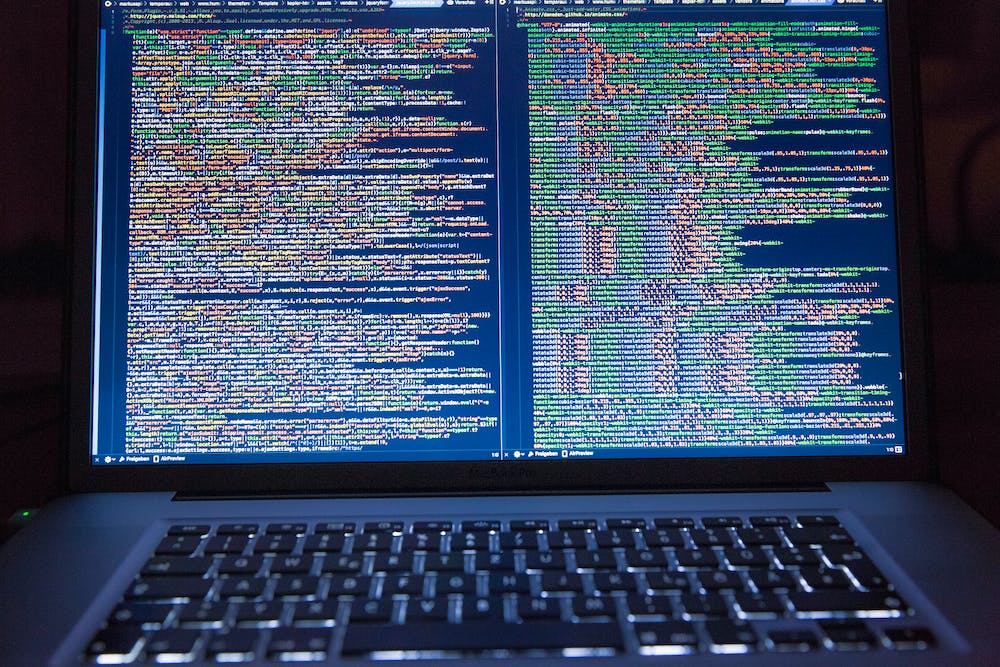
PHP is a powerful scripting language that is widely used for web development. One of its most important features is the ability to handle user input through various methods, including the _request superglobal. In this article, we will explore what _request is, how IT works, and how you can use it to enhance your web applications.
Understanding _request
_request is a superglobal variable in PHP that is used to collect data from HTML forms. It can handle data sent through both the GET and POST methods, making it a versatile tool for handling user input.
When a user submits a form on a web page, the data entered into the form is sent to the server. PHP then takes this data and stores it in the _request superglobal, making it accessible to the rest of the script.
GET vs. POST
As mentioned earlier, _request can handle data sent through both the GET and POST methods. The main difference between the two is how the data is sent to the server.
GET sends the data as part of the URL, while POST sends the data in the body of the HTTP request. GET is generally used for requests that do not change the state of the server, such as retrieving data from a database. POST, on the other hand, is used for requests that do change the state of the server, such as submitting a form or uploading a file.
Using _request in Your PHP Code
Now that we understand what _request is and how it works, let’s take a look at how you can use it in your PHP code.
When a form is submitted using either the GET or POST method, the data is stored in the _request superglobal. You can then access this data using the following syntax:
“`php
$value = $_REQUEST[‘fieldname’];
“`
Replace ‘fieldname’ with the name of the input field in your form. This will retrieve the value entered into that field and store it in the variable $value.
Example:
Let’s say you have a form with the following input fields:
“`html
“`
You can then access the data entered into these fields in your PHP code using _request:
“`php
$fullname = $_REQUEST[‘fullname’];
$email = $_REQUEST[’email’];
“`
Now you can use $fullname and $email to process the user’s input however you need to in your PHP code.
Security Considerations
While _request is a powerful tool for handling user input, it is important to be mindful of security considerations when using it in your PHP code.
Because _request can handle both GET and POST data, it is susceptible to various types of attacks, such as cross-site scripting (XSS) and SQL injection. To mitigate these risks, it is important to validate and sanitize the data received from _request before using it in your code.
You can use PHP’s filter_input() function to validate the data, and functions such as htmlspecialchars() and mysqli_real_escape_string() to sanitize it. By taking these precautions, you can ensure that your web application is secure and protected from potential threats.
Conclusion
PHP’s _request superglobal is a powerful tool for handling user input in web applications. By understanding how it works and using it effectively in your PHP code, you can create dynamic, interactive web forms that enhance the user experience.
However, it is important to be mindful of security considerations when using _request, and to validate and sanitize the data received from it to protect your web application from potential threats.
FAQs
What is the difference between _request and _GET/_POST?
_request is a combination of _GET and _POST, allowing you to access data sent through both methods in a single variable. This makes it a convenient option for handling user input in PHP.
How can I validate and sanitize data received from _request?
You can use PHP’s filter_input() function to validate the data received from _request, and functions such as htmlspecialchars() and mysqli_real_escape_string() to sanitize it. By taking these precautions, you can ensure that your web application is secure and protected from potential threats.
Can I use _request to handle file uploads?
No, _request is not suitable for handling file uploads. For file uploads, you should use the $_FILES superglobal instead.
Is it recommended to use _request for all user input in my PHP code?
While _request is a convenient option for handling user input, it is not always necessary to use it for all input in your PHP code. Consider the specific requirements of your web application and use _request where it makes sense, while also being mindful of security considerations and best practices for handling user input.