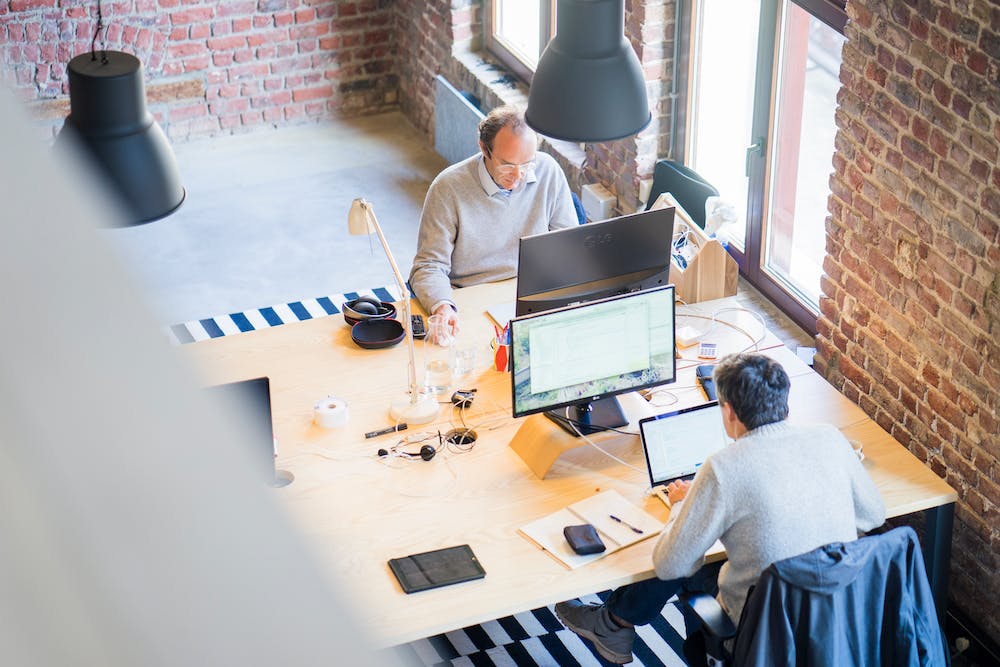
Introduction
The Fibonacci sequence is a fascinating mathematical concept that has intrigued mathematicians and enthusiasts
alike for centuries. Discovered by Leonardo Fibonacci, an Italian mathematician, in the early 13th century, this
sequence has numerous applications in various fields, such as mathematics, nature, and computer science.
In this article, we will delve into the magic of Fibonacci numbers, understand their properties, and explore an
astonishing Python program that generates the Fibonacci sequence with elegance and efficiency.
The Fibonacci Sequence
The Fibonacci sequence is a series of numbers in which each number (except for the first two) is the sum of the
two preceding ones. IT starts with 0 and 1, and the subsequent numbers are calculated by adding the previous two
numbers. Thus, the sequence begins: 0, 1, 1, 2, 3, 5, 8, 13, 21, and so on.
These numbers have numerous intriguing properties and connections to various phenomena in nature, art, and
science. They appear in the branching patterns of trees, the arrangement of leaves on stems, the formation of
pinecones, the spirals of seashells, and even the breeding patterns of rabbits, among others. This unique
mathematical sequence has captivated mathematicians for centuries and continues to be a subject of fascination
and exploration.
Python Program for Fibonacci Sequence
Python, being a versatile and powerful programming language, allows us to create elegant and efficient programs
to generate the Fibonacci sequence. Here’s an astonishing Python program that utilizes recursion to generate
the Fibonacci series:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
# Test the program
terms = 10 # Number of terms in the Fibonacci sequence
if terms <= 0:
print("Number of terms should be a positive integer.")
else:
print("Fibonacci sequence:")
for i in range(terms):
print(fibonacci(i))
In the above program, we define the Fibonacci function that takes an input, n
, and recursively
calculates the Fibonacci number at location n
in the sequence. IT starts with the base case where
n
is either 0 or 1, returning the same value. For any other value of n
, IT recursively
calls itself with n-1
and n-2
as arguments, and finally returns the sum of the two
results.
To test the program, we set the variable terms
to the desired number of terms we want in the
Fibonacci sequence. The program then checks if the number of terms is a positive integer. If so, IT proceeds to
print the Fibonacci sequence by calling the Fibonacci function for each value from 0 to terms-1
.
Conclusion
The Fibonacci sequence is an enchanting mathematical concept that has fascinated mathematicians, scientists, and
nature enthusiasts for centuries. Its simple yet remarkable properties make IT ubiquitous in various aspects of
the natural world and its applications spread to many domains, including computer science.
We explored an astonishing Python program that generates the Fibonacci sequence effortlessly using recursion. This
program showcases the power of Python in expressing intricate mathematical concepts in concise and elegant code.
FAQs
Q: Can the Fibonacci sequence arise in any other context apart from mathematics?
A: Absolutely! The Fibonacci sequence appears in various fields such as architecture, art, music, and even in the
financial world. For instance, architects and designers often use Fibonacci numbers to create visually pleasing
proportions in buildings and structures.
Q: What is the significance of the Fibonacci sequence in nature?
A: The Fibonacci sequence appears in numerous natural phenomena, including the spiral arrangement of leaves,
petals, and seeds in plants and flowers. This arrangement allows optimal exposure to sunlight and efficient use
of space, making IT a common pattern in nature’s design.
Q: Can the Fibonacci sequence be generated iteratively instead of using recursion?
A: Yes, IT is possible to generate the Fibonacci sequence using an iterative approach instead of recursion. IT can
be achieved by initializing two variables to store the previous two numbers and then iterating through a loop to
generate the subsequent numbers.
Q: Are there any practical applications of the Fibonacci sequence in computer science?
A: Yes, the Fibonacci sequence finds its applications in various areas of computer science such as algorithm
design, distributed computing, and data structures. IT serves as a fundamental example for understanding
recursion and dynamic programming, both of which are crucial concepts in computer science.