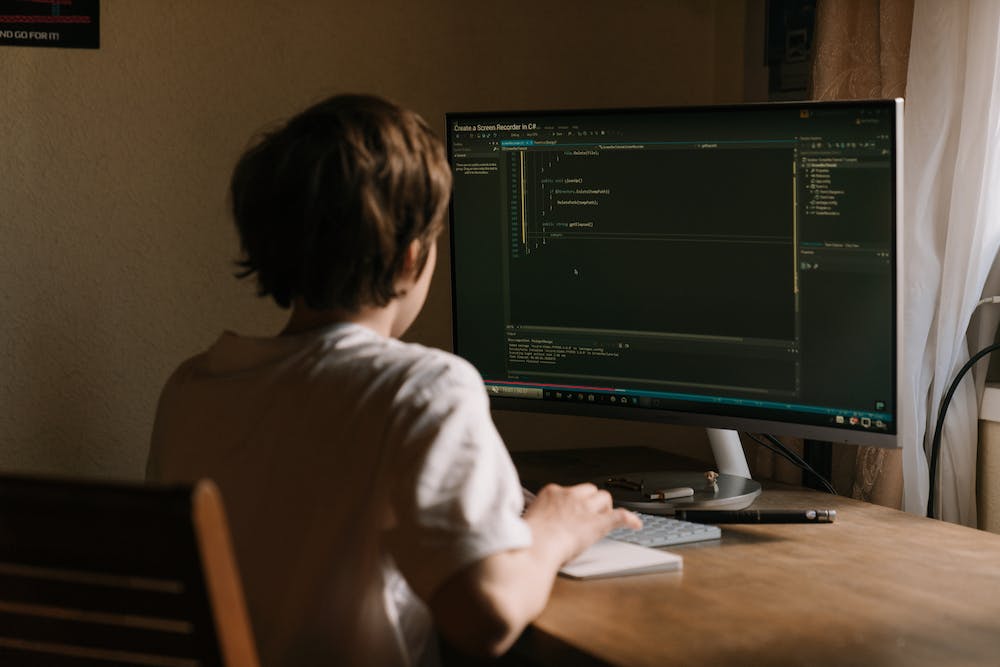
JavaScript is a powerful programming language that has become an integral part of web development. Traditionally, JavaScript has been used for adding interactivity and enhancing user experience on websites. However, with the advent of functional programming, JavaScript has evolved to become even more powerful and versatile. In this article, we will explore the hidden powers of JavaScript through functional programming and see how IT can amaze us with its capabilities.
Understanding Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions. Unlike imperative programming, which uses statements to change the state of a program, functional programming focuses on using pure functions that do not modify state and produce the same output for the same inputs.
JavaScript has always supported functional programming to some extent, but recent advancements in the language have made IT even more functional. The introduction of arrow functions, higher-order functions, and built-in functional methods like map, filter, and reduce have made IT easier to write functional code in JavaScript.
The Power of Pure Functions
One of the core principles of functional programming is the use of pure functions. A pure function is a function that, given the same inputs, always produces the same output and has no side effects. This immutability makes pure functions easy to reason about and test, as they do not rely on external state and only depend on their inputs.
Let’s take an example to understand the power of pure functions. Consider a simple function that calculates the square of a number:
function square(x) {
return x * x;
}
The above function is a pure function because IT always produces the same output for the same input and does not modify any external state. This allows us to use the function confidently in our code, knowing that IT will not introduce any unexpected behavior.
Working with Higher-Order Functions
In functional programming, functions are treated as first-class citizens, meaning they can be assigned to variables, passed as arguments to other functions, and returned as values from other functions. This opens up a whole new world of possibilities and allows us to write more reusable and composable code.
Higher-order functions are functions that operate on other functions. They can take a function as an argument, return a function, or do both. Higher-order functions enable us to abstract common patterns and make our code more concise and expressive.
Let’s consider an example where we have a list of numbers and want to calculate the sum of their squares. We can achieve this using the higher-order function reduce
:
const numbers = [1, 2, 3, 4, 5];
const sumOfSquares = numbers.reduce((sum, num) => sum + square(num), 0);
console.log(sumOfSquares); // Output: 55
In the above code, we pass a callback function to the reduce
method. This callback function takes two arguments: the accumulated sum and the current value. We use the square
function to calculate the square of each number and add IT to the sum. Finally, we get the desired output, which is the sum of the squares.
Using Immutable Data Structures
Functional programming favors immutability, which means that once a value is assigned, IT cannot be changed. In JavaScript, objects and arrays are mutable by default. However, we can use libraries like Immutable.js or Immer.js to work with immutable data structures.
Immutable data structures ensure that once a value is created, IT remains unchanged. This avoids accidental mutations and makes our code more predictable and easier to reason about. IT also simplifies debugging and allows for efficient change detection, which can be beneficial for performance optimization.
Let’s see an example using Immutable.js:
const { List } = require('immutable');
const originalList = List([1, 2, 3]);
const modifiedList = originalList.push(4);
console.log(originalList.toJS()); // Output: [1, 2, 3]
console.log(modifiedList.toJS()); // Output: [1, 2, 3, 4]
In the above code, we create an immutable list using the List
constructor from Immutable.js. We then use the push
method to add a new element to the list. IT‘s important to note that the push
method does not modify the original list but returns a new list with the added element. This preserves the immutability of the original list.
Conclusion
In conclusion, functional programming has unlocked the hidden powers of JavaScript, making IT an even more formidable language for web development. By embracing pure functions, higher-order functions, and immutable data structures, we can write more reliable, scalable, and maintainable code. Functional programming allows us to harness the full potential of JavaScript and take our programming skills to the next level.
FAQs
Q: What are the benefits of functional programming in JavaScript?
A: Functional programming in JavaScript offers several benefits, including improved code maintainability, enhanced code reusability, reduced side effects, and increased code testability. IT allows for easier reasoning about code, as functions are pure and do not rely on external state.
Q: Are functional programming and object-oriented programming mutually exclusive?
A: No, functional programming and object-oriented programming are not mutually exclusive. In fact, they can complement each other in many cases. JavaScript, being a multi-paradigm language, allows developers to use both functional and object-oriented programming techniques as per their requirements.
Q: Can functional programming be used in all JavaScript projects?
A: Functional programming can be used in most JavaScript projects. However, the decision to adopt functional programming depends on various factors such as project requirements, team expertise, and specific use cases. IT is important to assess the feasibility and benefits of functional programming in each project before making a decision.
Q: Are there any performance considerations when using functional programming in JavaScript?
A: While functional programming can lead to cleaner and more maintainable code, IT is important to consider performance implications. Functions like map
, filter
, and reduce
can have overhead due to their additional processing. However, modern JavaScript engines are optimized to handle functional programming constructs efficiently, and the overall performance impact is usually negligible for most applications.