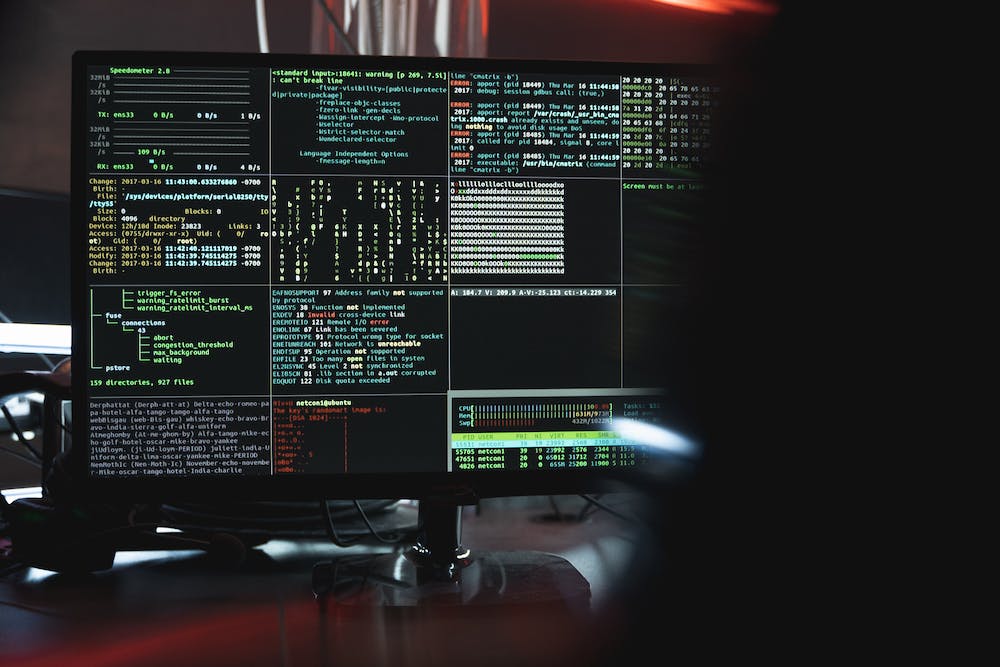
Introduction
What is Node.js?
Node.js is an open-source, cross-platform JavaScript runtime environment that allows you to execute JavaScript code outside of the browser. IT uses an event-driven, non-blocking I/O model which makes IT perfect for building scalable and efficient network applications. Node.js is built on the V8 JavaScript engine, the same engine that powers Google Chrome, offering superior performance and speed.
Step 1: Installation
The first step to mastering Node.js is to install IT on your machine. Node.js can be downloaded from the official Website (https://nodejs.org) and IT provides installers for various operating systems including Windows, macOS, and Linux. Once the installation is complete, you can verify that Node.js is correctly installed by opening a terminal or command prompt and running the command:
node --version
This will display the installed version of Node.js, confirming that the installation was successful.
Step 2: NPM (Node Package Manager)
NPM is the default package manager for Node.js and IT is an essential tool for managing packages and dependencies in your Node.js projects. NPM allows you to easily install, update, and uninstall packages from the command line. To check if NPM is installed, run:
npm --version
This will display the installed version of NPM. If NPM is not installed, you can install IT by running the following command:
npm install -g npm
Once NPM is installed, you can start using IT to install packages and manage your Node.js projects.
Step 3: Building a Simple Server
Node.js is commonly used for building web servers, and in this step, we will build a simple HTTP server using the built-in `http` module. Create a new file named `server.js` and add the following code:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'content-Type': 'text/plain' });
res.end('Hello, world!');
});
server.listen(3000, () => {
console.log('Server is listening on port 3000');
});
This code creates an HTTP server that listens on port 3000. When a request is received, IT responds with a plain text message saying ‘Hello, world!’. Save the file and run IT using Node.js:
node server.js
Now, if you open a web browser and visit `http://localhost:3000`, you will see the ‘Hello, world!’ message. Congratulations, you have built your first Node.js server!
Step 4: Working with Modules
Node.js uses a module system that allows you to modularize your code and reuse functionality across different files. In this step, we will create a simple module and use IT in our server. Create a new file named `greeting.js` and add the following code:
module.exports = {
sayHello: function() {
return 'Hello, from the greeting module!';
}
};
This code exports an object with a `sayHello` function. Now, go back to `server.js` and modify IT as follows:
const http = require('http');
const greeting = require('./greeting');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'content-Type': 'text/plain' });
res.end(greeting.sayHello());
});
server.listen(3000, () => {
console.log('Server is listening on port 3000');
});
Now, when you run the server again and visit `http://localhost:3000`, IT will display the greeting message from the `greeting.js` module.
Step 5: Asynchronous Programming
One of the key features of Node.js is its ability to handle asynchronous operations efficiently. In this step, we will demonstrate how to perform an asynchronous operation using the `fs` module to read a file. Create a new file named `fileReader.js` and add the following code:
const fs = require('fs');
fs.readFile('myfile.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
This code reads the contents of a file named `myfile.txt` and logs IT to the console. Make sure you have a file named `myfile.txt` in the same directory as `fileReader.js`. Run the code using Node.js:
node fileReader.js
The contents of the file will be printed to the console. This example demonstrates how Node.js handles I/O operations asynchronously, allowing your code to continue executing while waiting for the operation to complete.
Conclusion
Node.js is a powerful tool for building server-side applications using JavaScript. In this article, we covered five easy steps to help you get started with Node.js. We installed Node.js, learned about NPM, built a simple server, worked with modules, and explored asynchronous programming.
By mastering Node.js, you can unlock the full potential of JavaScript and build scalable, efficient, and high-performance server applications.
FAQs
Q: Is Node.js only for building servers?
A: No, Node.js is not limited to building servers. IT can be used for various purposes such as command-line scripts, desktop applications, and even IoT (internet of Things) devices.
Q: Are there any alternatives to NPM?
A: Yes, there are alternative package managers for Node.js such as Yarn and pnpm. These package managers offer some additional features and improvements over NPM.
Q: Can I use Node.js with front-end frameworks like React or Angular?
A: Yes, Node.js can be used alongside front-end frameworks to create full-stack applications. IT can be used as a backend server while React, Angular, or other front-end frameworks handle the client-side rendering.
Q: Are there any performance considerations when using Node.js?
A: While Node.js is known for its performance and scalability, IT may not be the best choice for all use cases. IT excels in handling concurrent connections and I/O-heavy operations but may not be the best fit for CPU-intensive tasks.
Q: Where can I find more resources to learn Node.js?
A: There are numerous online tutorials, documentation, and video courses available to learn Node.js. Some popular resources include the official Node.js documentation (https://nodejs.org), Node.js Tutorials on W3Schools (https://www.w3schools.com/nodejs/), and interactive platforms like Codecademy and Udemy.