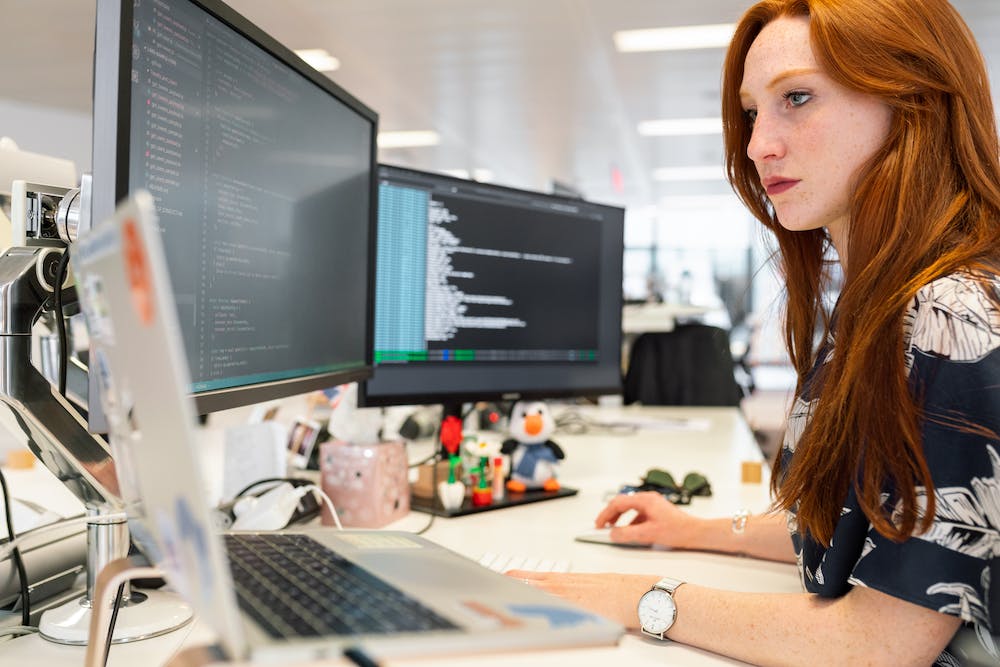
Introduction
Hangman is a classic word-guessing game that has captured the hearts and minds of people for generations. IT is not only a fun pastime but also a great exercise to enhance your vocabulary and problem-solving skills. In this article, we will guide you through the process of building your own Hangman game using Python programming language. Get ready to unleash your inner codebreaker and create a game that will keep players hooked for hours!
What is Hangman?
Hangman is a word-guessing game in which one player thinks of a word and the other player(s) try to guess IT by suggesting letters. The word to be guessed is represented by a sequence of dashes, each representing a letter. The guessing player(s) must try to guess the word by suggesting one letter at a time. If the suggested letter is present in the word, IT is revealed at the corresponding positions. If the suggested letter is not in the word, a part of the hangman’s figure is drawn. The player(s) win if they guess all the letters of the word before the entire hangman figure is drawn, otherwise they lose.
Building the Hangman Game
To create our Hangman game, we will be using Python programming language. Python provides a simple syntax and a wide range of libraries that make IT an ideal choice for game development. Here’s a step-by-step guide on how to build the game:
Step 1: Import necessary libraries
We need to import the random library to randomly select a word from a predefined list of words. We will also import the string library to check for valid input.
import random
import string
Step 2: Define the word list
Create a list of words from which the game will randomly select a word to be guessed. You can use any words of your choice, or you can find word lists available online.
word_list = ["apple", "banana", "carrot", "dragonfruit", "eggplant", "fig", "grape", "honeydew"]
Step 3: Initialize game variables
We need to initialize variables to keep track of the word to be guessed, the letters guessed so far, and the remaining attempts.
word = random.choice(word_list)
letters_guessed = []
attempts_remaining = 6
Step 4: Define the main game loop
The game should continue until the word is guessed correctly or the remaining attempts reach zero. Inside the loop, we will prompt the player for a letter and check if IT is present in the word. We will also keep track of the letters guessed so far and update the remaining attempts accordingly.
while True:
print("Word: ", end="")
for letter in word:
if letter in letters_guessed:
print(letter, end=" ")
else:
print("_", end=" ")
print("\nAttempts remaining:", attempts_remaining)
if attempts_remaining == 0:
print("You lose! The word was", word)
break
if set(word) == set(letters_guessed):
print("Congratulations! You guessed the word", word)
break
guess = input("Enter a letter: ").lower()
if len(guess) != 1 or guess not in string.ascii_lowercase:
print("Invalid input! Please enter a single lowercase letter.")
continue
if guess in letters_guessed:
print("You already guessed that letter!")
continue
letters_guessed.append(guess)
if guess not in word:
attempts_remaining -= 1
print("Wrong guess!")
Conclusion
Congratulations on creating your own Hangman game using Python! By following the step-by-step guide, you have learned how to build a fun and addictive game that can entertain players for hours. Feel free to explore further and enhance the game by adding more features such as graphics, difficulty levels, or even multiplayer support. Happy coding and enjoy unleashing your inner codebreaker!
FAQs
Q: Can I add my own word list to the game?
A: Absolutely! You can modify the “word_list” variable and add your own words to the list. Make sure to enclose each word in quotes and separate them with commas.
Q: How can I change the number of attempts allowed?
A: In the “Initialize game variables” section, you can modify the value of “attempts_remaining” to change the number of attempts allowed for guessing the word.
Q: Is there a way to make the game case-insensitive?
A: Yes, by converting both the word and the player’s guess to lowercase, you can make the game case-insensitive. Simply modify the code in the “main game loop” section to convert the input and letters to lowercase.
Q: Can I add graphics to the game?
A: Yes, you can enhance the game by adding graphics using Python libraries such as Pygame or Tkinter. These libraries provide functions to create interactive graphical interfaces for your game.