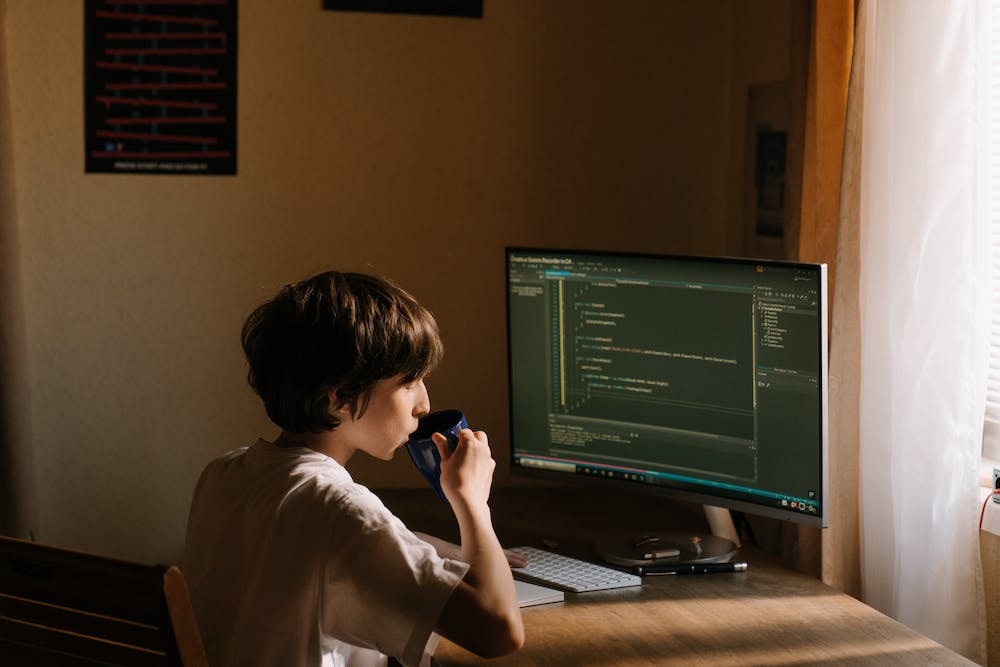
JavaScript is the most popular programming language in the world, and for good reason. IT powers the interactive elements of nearly every Website and web application, making it an essential skill for any developer.
With the release of ES6 (ECMAScript 2015), JavaScript received a major update, introducing a range of new features and syntax improvements that greatly enhance the language’s capabilities. In this article, we’ll explore some of the most powerful features of ES6 and how they can help you write cleaner, more efficient code.
Constants and Block-Scoped Variables
Prior to ES6, JavaScript had only two types of variable declarations: var
and function
. ES6 introduced two new ways to declare variables: let
and const
.
let: The let
keyword allows you to declare block-scoped variables, meaning they are only accessible within the block in which they are defined.
const: The const
keyword allows you to declare constants, whose values cannot be re-assigned once they are initialized.
Arrow Functions
Arrow functions provide a more concise syntax for writing function expressions in JavaScript. They are especially useful for writing callbacks and event handlers. Here’s an example of a traditional function and its equivalent using arrow syntax:
// Traditional function
function add(a, b) {
return a + b;
}
// Arrow function
const add = (a, b) => a + b;
Template Literals
Template literals allow you to embed expressions and variables inside strings, making it easier to create dynamic content. They are denoted by backticks (`
) instead of single or double quotes.
Here’s an example of using template literals to create a dynamic greeting:
const name = 'John';
const greeting = `Hello, ${name}!`;
console.log(greeting); // Output: Hello, John!
Destructuring Assignment
Destructuring assignment provides a concise way to extract values from arrays and objects and assign them to variables. It can greatly simplify your code by reducing the amount of boilerplate code needed to access nested data structures.
For example, you can use destructuring assignment to extract properties from an object:
const person = {
name: 'Jane Doe',
age: 30,
profession: 'Engineer'
};
const { name, profession } = person;
console.log(name, profession); // Output: Jane Doe Engineer
Spread and Rest Operators
The spread and rest operators are represented by the ellipsis (…) and provide a more flexible way to work with arrays and function arguments.
Spread Operator: The spread operator allows you to expand an array into individual elements, which can be useful for combining arrays or passing array elements as function arguments.
Rest Parameter: The rest parameter allows you to represent an indefinite number of arguments as an array, which can simplify function definitions that accept a variable number of arguments.
Modules
ES6 introduced a more standardized approach to working with modules in JavaScript. Modules allow you to organize your code into reusable and maintainable units, making it easier to manage large-scale projects.
By using the export
and import
keywords, you can define and import modules to structure your code in a more modular and organized way.
Classes
ES6 brought a more familiar and concise syntax for defining and working with classes in JavaScript. With the introduction of the class
keyword, you can now create class-based objects and inheritance hierarchies more easily.
Here’s an example of using classes to define a simple Person
class with a sayHello
method:
class Person {
constructor(name) {
this.name = name;
}
sayHello() {
console.log(`Hello, my name is ${this.name}.`);
}
}
const person = new Person('Alice');
person.sayHello(); // Output: Hello, my name is Alice.
Conclusion
ES6 brought a range of new features and syntax improvements to JavaScript, making it a more powerful and expressive programming language. By mastering the features of ES6, you can write cleaner, more efficient code and take your coding skills to the next level.
FAQs
Q: Will ES6 features work in all browsers?
A: Most modern browsers support ES6 features, but for older browsers, you may need to use a transpiler like Babel to convert ES6 code to ES5 for compatibility.
Q: Is it worth learning ES6 if I’m already comfortable with ES5?
A: Absolutely! ES6 introduces many new features and improvements that can significantly enhance your coding productivity and make your code more maintainable.
Q: Where can I learn more about ES6?
A: There are many online resources, tutorials, and courses available for learning ES6, including the official ECMAScript documentation and popular coding platforms like backlink works.
In conclusion, JavaScript ES6 is a powerful and versatile language that can greatly boost your coding skills. By mastering the features of ES6, you can write cleaner, more efficient code and stay at the forefront of modern web development.