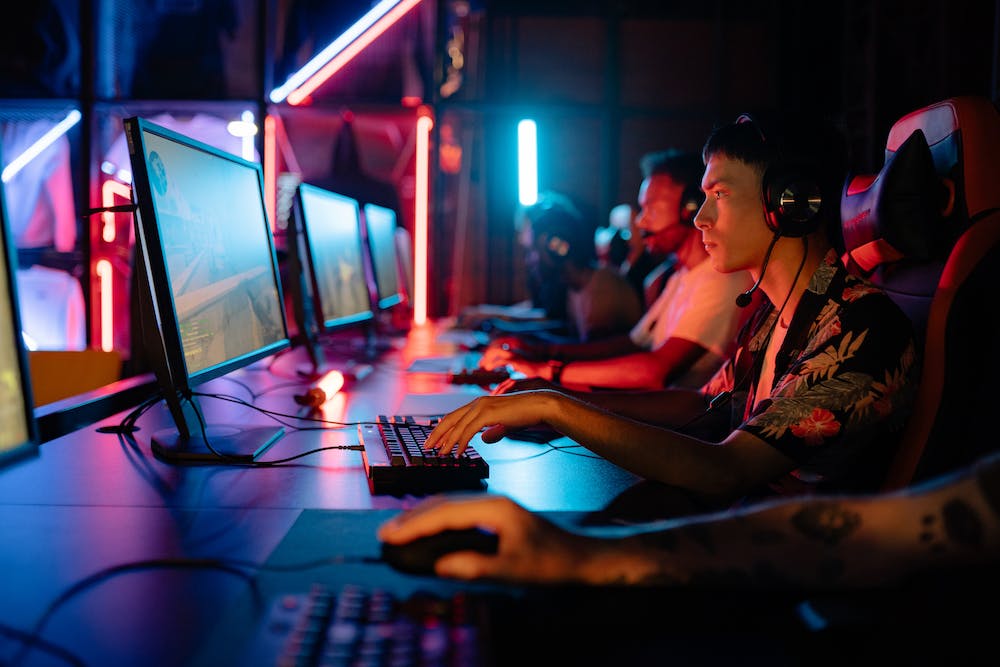
Python is a powerful programming language that has gained immense popularity in recent years. Its simplicity, readability, and versatility make IT a favorite among developers, data scientists, and machine learning enthusiasts. If you’re new to Python or looking to enhance your skills, one great way to start is by learning how to create a word count program. In this article, we’ll walk you through the process of building a word count program using Python and show you just how easy it is to use.
Getting Started with Python
If you’re new to Python, it’s essential to install Python on your computer. You can download Python from the official Website (python.org) and follow the installation instructions. Once you have Python installed, you can begin writing your first Python program.
Building the Word Count Program
Python makes it simple to build a word count program due to its straightforward syntax and powerful built-in functions. Let’s start by writing a basic word count program that counts the occurrence of each word in a given text.
# Word Count Program in Python
def word_count(text):
word_list = text.split()
word_count = {}
for word in word_list:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
# Example Usage
text = "Python is a powerful programming language. Python is easy to learn."
print(word_count(text))
In the above code, we define a function called word_count
that takes a text
parameter. Inside the function, we split the text into individual words and create a dictionary to store the word count. We then iterate through each word in the list and update the word count in the dictionary. Finally, we return the word count dictionary.
Optimizing the Word Count Program
While the basic word count program works fine, we can optimize it further by removing punctuation and converting all words to lowercase. This ensures that words like “Python” and “python” are counted as the same word.
import string
def word_count(text):
text = text.lower()
text = text.translate(str.maketrans('', '', string.punctuation)) # Remove Punctuation
word_list = text.split()
word_count = {}
for word in word_list:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
# Example Usage
text = "Python is a powerful programming language. Python is easy to learn."
print(word_count(text))
In this updated version of the word count program, we first convert the text to lowercase using the lower()
method. We then use the translate()
method to remove all punctuation from the text. This ensures that words are counted consistently, regardless of their casing or punctuation.
Adding User Input
So far, we have been using a predefined text for our word count program. However, it’s more practical to allow users to input their text. We can achieve this by using the input()
function in Python.
import string
def word_count(text):
text = text.lower()
text = text.translate(str.maketrans('', '', string.punctuation))
word_list = text.split()
word_count = {}
for word in word_list:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
# User Input
user_text = input("Enter the text: ")
print(word_count(text))
In this version, we use the input()
function to prompt the user to enter the text. The entered text is then passed to the word_count
function, and the word count is displayed.
Conclusion
Python’s simplicity and versatility make it an excellent choice for building various programs, including a word count program. In this article, we’ve demonstrated how easy it is to create a word count program in Python and how you can further optimize it for better accuracy. Whether you’re a beginner or an experienced Python developer, exploring new programs like this can help you sharpen your skills and expand your programming knowledge.
FAQs
Q: Can the word count program handle large texts?
A: Yes, the word count program can handle large texts efficiently. Python’s built-in data structures and functions make it suitable for processing large volumes of text data.
Q: Are there any libraries in Python that can help with word counting?
A: Yes, Python has libraries such as NLTK (Natural Language Toolkit) and SpaCy that offer advanced text processing capabilities, including word counting and analysis.
Q: Is Python the best choice for building a word count program?
A: Python is an excellent choice for building a word count program due to its simplicity, readability, and extensive libraries for text processing and analysis.