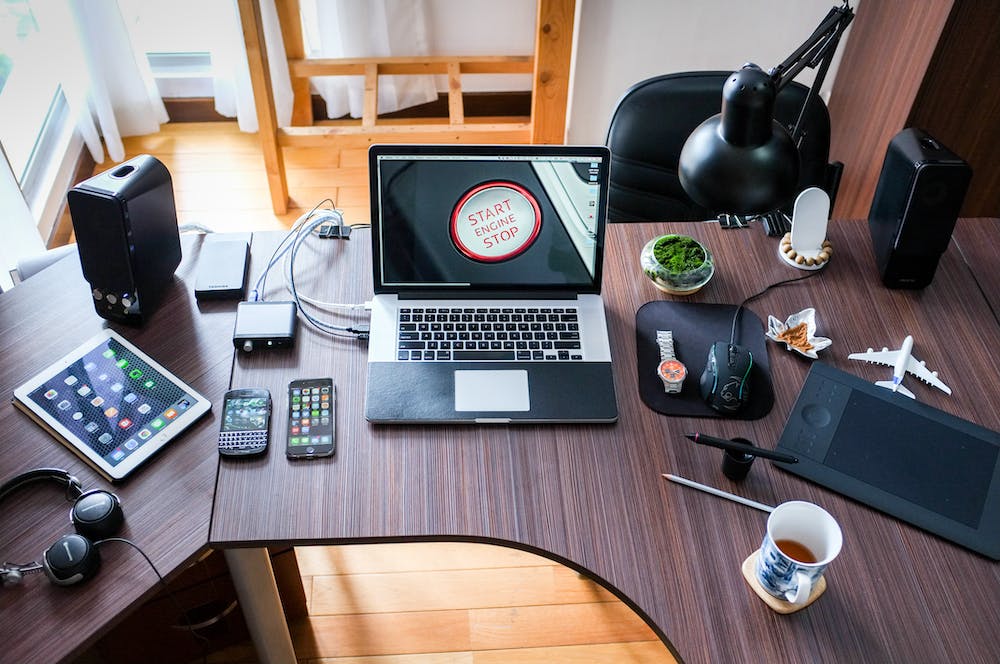
Introduction
PHP is a widely used programming language that allows developers to build dynamic and interactive websites. One of the essential features of PHP is the ability to check if a variable is set using the ‘if isset’ statement.
The ‘if isset’ Statement
The ‘if isset’ statement in PHP is used to check whether a variable is set or not. IT is commonly used in conditional statements to control the flow of the program based on the availability of a variable. The syntax for the ‘if isset’ statement is as follows:
if(isset($variable)){
// Code to be executed if the variable is set
} else {
// Code to be executed if the variable is not set
}
In the above syntax, the ‘$variable’ represents the variable that you want to check if IT is set or not. If the variable is set, the code inside the ‘if’ block will be executed, otherwise, the code inside the ‘else’ block will be executed.
Benefits of Using the ‘if isset’ Statement
The ‘if isset’ statement offers several benefits when used in PHP programming:
- Prevent undefined variable errors: Using the ‘if isset’ statement helps prevent undefined variable errors. IT ensures that your code will not throw an error if a variable is not set.
- Better control over program flow: By checking if a variable is set or not, you can make decisions based on the availability of a particular variable, allowing for better control over the flow of your program.
- Enhance code readability: The ‘if isset’ statement makes your code more readable and self-explanatory. IT clearly indicates that you are checking for the existence of a variable before performing any operations on IT.
Examples
Let’s take a look at a couple of examples to understand the usage of the ‘if isset’ statement:
// Example 1: Checking if a variable is set
$name = "John Doe";
if(isset($name)){
echo "The name is set: " . $name;
} else {
echo "The name is not set";
}
// Output: The name is set: John Doe
// Example 2: Checking if a variable is not set
$age = null;
if(isset($age)){
echo "The age is set: " . $age;
} else {
echo "The age is not set";
}
// Output: The age is not set
In the first example, the variable ‘$name’ is set to “John Doe,” so the ‘if’ block is executed, and the output displays the name. However, in the second example, the variable ‘$age’ is set to null, which means IT is not explicitly defined. This results in the ‘else’ block being executed, and the output indicates that the age is not set.
Conclusion
The ‘if isset’ statement in PHP is a powerful tool for checking if a variable is set or not. IT helps prevent errors and gives you better control over the flow of your program. By using this statement, you can enhance code readability, making IT easier to understand and maintain your codebase.
FAQs
Q: What is the difference between isset() and empty()?
A: The isset() function checks if a variable is set and is not null, while the empty() function checks if a variable is empty, which includes being set to null, an empty string, or the value 0.
Q: Can I use isset() to check if an array element is set?
A: Yes, isset() can be used to check if a specific element within an array is set. You can simply specify the array and the element index or key within the isset() function.
Q: Is IT necessary to use isset() before accessing the value of a variable?
A: IT is not always necessary to use isset() before accessing the value of a variable. However, IT is generally considered a good practice to check if a variable is set before using IT to avoid any potential errors.
Q: Can I combine multiple variables in a single isset() statement?
A: Yes, you can combine multiple variables in a single isset() statement by separating them with commas.
Q: Are there any performance implications of using isset()?
A: Using isset() has minimal performance impact, and IT is unlikely to cause any significant slowdown in your code execution.