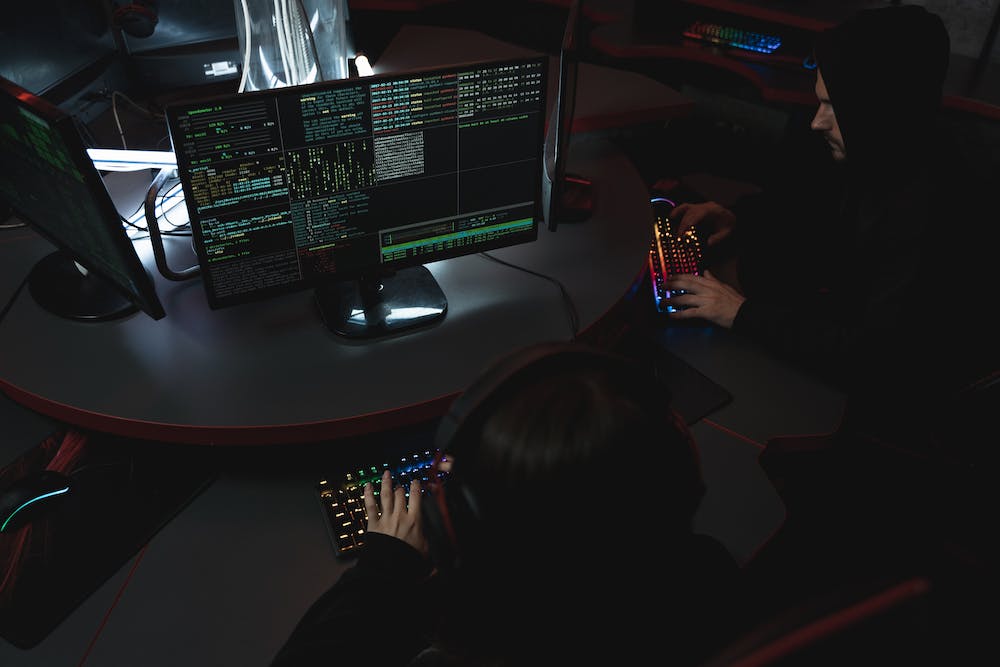
Chess is a game that has stood the test of time, captivating players of all ages and skill levels. IT‘s a game of strategy, intellect, and patience. And what better way to hone your chess skills than by coding your own chess game using Python?
In this article, we’ll guide you through the process of creating a simple yet functional Python chess game in just 5 minutes. Whether you’re a beginner or an experienced programmer, this project will give you a taste of the power of Python and its ability to bring ideas to life.
Getting Started
Before we dive into the coding part, let’s make sure you have Python installed on your machine. If not, head over to the official Python Website and download the latest version of Python. Once you have Python installed, you’re ready to start coding your chess game.
Setting Up the Board
The first step in creating your Python chess game is setting up the board. We’ll use a 2D list to represent the chess board, with each element representing a square on the board. Here’s a simple code snippet to define the initial state of the board:
board = [
["R", "N", "B", "Q", "K", "B", "N", "R"],
["P", "P", "P", "P", "P", "P", "P", "P"],
[" ", " ", " ", " ", " ", " ", " ", " "],
[" ", " ", " ", " ", " ", " ", " ", " "],
[" ", " ", " ", " ", " ", " ", " ", " "],
[" ", " ", " ", " ", " ", " ", " ", " "],
["p", "p", "p", "p", "p", "p", "p", "p"],
["r", "n", "b", "q", "k", "b", "n", "r"]
]
Here, “R” represents the rook, “N” represents the knight, “B” represents the bishop, “Q” represents the queen, and “K” represents the king. “P” represents the pawn, and lowercase letters represent the black pieces. Empty squares are denoted by a space character.
Displaying the Board
Now that we have our board set up, let’s write a function to display the board on the console. This function will take the 2D list representing the board as input and print it in a visually appealing format:
def print_board(board):
for i in range(8):
for j in range(8):
print(board[i][j], end=" ")
print()
Call the print_board function and pass the board list to it. You’ll see the initial state of the chess board displayed on the console.
Handling User Input
The next step is to allow the user to make moves on the chess board. We’ll write a simple function to take user input in the format “from_square to to_square” and update the board accordingly:
def make_move(board, from_square, to_square):
# Code to update the board based on user input
You can implement the make_move function to handle user input and update the board accordingly. This function will become the core of your chess game, allowing players to make moves and progress through the game.
Checkmate and Game Over
Finally, you’ll want to implement a mechanism to check for checkmate and end the game when one player’s king is captured. You can write a function to check for the king’s status and declare the game over when a checkmate is detected:
def is_checkmate(board):
# Code to check for checkmate
Implement the is_checkmate function to check for the king’s status and end the game when necessary. This will give your chess game a sense of completion and add a layer of strategy to the gameplay.
Conclusion
There you have it! In just a few simple steps, you’ve created a functional Python chess game. This project is a great way to improve your Python skills while having fun and challenging yourself. Whether you’re a chess enthusiast or a programming novice, this project is a fantastic way to unleash your inner grandmaster and dive into the world of Python programming.
Keep experimenting with your chess game to add features like castling, en passant captures, and pawn promotion. You can also create a simple graphical user interface using libraries like Tkinter to enhance the user experience. The possibilities are endless, so don’t be afraid to get creative and make the game your own.
FAQs
1. Is Python a good language for game development?
Python is a versatile language that can be used for a wide range of applications, including game development. While it may not be as performance-optimized as languages like C++ or Java, Python’s simplicity and ease of use make it a great choice for beginners and hobbyist game developers.
2. Can I use this chess game as a starting point for more advanced projects?
Absolutely! This simple chess game is an excellent starting point for more complex game development projects. You can expand on the game logic, add graphical elements, implement AI opponents, and much more. Use this project as a stepping stone to explore the world of game development and expand your programming skills.
3. Are there any resources to learn more about Python game development?
There are plenty of resources available online to help you further your Python game development skills. Websites, forums, and online courses offer tutorials, articles, and community support to guide you through the process of creating games with Python. backlink works is a great resource for finding articles and tutorials on Python game development.