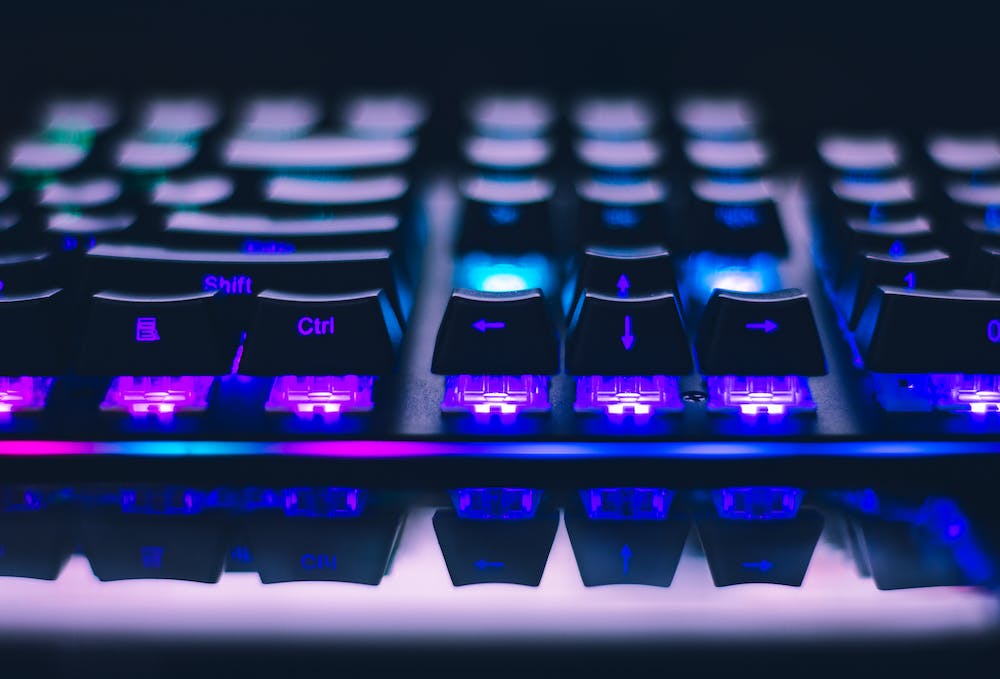
In the world of web development, JavaScript plays a vital role in bringing websites and web applications to life. The language continues to evolve, constantly introducing new features to make coding more efficient and powerful. One of the most exciting updates in recent years is the release of ECMAScript 2016, also known as ES7. This version introduces several mind-blowing features that will revolutionize your code and take your JavaScript skills to the next level.
1. Array.prototype.includes()
Working with arrays in JavaScript has become even easier with the introduction of the includes()
method. This method allows you to check if an array contains a specific element, eliminating the need for traditional indexOf()
checks.
const colors = ['red', 'green', 'blue'];
console.log(colors.includes('green')); // true
console.log(colors.includes('yellow')); // false
This feature enhances code readability and simplifies array manipulation, making IT a game-changer for developers.
2. Exponentiation Operator
Performing mathematical calculations with JavaScript has become more convenient with the exponentiation operator (**
). IT allows you to calculate the exponent of a number easily without the need for complex code.
const squared = 2 ** 2; // 4
const cubed = 2 ** 3; // 8
const toThePowerOf = 2 ** 4; // 16
This operator provides a concise and intuitive way to work with exponential calculations, improving code efficiency and readability.
3. Async/Await
Promises have been a core feature in JavaScript for handling asynchronous operations. ES7 takes promises to the next level with the introduction of async/await, a powerful and intuitive way to write asynchronous code.
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Data fetched successfully!');
}, 2000);
});
}
async function fetchDataAsync() {
try {
const result = await fetchData();
console.log(result);
} catch (error) {
console.log(error);
}
}
fetchDataAsync();
By using the async
and await
keywords, you can write asynchronous code as if IT were synchronous. This makes code easier to read, write, and maintain.
4. Object.values() and Object.entries()
ES7 introduces two new methods, Object.values()
and Object.entries()
, which provide a convenient way to extract values and key-value pairs from objects.
const person = {
name: 'John',
age: 30,
city: 'New York'
};
const values = Object.values(person);
console.log(values); // ['John', 30, 'New York']
const entries = Object.entries(person);
console.log(entries); // [['name', 'John'], ['age', 30], ['city', 'New York']]
These methods eliminate the need for manual looping over object properties, simplifying the retrieval of values and pairs in a more efficient way.
5. String padding
String manipulation is a common task in JavaScript, and ES7 introduces two helper methods, padStart()
and padEnd()
, to easily add padding to strings.
const name = 'John';
const paddedName = name.padStart(10, '-'); // '------John'
console.log(paddedName);
const amount = '99.99';
const formattedAmount = amount.padEnd(10, '0'); // '99.990000'
console.log(formattedAmount);
These methods allow you to control the length of strings by adding characters at the beginning or end, ensuring consistent formatting and alignment.
Conclusion
Javascript ES7 introduces several powerful features that can greatly enhance your coding experience. The includes()
method makes array manipulation more straightforward, while the exponentiation operator simplifies mathematical calculations. The async/await syntax streamlines asynchronous operations, and the Object.values()
and Object.entries()
methods provide easier access to object properties. Lastly, the string padding methods enable consistent and controlled string formatting.
By embracing these ES7 features, you can write cleaner, more efficient, and easier-to-maintain code, ultimately revolutionizing the way you approach web development.
FAQs
1. Can I use these ES7 features in all browsers?
While ES7 features are progressively becoming supported in modern browsers, IT‘s important to consider the browser compatibility of these features. To ensure cross-browser compatibility, you can use transpilers like Babel to convert your ES7 code to older ES5 syntax.
2. Are there any performance implications when using these new features?
Generally, the performance impact of using ES7 features is negligible. The JavaScript engines in modern browsers are optimized to handle these new features efficiently. However, IT‘s always good practice to test and profile your code to ensure optimal performance.
3. Can I start using these features in my projects right away?
Yes, you can start using these ES7 features in your projects. However, IT‘s important to consider the browsers or environments you’re targeting and whether they support these features. If you’re developing for older browsers, make sure to use transpilers like Babel to ensure compatibility.
4. Are there any other notable ES7 features?
Yes, apart from the features mentioned in this article, ES7 also introduces the String.prototype.includes()
method, which enables easy substring checks. Additionally, the exponentiation operator can be used with variables and expressions, providing dynamic computations.
5. How can I learn more about JavaScript ES7?
To gain a deeper understanding of JavaScript ES7 and its features, you can refer to the ECMAScript specification (https://www.ecma-international.org/ecma-262/7.0/). Additionally, online resources, tutorials, and books dedicated to ES7 can provide valuable insights and examples.