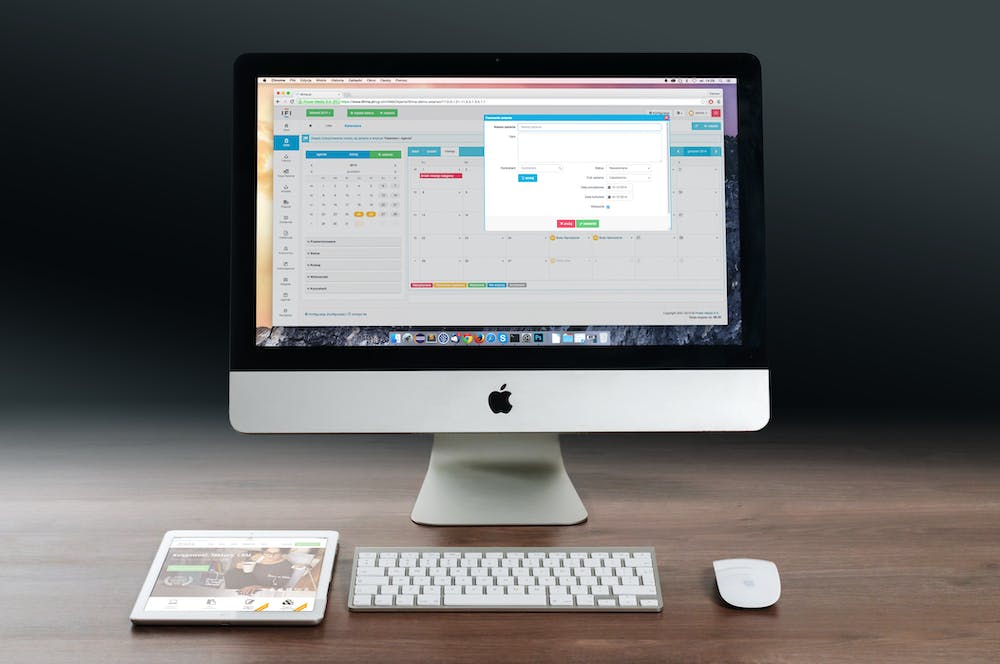
Python is a versatile and powerful programming language that is widely used in various fields, including web development, data analysis, artificial intelligence, and more. Its simplicity and ease of use make IT an ideal choice for beginners and experienced programmers alike. In this article, we will explore the incredible potential of Python and how IT can be harnessed to create a mind-blowing binary search program.
So, what exactly is a binary search program? In computer science, a binary search is an efficient algorithm used to locate a specific element in a sorted array or list. IT works by repeatedly dividing the search space in half until the target element is found. This algorithm is incredibly fast, with a time complexity of O(log n), making IT a valuable tool in many applications.
Python’s simplicity and readability make IT the perfect language to implement a binary search program. Let’s take a closer look at how you can revolutionize your coding skills by leveraging the power of Python.
Step 1: Understanding the Binary Search Algorithm
Before we dive into the code, let’s briefly go over the binary search algorithm. The basic idea behind a binary search is as follows:
1. Start with the entire sorted array or list.
2. Divide the search space in half.
3. Check if the middle element is equal to the target element.
4. If IT is, the search is complete.
5. If the middle element is greater than the target element, repeat the search on the left half of the array or list.
6. If the middle element is less than the target element, repeat the search on the right half of the array or list.
7. Repeat steps 2-6 until the target element is found, or the search space is empty.
By iteratively applying these steps, a binary search can quickly find the desired element in a sorted array or list.
Step 2: Coding the Binary Search Program in Python
Now that we have a clear understanding of the binary search algorithm, let’s dive into coding IT in Python. Here’s a simple implementation of the binary search algorithm:
“`python
def binary_search(arr, target):
low = 0
high = len(arr) – 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid – 1
return -1
“`
Let’s break down the code:
– We define a function called `binary_search` that takes two parameters: `arr` (the sorted array or list) and `target` (the element we want to search for).
– We initialize two variables, `low` and `high`, to represent the lower and upper bounds of the search space.
– Inside the while loop, we calculate the middle index using the formula `(low + high) // 2`.
– We compare the middle element with the target element. If they are equal, we found the target and return its index.
– If the middle element is less than the target, we update the lower bound `low` to be the middle index + 1.
– If the middle element is greater than the target, we update the upper bound `high` to be the middle index – 1.
– We repeat these steps until the target element is found or the search space is empty.
– If the target element is not found, we return -1.
You can now use this binary search function in your Python programs to quickly find elements in sorted arrays or lists.
Conclusion
Python is a truly remarkable programming language that offers immense power and flexibility. By harnessing the simplicity and ease of use of Python, you can create mind-blowing binary search programs with ease. The binary search algorithm, with its time complexity of O(log n), is indispensable in many applications, and Python makes IT incredibly accessible.
By understanding the fundamentals of the binary search algorithm and implementing IT in Python, you can revolutionize your coding skills and unlock new possibilities in your programming journey. Whether you’re a beginner or an experienced programmer, leveraging Python for binary search programs is a guaranteed way to enhance your coding abilities.
FAQs
Q: What is a binary search?
A: A binary search is an efficient algorithm used to locate a specific element in a sorted array or list by repeatedly dividing the search space in half.
Q: Why is Python a great language for binary search programs?
A: Python’s simplicity, readability, and ease of use make IT an excellent choice for implementing binary search programs.
Q: What is the time complexity of the binary search algorithm?
A: The time complexity of the binary search algorithm is O(log n), making IT incredibly fast.
Q: Can binary search be applied to unsorted arrays or lists?
A: No, binary search requires a sorted array or list to work correctly.
Q: Are there any libraries or modules in Python that provide binary search functionality?
A: Yes, the `bisect` module in Python provides binary search functionalities for sorted lists.
References:
– Python documentation: https://docs.python.org/
– GeeksforGeeks: https://www.geeksforgeeks.org/