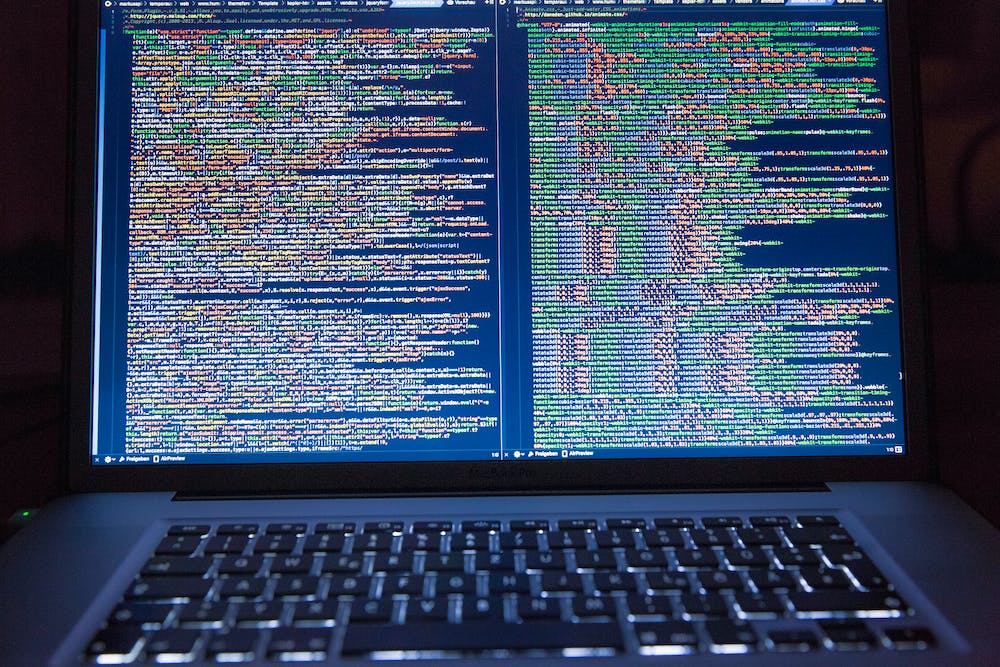
Introduction
PHP and MySQL are two popular technologies used for web development that work seamlessly together. PHP is a powerful and versatile scripting language, while MySQL is a widely used relational database management system. One of the key aspects of working with databases is the manipulation of data, and in this article, we will focus on mastering the PHP MySQL Insert operation to effortlessly insert data into a database.
Why is PHP MySQL Insert important?
PHP MySQL Insert is an essential operation when IT comes to managing and maintaining a database-driven Website. IT allows you to dynamically add new data to your database, whether IT‘s user registrations, blog posts, product details, or any other type of information you need to store. Without the ability to insert data, a database would be static and unable to evolve with the needs of your application.
Getting Started with PHP MySQL Insert
Before diving into the code, make sure you have a working environment with PHP and MySQL configured. Once your environment is set up, follow these steps:
- Create a database and a table: Start by creating a database and table structure that will store the data you want to insert. Use MySQL commands to create the necessary database and table.
- Establish a connection: In PHP, you need to establish a connection to your MySQL database using the appropriate credentials. This connection allows PHP to interact with the database and execute queries.
- Prepare an SQL Insert statement: The SQL Insert statement is used to define what data you want to insert into the database table. Make sure to provide the table name and the column names you want to insert data into.
- Execute the Insert statement: Use PHP to execute the SQL Insert statement. This step will add the data you want into the specified table. Make sure to handle any potential errors that may occur during the execution process.
- Clean up: After successfully inserting the data, close the database connection to free up resources.
Examples and Code Snippets
Let’s take a look at a simple example to illustrate the PHP MySQL Insert process. Assume we have a database called “exampleDB” with a table named “users” that has the following columns: “id”, “name”, and “email”. We want to insert a new user with the name “John Doe” and email “[email protected]”.
<?php
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$dbname = "exampleDB";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Prepare the Insert statement
$sql = "INSERT INTO users (name, email) VALUES ('John Doe', '[email protected]')";
// Execute the Insert statement
if ($conn->query($sql) === TRUE) {
echo "New user inserted successfully";
} else {
echo "Error: " . $sql . "
" . $conn->error;
}
// Close the connection
$conn->close();
?>
This example demonstrates the basic steps involved in performing a PHP MySQL Insert operation. The connection is established, the SQL Insert statement is prepared, executed, and finally, the connection is closed.
Conclusion
Mastering PHP MySQL Insert is crucial for anyone working with databases in web development. IT allows you to effortlessly insert data into your database, ensuring your site remains dynamic and adaptable. By following the steps outlined in this article, you can start harnessing the power of PHP and MySQL to easily manipulate data and create dynamic web applications.
FAQs
Q: Can I insert multiple rows of data using PHP MySQL Insert?
A: Yes, PHP MySQL Insert allows you to insert multiple rows of data in a single query. This can be achieved by constructing the SQL Insert statement with multiple sets of values.
Q: How can I retrieve the auto-generated ID after inserting a new row?
A: If your database table has an auto-incrementing ID column (typically set as the primary key), you can retrieve the auto-generated ID after inserting a new row. The mysqli_insert_id() function in PHP can be used to obtain the last inserted ID value.
Q: Are there any security considerations I should keep in mind when using PHP MySQL Insert?
A: Absolutely! When inserting data into your database, make sure to properly validate and sanitize user input to protect against SQL injection attacks. Use prepared statements or parameterized queries to prevent malicious data from affecting your database.