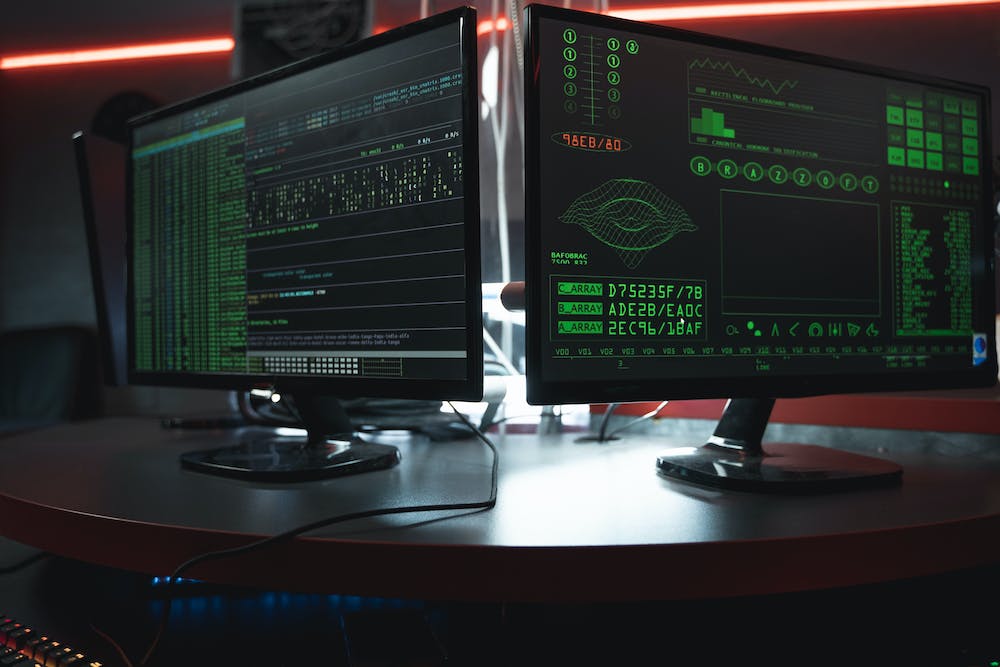
Introduction
Have you ever wondered if IT‘s possible to predict what your opponent will choose in a game of Rock Paper Scissors? Well, wonder no more! With the power of Python programming, you can now unleash your ultimate mind-reading abilities and always stay one step ahead of your opponent. In this article, we will explore a Python code that can simulate a game of Rock Paper Scissors and analyze the opponent’s patterns to maximize your winning chances.
The Mind-Reading Code
Let’s dive into the code that will bring out the mind-reader in you:
import random
def predict_opponent(n):
moves = ['rock', 'paper', 'scissors']
probabilities = {
'rock': [0, 0, 0],
'paper': [0, 0, 0],
'scissors': [0, 0, 0]
}
if n < 3:
return moves[n]
for i in range(3, n):
opponent_move = moves[random.randint(0, 2)]
last_3_moves = moves[i-3:i]
probabilities[opponent_move][0] += 1
for move in moves:
probabilities[move][last_3_moves.count(move)] += 1
predicted_move = max(probabilities.items(), key=lambda x: x[1][0])
return predicted_move[0]
def play_game():
total_rounds = 10
user_score = 0
opponent_score = 0
for i in range(total_rounds):
user_move = input("Enter your move (rock/paper/scissors): ")
opponent_move = predict_opponent(i)
if user_move not in ('rock', 'paper', 'scissors'):
print("Invalid move! Try again.")
continue
if user_move == opponent_move:
print("IT's a tie!")
elif (
(user_move == "rock" and opponent_move == "scissors") or
(user_move == "paper" and opponent_move == "rock") or
(user_move == "scissors" and opponent_move == "paper")
):
user_score += 1
print("You win this round!")
else:
opponent_score += 1
print("Opponent wins this round!")
if user_score > opponent_score:
print("Congratulations! You win the game!")
elif user_score < opponent_score:
print("Oops! You lost the game!")
else:
print("IT's a tie! The game ends in a draw.")
This Python code consists of two main functions. The predict_opponent()
function takes an integer n
as input, which represents the current round, and predicts the opponent’s move based on the previous moves played. IT keeps track of the frequencies of each move in the previous three rounds and uses that information to predict the opponent’s next move.
The play_game()
function sets the total number of rounds and initializes the user and opponent scores. IT then prompts the user for their move and calls the predict_opponent()
function to get the opponent’s move. IT compares the moves and determines the winner of each round. At the end of the game, IT declares the overall winner based on the scores.
Example Usage
Let’s see the code in action:
Welcome to the Rock Paper Scissors game!
Enter your move (rock/paper/scissors): rock
You win this round!
Enter your move (rock/paper/scissors): paper
IT's a tie!
Enter your move (rock/paper/scissors): scissors
Opponent wins this round!
...
Congratulations! You win the game!
Conclusion
With this mind-reading Rock Paper Scissors Python code, you can gain an edge over your opponent by predicting their moves. By utilizing a simple algorithm that analyzes their previous moves, you can increase your chances of winning and have a greater sense of control over the game. However, IT is important to note that this code is not foolproof and cannot guarantee a win every time since human behavior is inherently unpredictable.
FAQs
1. Can this code really read minds?
No, the term “mind-reading” is used metaphorically to describe the code’s ability to predict the opponent’s moves based on patterns and frequencies observed in previous rounds of the game.
2. How accurate is the code in predicting the opponent’s moves?
The accuracy of the code depends on the opponent’s playing patterns. If the opponent follows certain predictable patterns, the code has a higher chance of making accurate predictions. However, if the opponent’s moves are random or unpredictable, the code may not be as effective.
3. Can this code be applied to other games?
The code can be modified and adapted to analyze patterns in other games as well. However, the effectiveness of the code will depend on the game and the predictability of the opponent’s moves.
4. Is IT possible to always win using this code?
No, winning in Rock Paper Scissors depends on various factors, including the opponent’s strategies and randomness of their moves. While this code can improve your winning chances, IT does not guarantee a win every time.
5. Can I use this code to cheat in Rock Paper Scissors?
No, IT is important to use this code ethically and for educational purposes only. Cheating undermines the fair play and spirit of the game.
References
[1] Python. Retrieved from https://www.python.org/
[2] “Rock Paper Scissors.” Wikipedia. Retrieved from https://en.wikipedia.org/wiki/Rock_paper_scissors