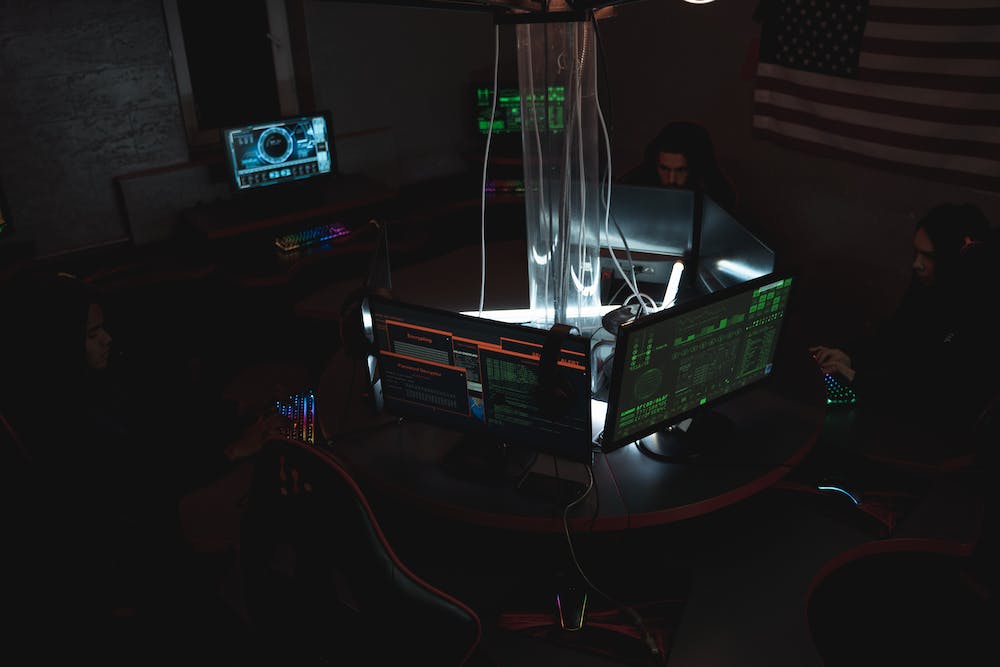
AJAX (Asynchronous JavaScript and XML) is a powerful technology that allows web developers to build dynamic web applications by sending and receiving data asynchronously between the browser and the server. Laravel, a popular PHP framework, provides excellent support for AJAX, making IT easy to implement interactive features in web applications.
Why AJAX is Important?
Traditionally, web applications used to refresh the entire page whenever data needed to be updated, resulting in a poor user experience. AJAX came to the rescue by enabling developers to update specific parts of a web page asynchronously without reloading the entire page. This significantly improved the perceived performance and responsiveness of web applications.
AJAX allows data to be fetched, processed, and displayed in the background, without disrupting the user’s ongoing interaction with the page. IT eliminates the need for full page reloads, providing a seamless and interactive user experience.
AJAX in Laravel
Laravel, being a modern and feature-rich PHP framework, provides excellent support for AJAX. IT integrates seamlessly with JavaScript frameworks like jQuery, making IT easy to handle AJAX requests and responses.
In Laravel, you can use AJAX to enhance various aspects of your web application, including form submissions, real-time notifications, dynamic content loading, and much more.
Form Submissions
With AJAX, you can submit forms without refreshing the page. This allows for immediate validation feedback and reduces the perceived loading time for the user. Laravel’s AJAX support makes IT straightforward to handle form submissions and validation.
For example, let’s say you have a registration form on your Website. Instead of submitting the form normally, you can use AJAX to send the form data to the server and receive the response. If there are any validation errors, they can be displayed without reloading the page.
$.ajax({
method: 'POST',
url: '/register',
data: $('#registration-form').serialize(),
success: function(response) {
// Handle the server response
},
error: function(error) {
// Handle the error
}
});
Real-Time Notifications
AJAX is perfect for implementing real-time notifications in your Laravel application. Whether you want to show new messages, notifications, or any other type of real-time updates, AJAX can help you achieve this seamlessly.
Laravel provides support for websockets through packages like Laravel Echo, making IT even easier to implement real-time features. With websockets, you can establish a persistent connection between the server and the client, allowing for instant updates without the need for manual polling.
Dynamic content Loading
Another powerful use case of AJAX in Laravel is dynamic content loading. You can fetch data from the server asynchronously and update the page’s content without reloading IT entirely.
For instance, imagine you have a blog with pagination. Instead of navigating to a new page when the user clicks on a pagination link, you can use AJAX to fetch the next set of blog posts and update the content dynamically. This provides a smooth user experience and eliminates unnecessary page reloads.
$('.pagination-link').click(function(e) {
e.preventDefault();
var page = $(this).attr('data-page');
$.ajax({
method: 'GET',
url: '/blogs?page=' + page,
success: function(response) {
// Update the content with the new blog posts
},
error: function(error) {
// Handle the error
}
});
});
Conclusion
AJAX in Laravel empowers web developers to create highly interactive and responsive web applications. With its support for AJAX, Laravel simplifies the implementation of various dynamic features, including form submissions, real-time notifications, and dynamic content loading.
By leveraging the power of AJAX in Laravel, web developers can enhance the overall user experience, improve performance, and create web applications that feel seamless and interactive.
FAQs
Q: Can AJAX be used with other PHP frameworks?
A: Yes, AJAX can be used with any PHP framework or even with plain PHP. However, Laravel provides a great set of tools and features that make working with AJAX more streamlined and efficient.
Q: What is the main advantage of using AJAX in web development?
A: The main advantage of using AJAX is the ability to update specific parts of a web page without reloading the entire page. This provides a better user experience by reducing perceived loading time and allowing for more interactive features.
Q: Can AJAX requests be secured in Laravel?
A: Yes, Laravel provides various mechanisms for securing AJAX requests, such as using CSRF (Cross-Site Request Forgery) tokens. These tokens ensure that AJAX requests originate from trusted sources, preventing malicious attacks.
Q: Are there any limitations to using AJAX in Laravel?
A: There are no specific limitations to using AJAX in Laravel. However, IT‘s important to ensure proper error handling and validation in your AJAX requests to handle any potential server-side issues.
Q: Can AJAX be used for file uploads in Laravel?
A: Yes, AJAX can be used for file uploads in Laravel. You can use libraries like Dropzone.js or create your own implementation to handle file uploads asynchronously.