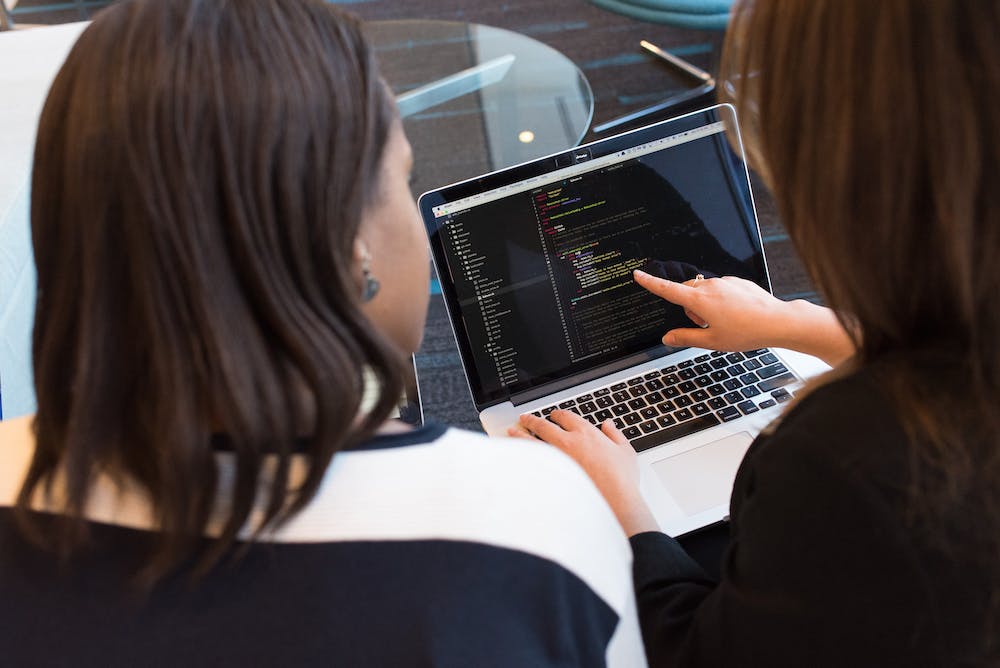
Python is a powerful and versatile programming language that is widely used in a variety of fields, including web development, data analysis, artificial intelligence, and more. One area where Python truly shines is in its ability to perform complex calculations and create custom calculator code for specific tasks. In this article, we will explore some mind-blowing calculator code tricks using Python that will showcase the true power of this language.
1. Simple Calculator Code
Let’s start with a simple calculator code that can perform basic arithmetic operations such as addition, subtraction, multiplication, and division. Using Python, we can create a user-friendly calculator that takes input from the user and performs the desired operation.
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
return "Cannot divide by zero"
else:
return x / y
In this example, we have defined four functions for performing the basic arithmetic operations. We then prompt the user to input two numbers and the desired operation, and based on the input, we call the appropriate function to perform the calculation.
2. Advanced Calculator Code
Python also allows us to create more advanced calculator code that can handle complex mathematical functions and operations. By using libraries such as math and numpy, we can integrate advanced mathematical functions into our calculator code.
import math
import numpy as np
def calculate_sin(x):
return math.sin(x)
def calculate_cos(x):
return math.cos(x)
def calculate_log(x):
return math.log(x)
def calculate_exp(x):
return math.exp(x)
def calculate_mean(numbers):
return np.mean(numbers)
def calculate_median(numbers):
return np.median(numbers)
In this example, we have integrated the math and numpy libraries to perform trigonometric, logarithmic, exponential, and statistical calculations. This advanced calculator code showcases the versatility of Python and its ability to handle complex mathematical operations with ease.
3. Custom Calculator Code
One of the most powerful features of Python is its flexibility to create custom calculator code tailored to specific needs. Whether IT‘s a financial calculator, engineering calculator, or any other specialized calculator, Python can be used to develop a customized solution.
def calculate_future_value(principal, rate, time):
return principal * (1 + rate) ** time
def calculate_mortgage_payment(principal, rate, time):
return principal * (rate * (1 + rate) ** time) / ((1 + rate) ** time - 1)
In this example, we have created custom calculator code for calculating the future value of an investment and the monthly mortgage payment. These examples demonstrate how Python can be used to create sophisticated calculator code for specific financial calculations.
4. GUI Calculator Code
Python also provides the ability to create graphical user interface (GUI) applications, which can be used to develop calculator code with a user-friendly interface. By using libraries such as Tkinter, PyQt, or Kivy, we can create GUI-based calculator applications that are intuitive and easy to use.
import tkinter as tk
def on_button_click(button):
# Perform calculation based on button clicked
pass
# Create GUI elements
# ...
# Configure layout
# ...
# Create main loop
# ...
In this example, we have outlined the basic structure of a GUI calculator code using Tkinter. With the ability to create buttons, input fields, and event handlers, we can develop a fully functional calculator application with a sleek and modern user interface.
5. Error Handling in Calculator Code
Error handling is an important aspect of calculator code to ensure that the application can gracefully handle unexpected input or invalid operations. Python provides robust error handling mechanisms such as try-except blocks and assertions, which can be used to detect and handle errors effectively.
def divide(x, y):
try:
result = x / y
except ZeroDivisionError:
result = "Cannot divide by zero"
return result
In this example, we have used a try-except block to handle the division by zero error and return a custom error message. By incorporating error handling into our calculator code, we can enhance the reliability and robustness of the application.
6. Unit Testing for Calculator Code
Unit testing is an essential practice in software development to validate the functionality of code and ensure that it performs as expected. Python offers built-in testing frameworks such as unittest and pytest, which can be utilized to create comprehensive test cases for calculator code.
import unittest
class TestCalculator(unittest.TestCase):
def test_addition(self):
self.assertEqual(add(3, 7), 10)
def test_division(self):
self.assertEqual(divide(10, 2), 5)
In this example, we have created unit tests for the addition and division functions of our calculator code. By executing these test cases, we can verify that the calculator code performs accurately and efficiently.
Conclusion
Python is a versatile and powerful programming language that can be used to develop sophisticated calculator code for a wide range of applications. Whether it’s a simple calculator for basic arithmetic operations, an advanced calculator for complex mathematical functions, or a custom calculator for specialized calculations, Python provides the flexibility and capability to meet diverse requirements. With features such as error handling, unit testing, and GUI development, Python empowers developers to create calculator code that is robust, reliable, and user-friendly. By unleashing the power of Python, developers can elevate their calculator code to new heights and unlock endless possibilities for mathematical computation.
FAQs
Q: Is Python a suitable language for developing calculator code?
A: Yes, Python is well-suited for developing calculator code due to its simplicity, readability, and extensive libraries for mathematical computation.
Q: Can Python be used to create GUI-based calculator applications?
A: Absolutely! Python offers several GUI libraries such as Tkinter, PyQt, and Kivy, which can be used to develop intuitive and user-friendly calculator applications with graphical interfaces.
Q: How can I ensure the reliability of my calculator code in Python?
A: You can ensure the reliability of your calculator code in Python by implementing error handling mechanisms, conducting thorough unit testing, and adhering to best practices in software development.
Q: Are there any specific libraries or resources for developing financial calculator code in Python?
A: Yes, Python offers several libraries and resources for developing financial calculator code, such as NumPy for statistical calculations, and pandas for financial data analysis.