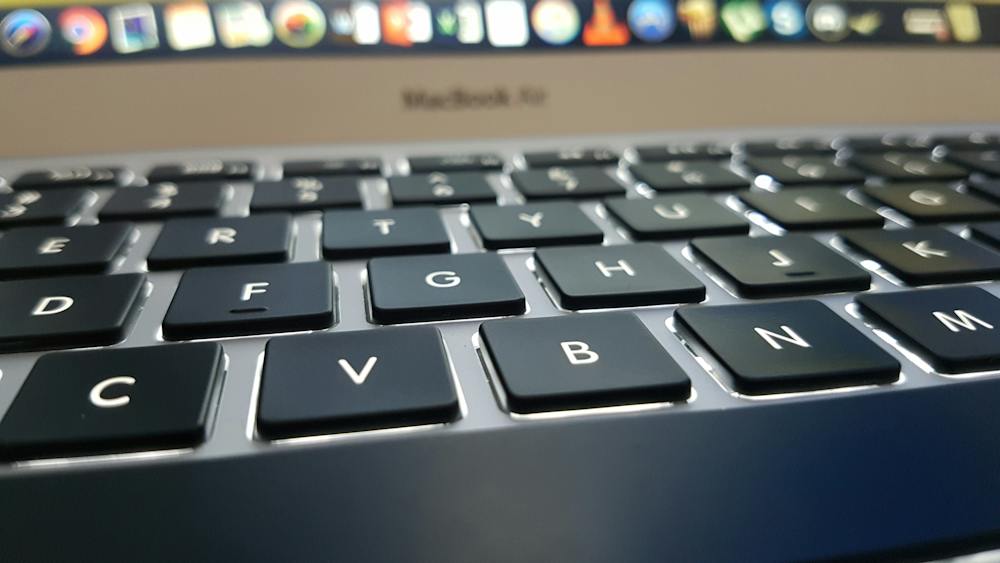
Introduction
Python, a powerful and versatile programming language, has gained immense popularity among developers. Its simplicity, readability, and extensive libraries make IT an excellent choice for both beginners and experienced programmers.
In this article, we will explore ten basic Python programs that every beginner should try. These programs will not only help you grasp the fundamental concepts of Python but also provide a hands-on experience that boosts your programming skills. So, let’s dig into Python’s best-kept secrets!
1. Hello, World!
Every programming tutorial begins with the classic “Hello, World!” program. IT‘s the simplest way to get a taste of Python’s syntax. This program prints a welcoming message to the console:
print("Hello, World!")
By writing this program, you’ll learn how to use Python’s print function, which displays the specified text on the console.
2. Calculating the Area of a Circle
Next, let’s dive into a mathematical example by calculating the area of a circle. This program prompts the user to enter the radius of the circle and computes its area:
import math
radius = float(input("Enter the radius of the circle: "))
area = math.pi * radius ** 2
print("The area of the circle is:", area)
Here, we utilize the math library and its constant math.pi
to calculate the area. IT‘s a practical program that showcases the use of variables, user input, and mathematical operations in Python.
3. Checking Prime Numbers
Moving on, let’s create a program that checks whether a given number is prime or not. This program prompts the user to enter a number and verifies its prime status:
num = int(input("Enter a number: "))
if num > 1:
for i in range(2, int(math.sqrt(num)) + 1):
if (num % i) == 0:
print(num, "is not a prime number.")
break
else:
print(num, "is a prime number.")
else:
print(num, "is not a prime number.")
This program demonstrates the use of conditional statements and loops in Python. IT provides an opportunity to understand how logic is implemented in programming.
4. Generating Fibonacci Series
Fibonacci series is a popular sequence of numbers where each number is the sum of the two preceding ones. Let’s build a program that generates the Fibonacci series up to a specified limit:
limit = int(input("Enter the limit: "))
# Initializing the first two numbers
a, b = 0, 1
fib_series = []
while a < limit:
fib_series.append(a)
a, b = b, a + b
print("Fibonacci Series:")
for num in fib_series:
print(num)
In this program, we use a while loop to generate Fibonacci numbers up to the specified limit. IT showcases the use of lists and demonstrates the power of loops in Python programming.
