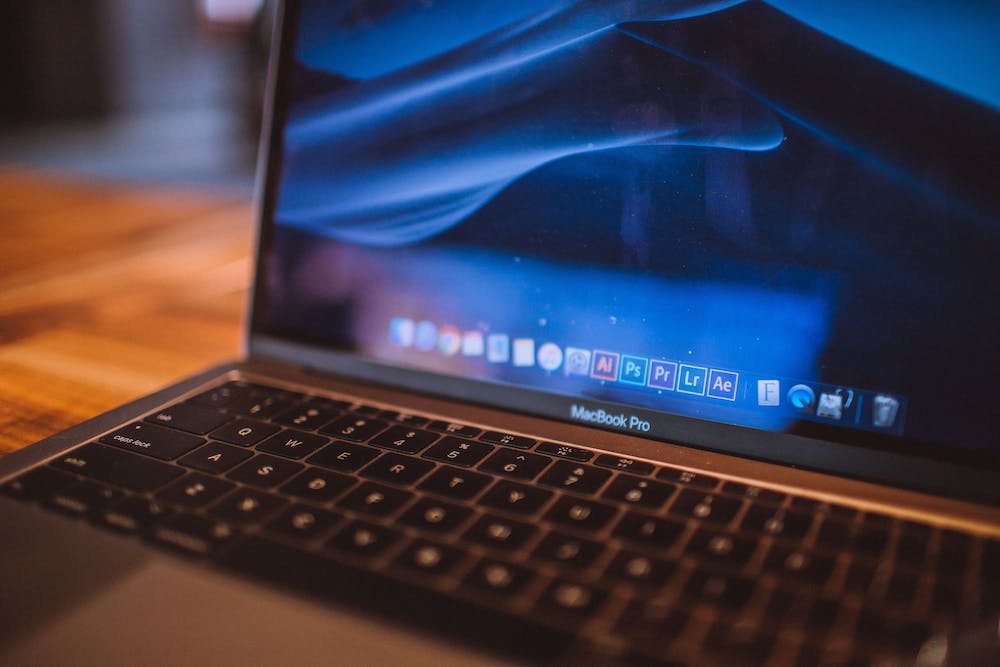
PHP7.4 is the latest release of the popular programming language PHP. IT brings a plethora of new features and improvements, making IT a powerful tool for web development. In this article, we will explore some of the hidden powers of PHP7.4 that will leave you amazed.
1. Typed Properties
One of the most exciting features introduced in PHP7.4 is the ability to declare typed properties in classes. This allows you to specify the data type for each property, ensuring type safety. Let’s consider an example:
“`php
class Person {
public string $name;
public int $age;
public function __construct(string $name, int $age) {
$this->name = $name;
$this->age = $age;
}
}
$person = new Person(“John Doe”, 30);
echo “Name: ” . $person->name . “
“;
echo “Age: ” . $person->age;
“`
In the above example, we have declared two properties, `$name` of type string and `$age` of type int. This ensures that only values of the specified types can be assigned to these properties.
2. Arrow Functions
Arrow functions, also known as short closures, have been introduced in PHP7.4. They provide a concise syntax for writing anonymous functions. Here’s an example:
“`php
$numbers = [1, 2, 3, 4, 5];
$squared = array_map(fn($number) => $number ** 2, $numbers);
print_r($squared);
“`
The above code uses an arrow function to square each number in the `$numbers` array using `array_map` function.
3. Null Coalescing Assignment Operator
PHP7.4 introduces the null coalescing assignment operator (`??=`) which provides a shorthand way of assigning a value to a variable only if IT is currently null. Here’s an example:
“`php
$name = null;
$name ??= “John Doe”;
echo $name; // Output: John Doe
“`
In the above code, the value of `$name` is only assigned as “John Doe” if IT is currently null.
4. Preloading
Preloading is a new feature in PHP7.4 that allows you to load PHP code into memory during startup, resulting in faster execution and improved performance. To enable preloading, you need to create a script that contains the code you want to preload and configure PHP accordingly. This can be a great optimization technique for large applications.
5. Foreign Function Interface (FFI)
PHP7.4 introduces the FFI extension, which allows you to call functions and access variables from C libraries directly within PHP. This opens up endless possibilities for leveraging existing libraries and building powerful applications.
Conclusion
PHP7.4 brings an array of exciting features and improvements that take PHP to the next level. From typed properties to arrow functions and preloading, developers now have powerful tools to enhance their PHP applications. The introduction of the FFI extension also opens up new possibilities for integrating existing C libraries. Embracing these features will undoubtedly lead to more efficient and performant code. Prepare to be amazed by the hidden powers of PHP7.4!
FAQs
Q: Can I use the new features of PHP7.4 with older versions of PHP?
A: No, the new features introduced in PHP7.4 are only available in PHP7.4 and later versions. You need to upgrade your PHP installation to leverage these features.
Q: Are there any backward compatibility issues when upgrading to PHP7.4?
A: Upgrading to PHP7.4 should generally be smooth without any major backward compatibility issues. However, IT is always recommended to test your codebase thoroughly before upgrading to a new version of PHP to ensure compatibility.
Q: What are the performance benefits of preloading in PHP7.4?
A: Preloading allows PHP code to be loaded into memory during startup, eliminating the need for repeated file loading and parsing. This can significantly improve performance by reducing the time spent on file operations.
Q: Can I use arrow functions instead of regular functions in all scenarios?
A: While arrow functions offer a more concise syntax, they have some limitations. For example, they do not have access to `$this` and cannot be used as methods within classes. IT is important to understand these limitations and use arrow functions appropriately.