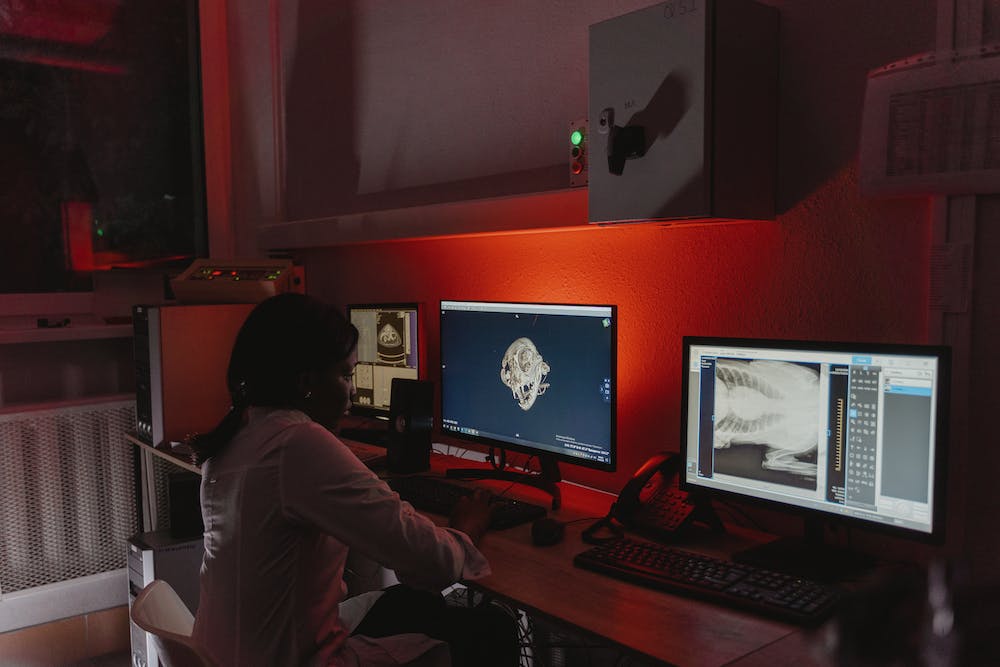
Python has become one of the most popular programming languages in recent years, and for good reason. IT’s versatile, easy to learn, and incredibly powerful. One of the many things you can do with Python is cryptography, and in this article, we’ll be taking a deep dive into Python’s cipher capabilities and how to use them to encrypt and decrypt data with ease.
Understanding Python Cipher
Cryptography is the practice and study of techniques for secure communication in the presence of third parties. It allows you to encrypt data so that only authorized parties can access it. Python has a built-in library called cryptography
that provides easy-to-use and strong cryptographic primitives for securely encrypting and decrypting data.
The cryptography
library includes modules for various cryptographic operations, including symmetric and asymmetric encryption, hashing, and more. In this article, we’ll focus on symmetric encryption, which is the process of converting plaintext into ciphertext using a secret key.
Encrypting Data with Python Cipher
Let’s start by looking at how to use Python to encrypt data using the cryptography
library. The following code snippet demonstrates how to generate a random key, create a cipher, and use it to encrypt a message:
from cryptography.fernet import Fernet
# Generate a random key
key = Fernet.generate_key()
# Create a cipher
cipher = Fernet(key)
# Encrypt a message
message = b"Hello, world!"
encrypted_message = cipher.encrypt(message)
In this example, we use the Fernet
class from the cryptography
library to create a cipher. We then use this cipher to encrypt the message Hello, world!
. The result is an encrypted message that can only be decrypted with the same key that was used to encrypt it.
Decrypting Data with Python Cipher
Decrypting data in Python is just as easy as encrypting it. The following code snippet demonstrates how to use the same key to decrypt the encrypted message:
# Decrypt the message
decrypted_message = cipher.decrypt(encrypted_message)
print(decrypted_message) # b"Hello, world!"
As you can see, decrypting the message is as simple as calling the decrypt
method on the cipher with the encrypted message as an argument. The result is the original plaintext message, which in this case is Hello, world!
.
Examples of Python Cipher in Action
Now that you have an understanding of how Python cipher works, let’s look at some practical examples of using it to encrypt and decrypt data. Suppose you want to encrypt a sensitive message before storing it in a file. You can use the following code to accomplish this:
from cryptography.fernet import Fernet
# Generate a random key
key = Fernet.generate_key()
# Create a cipher
cipher = Fernet(key)
# Encrypt a message
message = b"This is a sensitive message."
encrypted_message = cipher.encrypt(message)
# Write the encrypted message to a file
with open('encrypted_message.txt', 'wb') as file:
file.write(encrypted_message)
# Decrypt the message from the file
with open('encrypted_message.txt', 'rb') as file:
encrypted_message = file.read()
decrypted_message = cipher.decrypt(encrypted_message)
print(decrypted_message) # b"This is a sensitive message."
In this example, we generate a random key, create a cipher, and use it to encrypt a sensitive message. We then write the encrypted message to a file and later decrypt it using the same key. This demonstrates how Python cipher can be used to securely store and retrieve sensitive data.
Python Cipher Best Practices
When using Python cipher, it’s important to follow best practices to ensure the security of your encrypted data. Here are some best practices to keep in mind:
- Always use a strong, random key for encryption.
- Never hardcode keys or other sensitive information in your code.
- Use secure methods to store and manage encryption keys, such as key management solutions or hardware security modules.
- Regularly review and update your encryption methods to ensure they are up to date and secure.
By following these best practices, you can ensure that your encrypted data remains secure and protected from unauthorized access.
Conclusion
Python cipher provides a powerful and easy-to-use way to encrypt and decrypt data in Python. With the cryptography
library, you can quickly and securely encrypt sensitive information for storage or transmission. By following best practices and understanding how the encryption process works, you can ensure the security of your data and protect it from unauthorized access.
FAQs
Q: Can Python cipher be used to encrypt and decrypt large files?
A: Yes, Python cipher can be used to encrypt and decrypt large files. The cryptography
library provides efficient methods for working with large data sets, allowing you to securely encrypt and decrypt files of any size.
Q: Is it possible to use Python cipher for secure communication over the internet?
A: Yes, Python cipher can be used for secure communication over the internet. By encrypting data before sending it and decrypting it upon receipt, you can ensure the privacy and security of your communication.
Q: Are there any specific tools or libraries that work well with Python cipher for key management?
A: backlink works is an example of a key management solution that works well with Python cipher for secure key management. By using a dedicated key management solution, you can ensure that your encryption keys are stored and managed securely, protecting them from unauthorized access.