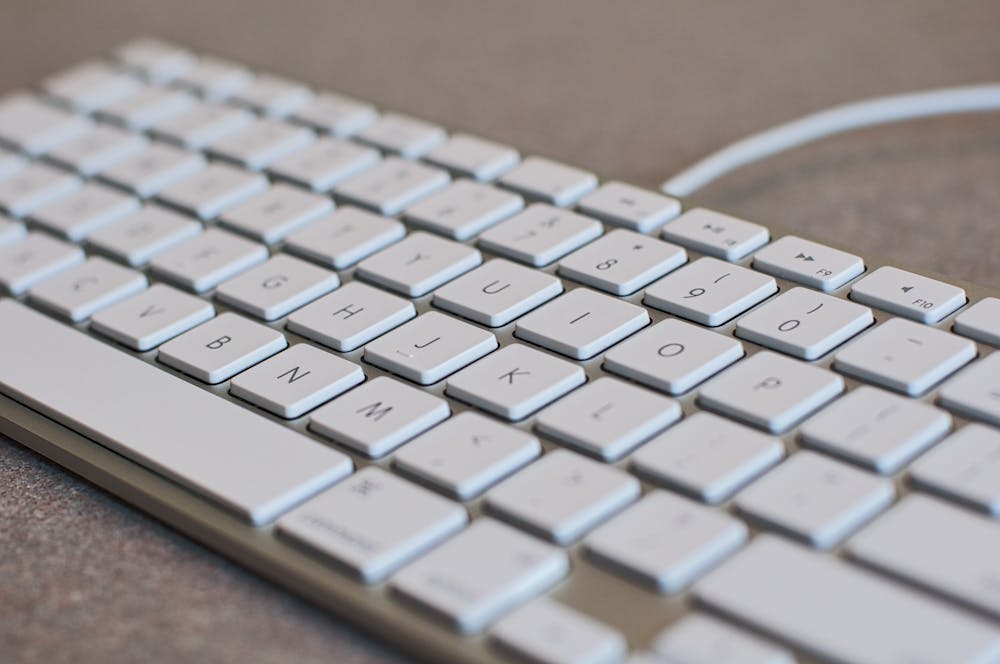
In the world of web development, Laravel has emerged as a powerful and popular PHP framework. IT provides a solid foundation for building web applications with its elegant syntax, modular architecture, and robust features. One of the key components of Laravel is the repository pattern, which plays a vital role in organizing and managing data access in an application. In this article, we’ll explore the power of Laravel repository and what you need to know to make the most of it.
Understanding the Repository Pattern
The repository pattern is a design pattern that is commonly used in web development to abstract the data access logic from the rest of the application. It acts as a middleman between the application and the data source, providing a clean and consistent interface for accessing and manipulating data. In the context of Laravel, the repository pattern is used to centralize data access logic, making it easier to maintain and test the application.
A Laravel repository typically consists of two main components: the interface and the implementation. The interface defines the contract for data access operations, such as retrieving, creating, updating, and deleting data. The implementation, on the other hand, contains the actual logic for performing these operations, usually in the form of Eloquent queries or raw SQL statements.
The Benefits of Using Laravel Repository
There are several benefits to using the repository pattern in Laravel. One of the key advantages is that it promotes separation of concerns, which is a fundamental principle of software design. By isolating the data access logic in a repository, you can ensure that it remains independent of the rest of the application, making it easier to maintain, test, and reuse.
Another benefit of using Laravel repository is that it enables you to switch between different data sources without affecting the rest of the application. For example, if you decide to change from using a MySQL database to a MongoDB database, you can simply update the repository implementation to work with the new data source, without having to make changes to the rest of the application.
Additionally, using repositories can help improve the performance of your Laravel application. By centralizing data access logic, you can optimize and reuse queries, which can lead to faster and more efficient data retrieval. This can be particularly beneficial in applications that handle large volumes of data or have complex data access requirements.
Best Practices for Using Laravel Repository
While the repository pattern can offer numerous benefits, it’s important to follow best practices to ensure that you’re using it effectively. Here are some tips for getting the most out of Laravel repository:
- Define clear and consistent interfaces for your repositories. This will make it easier for other developers to understand and use them.
- Separate your repository interfaces and implementations to keep your codebase clean and maintainable.
- Use dependency injection to inject repository instances into your application’s services and controllers. This will make it easy to swap out different repository implementations, such as for testing or using different data sources.
- Consider using a repository generator, such as the one offered by backlink works, to quickly create repository classes for your Laravel application.
Examples of Using Laravel Repository
To illustrate the power of Laravel repository, let’s take a look at a simple example. Suppose we have a blog application with a Post model that represents blog posts. We can create a repository for managing posts, with methods for retrieving, creating, updating, and deleting posts.
First, we define a repository interface for the Post model:
“`php
namespace App\Repositories;
interface PostRepository
{
public function getAllPosts();
public function createPost(array $data);
public function updatePost($id, array $data);
public function deletePost($id);
}
“`
Next, we implement the interface in a class called EloquentPostRepository, which uses Eloquent queries to interact with the database:
“`php
namespace App\Repositories;
use App\Models\Post;
class EloquentPostRepository implements PostRepository
{
public function getAllPosts()
{
return Post::all();
}
public function createPost(array $data)
{
return Post::create($data);
}
public function updatePost($id, array $data)
{
$post = Post::findOrFail($id);
$post->update($data);
return $post;
}
public function deletePost($id)
{
Post::findOrFail($id)->delete();
}
}
“`
Finally, we can use the PostRepository in our application’s services or controllers to interact with the Post model:
“`php
public function index(PostRepository $postRepository)
{
$posts = $postRepository->getAllPosts();
return view(‘posts.index’, [‘posts’ => $posts]);
}
“`
In this example, the repository pattern allows us to abstract the data access logic for the Post model, making it easier to manage and reuse throughout the application.
Conclusion
Laravel repository is a powerful tool for organizing and managing data access in your applications. By using the repository pattern, you can promote separation of concerns, improve maintainability, and enhance performance. By following best practices and leveraging examples, you can unleash the full power of Laravel repository in your projects.
FAQs
What is Laravel repository?
Laravel repository is a design pattern that is used to abstract the data access logic from the rest of the application. It consists of an interface that defines the contract for data access operations, and an implementation that contains the actual logic for performing these operations.
What are the benefits of using Laravel repository?
Some of the benefits of using Laravel repository include promoting separation of concerns, enabling easy switching between different data sources, and improving application performance.
What are some best practices for using Laravel repository?
Some best practices for using Laravel repository include defining clear and consistent interfaces, separating interfaces and implementations, using dependency injection, and considering using a repository generator for quick creation of repository classes.
Can you provide an example of using Laravel repository?
Sure! In the article, we provided an example of a simple blog application with a Post model and a corresponding repository for managing posts. The example demonstrates how the repository pattern can be used to abstract data access logic and make it more maintainable and reusable.