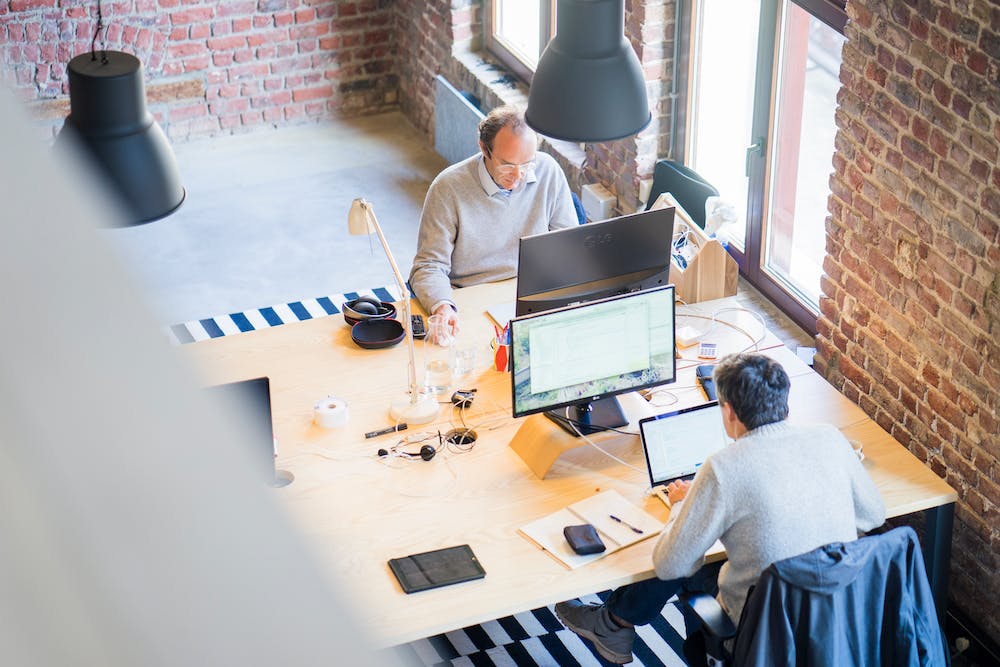
Introduction
Python, the versatile programming language, has become immensely popular among developers due to its simplicity, readability, and extensive collection of libraries. IT is often referred to as “the snake code” due to its association with the snake logo of the Python programming language. In this article, we will explore some hidden secrets and mind-blowing tricks of Python programming, unveiling the true potential of this powerful language.
Python’s Hidden Secrets
Python is packed with hidden secrets that can save your time and effort while coding. Let’s dive into some of these secrets:
1. Magic Methods
Magic methods in Python are special methods that start and end with a double underscore (dunder). These methods provide functionality to classes, allowing you to define how objects of the class behave in various scenarios. For example, implementing the __str__()
magic method enables you to customize the string representation of an object when printed.
Example:
class Person:
def __init__(self, name):
self.name = name
def __str__(self):
return f"Person: {self.name}"
p = Person("John")
print(p) # Output: Person: John
2. List Comprehension
List comprehension is a concise way to create lists in Python. IT allows you to generate a new list based on an existing list, applying transformations or filters to the elements.
Example:
numbers = [1, 2, 3, 4, 5]
squared_numbers = [x**2 for x in numbers]
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
3. Context Managers
Context managers provide an efficient way to allocate and release resources in Python. They ensure that resources are properly managed even in the presence of exceptions. The with
statement is used to define a context manager.
Example:
with open("file.txt", "r") as file:
data = file.read()
# After the block of code, the file is automatically closed
4. Decorators
Decorators allow you to modify the behavior of a function by wrapping IT with another function. They provide a clean and concise way to add functionality to existing functions without modifying their code.
Example:
def uppercase_decorator(function):
def wrapper():
result = function()
return result.upper()
return wrapper
@uppercase_decorator
def say_hello():
return "Hello, World!"
print(say_hello()) # Output: HELLO, WORLD!
Mind-Blowing Tricks in Python
Python not only hides secrets but also offers some mind-blowing tricks that can enhance your coding experience. Let’s explore a few of them:
1. Unpacking
Unpacking allows you to assign multiple values from a list or tuple to their respective variables in a single line of code. This feature can be handy when working with functions that return multiple values.
Example:
numbers = [1, 2, 3]
a, b, c = numbers
print(a, b, c) # Output: 1 2 3
2. Enumerate
The enumerate()
function in Python generates an index along with the elements of an iterable. IT provides a convenient way to iterate over both the indices and values of a list or any other iterable object.
Example:
fruits = ["apple", "banana", "orange"]
for index, fruit in enumerate(fruits):
print(f"Index: {index}, Fruit: {fruit}")
# Output:
# Index: 0, Fruit: apple
# Index: 1, Fruit: banana
# Index: 2, Fruit: orange
3. Lambda Functions
Lambda functions are anonymous functions that can be defined in a single line of code. They are used when you need a simple function that is not required to be named.
Example:
square = lambda x: x**2
print(square(5)) # Output: 25
Conclusion
Python, with its hidden secrets and mind-blowing tricks, has proven to be a game-changer in the world of programming. The magic methods, list comprehension, context managers, and decorators provide powerful tools to simplify complex coding tasks. Additionally, the unpacking technique, enumerate function, and lambda functions enhance efficiency and readability in Python programming. By harnessing the full potential of Python, developers can create elegant and concise code that is both efficient and maintainable.
FAQs
Q1: Are magic methods limited to classes in Python?
A1: Yes, magic methods are specific to classes in Python. They allow classes to define custom behaviors for built-in operators, such as addition, subtraction, and comparison, among others.
Q2: Can list comprehension be used for nested iterations?
A2: Yes, list comprehension can handle nested iterations by using multiple for loops. IT provides an elegant way to generate lists by combining elements from multiple iterables.
Q3: How can I create my own context manager in Python?
A3: You can create a context manager by implementing the __enter__()
and __exit__()
methods in a class. The __enter__()
method is called when entering the context, and the __exit__()
method is called when exiting the context.
Q4: Are decorators only used for functions?
A4: No, decorators can be applied to both functions and classes. When applied to a class, decorators modify its behavior or attributes.
Q5: Can lambda functions handle multiple arguments?
A5: Yes, lambda functions are flexible and can handle multiple arguments. They can be used as any other function by passing multiple arguments separated by commas.