5. Counting Words in a Sentence
Now, let’s create a program that counts the number of words in a given sentence. This program prompts the user to enter a sentence and returns its word count:
sentence = input("Enter a sentence: ")
word_count = len(sentence.split())
print("Word count:", word_count)
This program demonstrates the use of string manipulation and the split function, which divides the sentence into words based on spaces. IT‘s a small yet powerful program that shows how Python can handle textual data.
6. Simple User Login System
To add interactivity, let’s build a simple user login system. This program asks the user to enter their username and password, then validates the input:
username = input("Enter your username: ")
password = input("Enter your password: ")
if username == "admin" and password == "password":
print("Login successful!")
else:
print("Invalid credentials. Login failed.")
This program showcases the use of conditional statements to determine if the user input matches the predefined values. IT offers a glimpse into building basic interactive systems with Python.
7. Sorting a List of Numbers
Sorting is an essential operation in programming. Let’s create a program that sorts a list of numbers in ascending order using the built-in sort
function:
numbers = [4, 2, 8, 5, 1, 9]
numbers.sort()
print("Sorted numbers:", numbers)
This program demonstrates how Python’s sort
function can arrange a list of numbers in ascending order. IT highlights the convenience of using built-in functions for common tasks.
8. Creating a Simple Calculator
A calculator program adds a touch of complexity to your learning journey. Let’s build a simple calculator that performs four basic arithmetic operations:
num1 = float(input("Enter the first number: "))
operator = input("Enter the operator (+, -, *, /): ")
num2 = float(input("Enter the second number: "))
if operator == "+":
print("Result:", num1 + num2)
elif operator == "-":
print("Result:", num1 - num2)
elif operator == "*":
print("Result:", num1 * num2)
elif operator == "/":
print("Result:", num1 / num2)
else:
print("Invalid operator. Please try again.")
This program introduces user-defined input, conditional statements, and mathematical operations to create a functioning calculator. IT‘s a stepping stone towards more complex projects.
9. Working with Files
File handling is a crucial aspect of programming. Let’s create a program that reads a text file and displays its contents:
with open("sample.txt", "r") as file:
content = file.read()
print("File contents:")
print(content)
In this program, we use the with open
statement to handle file operations. IT showcases how Python’s file handling capabilities simplify reading and manipulating external data.
10. Creating a Simple Web Scraping Tool
Lastly, let’s dive into web scraping by creating a simple program that extracts data from a Website. Although this program requires additional libraries, IT demonstrates the real-world application of Python:
import requests
from bs4 import BeautifulSoup
# Request the webpage
url = "https://www.example.com"
response = requests.get(url)
# Parse the HTML content
soup = BeautifulSoup(response.content, "html.parser")
# Extract relevant data
title = soup.title.string
print("Website title:", title)
This program uses the requests
library to fetch the HTML content of a webpage and the BeautifulSoup
library to parse and extract relevant data. Although IT requires additional knowledge, web scraping opens the door to exciting possibilities.
Conclusion
Python offers an incredible range of possibilities, and these ten basic programs only scratch the surface. By experimenting with these examples, you’ll gain hands-on experience and a solid foundation in Python programming.
Remember to continuously challenge yourself, explore additional libraries and frameworks, and keep discovering the best-kept secrets of Python. Happy coding!
FAQs
1. Can I learn Python programming as a beginner?
Absolutely! Python is widely regarded as one of the best programming languages for beginners. Its readability and simplicity make IT easy to understand and learn.
2. Do I need any prior coding experience to learn Python?
No, you don’t need any prior coding experience to learn Python. IT‘s an excellent choice for beginners as IT provides a gentle learning curve and extensive documentation.
3. Which Python version should I use?
There are currently two major versions of Python, namely Python 2 and Python 3. IT is recommended to use Python 3 as Python 2 will soon reach its end of life and is no longer actively supported.
4. Can Python be used for web development?
Yes, Python can be used for web development. Popular web frameworks like Django and Flask allow developers to build robust and scalable web applications using Python.
5. Are there any job opportunities for Python developers?
Absolutely! Python is widely used in various industries, making IT a highly sought-after skill. Python developers are in demand for web development, data analysis, machine learning, and many other domains.
6. How can I continue learning Python?
There are numerous resources available to continue your Python learning journey. Online tutorials, documentation, coding challenges, and joining developer communities can greatly enhance your skills.