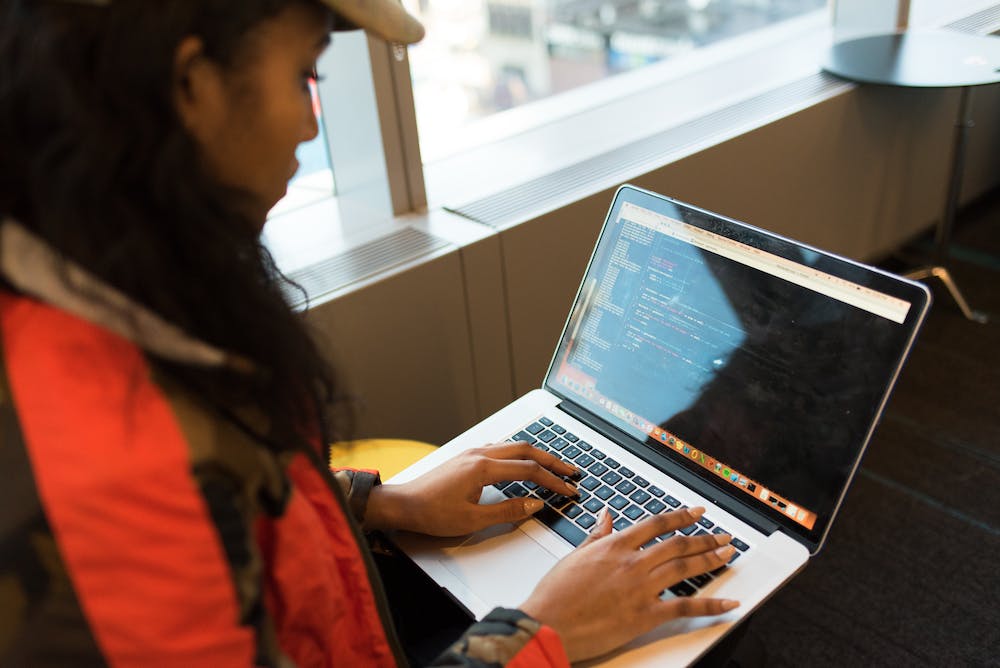
Logistic regression is a widely used statistical analysis technique that is primarily used to predict binary outcomes. IT is a classification algorithm that helps to determine the probability of an event occurring based on the input variables. In this article, we will delve into the intricacies of logistic regression and provide you with a comprehensive Python code example to better understand its implementation.
Understanding Logistic Regression
Logistic regression is an extension of linear regression. While linear regression predicts continuous numerical values, logistic regression deals with binary outcomes. IT is commonly used for tasks such as spam detection, predicting customer churn, or determining the likelihood of a disease based on medical data.
The key concept behind logistic regression is the logistic function (also known as the sigmoid function), which maps any real-valued number into a value between 0 and 1. This mapping allows us to interpret the output as a probability of the event being positive or negative.
The sigmoid function is defined as:
def sigmoid(z):
return 1 / (1 + math.exp(-z))
Logistic regression uses a set of input features (also known as independent variables) to calculate the weighted sum, often called the logit or linear predictor. The weighted sum is then passed through the sigmoid function to obtain the predicted probability.
Python Code Example for Logistic Regression
Let’s dive into a Python code example that demonstrates logistic regression. In this example, we will use the scikit-learn library, which provides a user-friendly interface for implementing various machine learning algorithms, including logistic regression.
# Importing the required libraries
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Creating the logistic regression model
model = LogisticRegression()
# Splitting the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Fitting the model on the training data
model.fit(X_train, y_train)
# Predicting the outcomes for the test set
y_pred = model.predict(X_test)
# Evaluating the accuracy of the model
accuracy = accuracy_score(y_test, y_pred)
In the code above, we first import the necessary libraries, including the LogisticRegression class from scikit-learn. We then create an instance of the logistic regression model. Next, we split our dataset into training and testing sets using the train_test_split function. This ensures that we have data to train the model on and data to evaluate its performance.
We then fit the model on the training data using the fit method. Once the model is trained, we use IT to predict the outcomes for the test set. Finally, we evaluate the accuracy of our model using the accuracy_score function, which compares the predicted outcomes with the actual outcomes.
Conclusion
Logistic regression is a powerful classification algorithm that can be implemented using various programming languages, including Python. IT allows us to predict binary outcomes by mapping inputs to probabilities using the sigmoid function. By understanding the concept of logistic regression and its implementation in Python, you can leverage this technique for a wide range of applications.
FAQs
Q: What is the difference between linear regression and logistic regression?
A: Linear regression predicts continuous numerical values, while logistic regression predicts binary outcomes.
Q: What is the sigmoid function?
A: The sigmoid function is an S-shaped curve that maps any real-valued number into a value between 0 and 1.
Q: What libraries can be used to implement logistic regression in Python?
A: Python provides various libraries for implementing logistic regression, such as scikit-learn, TensorFlow, and PyTorch.
Q: What is the importance of logistic regression in machine learning?
A: Logistic regression is widely used in machine learning for binary classification tasks, making IT an important algorithm for various applications.
Q: How can logistic regression be evaluated?
A: Logistic regression models can be evaluated using metrics such as accuracy, precision, recall, and area under the receiver operating characteristic curve (AUC-ROC).