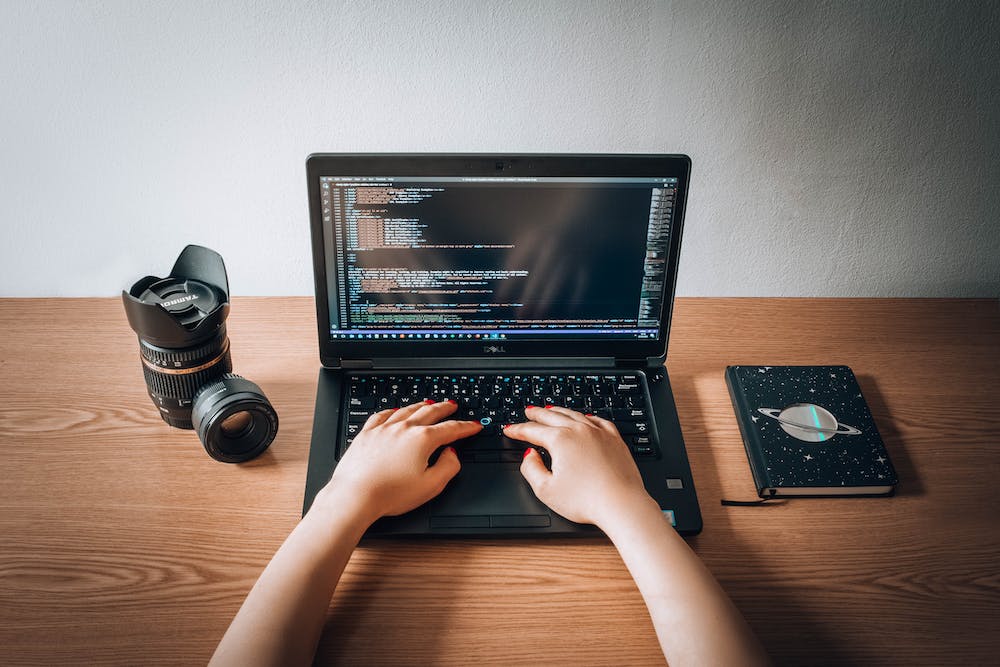
In the domain of computer science and mathematics, the Fibonacci sequence is a well-known and intriguing mathematical pattern. Its simplicity and elegance have captivated the minds of programmers, mathematicians, and enthusiasts alike.
In this article, we will dive into the hidden secrets of the Fibonacci sequence and explore how to unlock the full potential of Python to generate mind-blowing code for Fibonacci computations. So, let’s embark on this exciting journey!
The Fibonacci Sequence: A Quick Introduction
The Fibonacci sequence is a series of numbers in which each number is the sum of the two preceding ones. IT starts with 0 and 1:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, ...
The sequence is named after Leonardo of Pisa, also known as Fibonacci. He introduced this sequence to the Western world in his book “Liber Abaci” published in 1202, where he used IT to solve a rabbit breeding problem.
Fibonacci Computation using Python
Python’s simplicity and versatility make IT an ideal tool for computing the Fibonacci sequence. Let’s explore some powerful techniques to generate mind-blowing code:
1. Recursive Approach
The recursive approach is a natural way to compute Fibonacci numbers, as IT directly follows the definition of the sequence. We can define a function that calls itself to calculate the Fibonacci number for a given position:
def fibonacci_recursive(n):
if n <= 1:
return n
else:
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
Although elegant, the recursive approach suffers from performance issues for large values of n, as IT recalculates the same Fibonacci numbers multiple times.
2. Memoization
Memoization is a technique used to optimize recursive algorithms by caching the results of expensive function calls. Python provides built-in support for memoization using decorators:
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci_memoization(n):
if n <= 1:
return n
else:
return fibonacci_memoization(n-1) + fibonacci_memoization(n-2)
By applying memoization, we can significantly improve the performance of the recursive approach, as previously computed Fibonacci numbers are stored for future use.
3. Iterative Approach
If we are primarily concerned about performance, an iterative approach is often the best choice. IT avoids the overhead of function calls and unnecessary recalculations:
def fibonacci_iterative(n):
if n <= 1:
return n
else:
a, b = 0, 1
for _ in range(n-1):
a, b = b, a + b
return b
The iterative approach is more efficient than the recursive approach, as IT calculates Fibonacci numbers in a loop, storing only the necessary previous values.
4. Matrix Exponentiation
If we are dealing with very large Fibonacci numbers, we can exploit matrix exponentiation to speed up computations. This approach leverages the fact that raising a matrix to a power can be done efficiently:
import numpy as np
def fibonacci_matrix(n):
matrix = np.array([[1, 1], [1, 0]], dtype=object)
result = np.array([[1, 0], [0, 1]], dtype=object)
while n > 0:
if n % 2 == 1:
result = np.matmul(result, matrix)
matrix = np.matmul(matrix, matrix)
n //= 2
return result[0, 1]
By using matrix exponentiation, we can compute extremely large Fibonacci numbers efficiently by raising the Fibonacci matrix to the power of n.
Conclusion
The Fibonacci sequence is not only a fascinating mathematical concept but also a playground for Python programmers to demonstrate their coding skills. In this article, we explored various approaches to compute Fibonacci numbers using Python. From the simple recursive approach to the optimized matrix exponentiation technique, each method has its own advantages and applications.
Whether you seek elegance or performance, Python provides the tools to tackle Fibonacci computations effectively. So, embrace the hidden Fibonacci secrets and unlock the full power of Python!
FAQs
Q1: What are the practical applications of the Fibonacci sequence?
A1: The Fibonacci sequence has found its applications in various fields, including mathematics, computer science, finance, art, and nature. IT can be used to model growth patterns, analyze financial markets, create aesthetically pleasing designs, and much more.
Q2: Can the Fibonacci sequence be negative?
A2: Although the standard Fibonacci sequence starts with 0 and 1, IT is possible to generalize the sequence to negative numbers by considering negative indices. However, this extension is not as commonly discussed as the original sequence.
Q3: Are there any other mathematical sequences related to Fibonacci?
A3: Yes, there are several related sequences built on the Fibonacci sequence. Some examples include the Lucas sequence, Pell sequence, and Fibonacci cubes, which add their own unique twists to the original pattern.
Q4: Are there any faster algorithms for computing Fibonacci numbers?
A4: While the approaches discussed in this article already offer significant optimizations, ongoing research continues to explore even faster algorithms. Some notable examples include using golden ratio formulas, exploiting number-theoretic properties, and advanced mathematical techniques.