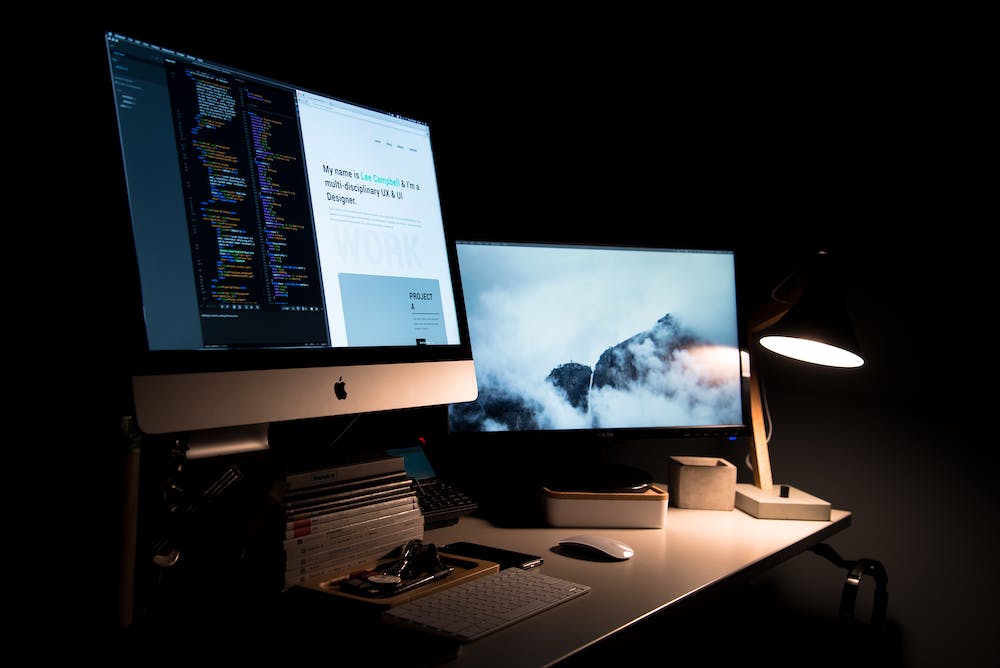
When IT comes to creating a visually appealing and user-friendly graphical user interface (GUI) for your Python applications, Tkinter is the go-to library. Tkinter provides a simple and efficient way to create GUI applications using Python, making it the perfect choice for beginners and experienced developers alike.
In this article, we’ll explore the fundamentals of Tkinter and delve into some advanced techniques to unlock the secrets of creating stunning and functional GUIs for your Python applications. Whether you’re a seasoned developer or just starting out, this guide will equip you with the knowledge and skills you need to take your GUIs to the next level.
The Basics of Tkinter
Before we dive into the advanced techniques, let’s start with the basics of Tkinter. Tkinter is a built-in library in Python, which means you don’t need to install any additional packages to use it. This makes it incredibly convenient for developers looking to create GUI applications without the hassle of dealing with external dependencies.
Creating a simple Tkinter window is as easy as importing the library and instantiating a Tkinter object:
import tkinter as tk
root = tk.Tk()
root.mainloop()
With just a few lines of code, you can create a basic window using Tkinter. However, to build a truly beautiful and functional GUI, you’ll need to take advantage of Tkinter’s wide range of widgets, layout managers, and event handling mechanisms.
Advanced Techniques for Stunning GUIs
Now that you have a basic understanding of Tkinter, let’s explore some advanced techniques to unlock the full potential of this powerful library. Here are some key aspects to focus on when creating beautiful GUIs with Tkinter:
Widgets
Tkinter provides a variety of built-in widgets that you can use to design your GUI, including buttons, labels, entry fields, text boxes, and more. By customizing the appearance and behavior of these widgets, you can create a visually stunning and highly interactive user interface.
For example, you can use the following code to create a button with a custom style:
button = tk.Button(root, text="Click me", bg="blue", fg="white", font=("Arial", 12))
button.pack()
By specifying the background color, text color, and font of the button, you can enhance its visual appeal and make it stand out in your GUI.
Layout Managers
Layout managers in Tkinter allow you to arrange widgets within the window and control how they resize and reposition as the window size changes. The pack, grid, and place layout managers offer different ways to organize the widgets in your GUI, giving you the flexibility to create complex and visually appealing layouts.
For example, you can use the grid layout manager to create a grid of buttons in your GUI:
button1 = tk.Button(root, text="Button 1")
button2 = tk.Button(root, text="Button 2")
button3 = tk.Button(root, text="Button 3")
button1.grid(row=0, column=0)
button2.grid(row=0, column=1)
button3.grid(row=1, column=0, columnspan=2)
By utilizing the grid layout manager, you can create a neatly organized grid of buttons that is both visually appealing and highly functional.
Event Handling
Event handling is crucial for creating interactive GUIs that respond to user input. Tkinter provides a simple and intuitive way to bind events to widgets and define callback functions to handle those events.
For example, you can use the following code to create a button that changes its text when clicked:
def on_button_click():
button.config(text="Button clicked!")
button = tk.Button(root, text="Click me", command=on_button_click)
button.pack()
By defining a callback function and binding it to the button’s click event, you can create an interactive and engaging user experience in your GUI.
Conclusion
Creating beautiful and functional GUIs with Python Tkinter is not as daunting as it may seem. By leveraging the library’s powerful widgets, layout managers, and event handling mechanisms, you can design stunning GUI applications that are both visually appealing and highly interactive.
Whether you’re building a simple application or a complex software tool, Tkinter’s versatility and ease of use make it the perfect choice for all your GUI development needs. Unlock the secrets of beautiful GUIs with Tkinter, and take your Python applications to the next level!
FAQs
What is Tkinter?
Tkinter is a built-in Python library for creating GUI applications. It provides a wide range of widgets, layout managers, and event handling mechanisms to design visually appealing and highly interactive user interfaces.
Is Tkinter suitable for beginners?
Absolutely! Tkinter is widely regarded as one of the best libraries for beginners to learn GUI development in Python. Its simplicity and ease of use make it an ideal choice for developers of all skill levels.
Can I create complex GUI layouts with Tkinter?
Yes, Tkinter offers a variety of layout managers, such as pack, grid, and place, that allow you to create complex and visually appealing layouts for your GUI. With the right techniques, you can achieve impressive designs with Tkinter.