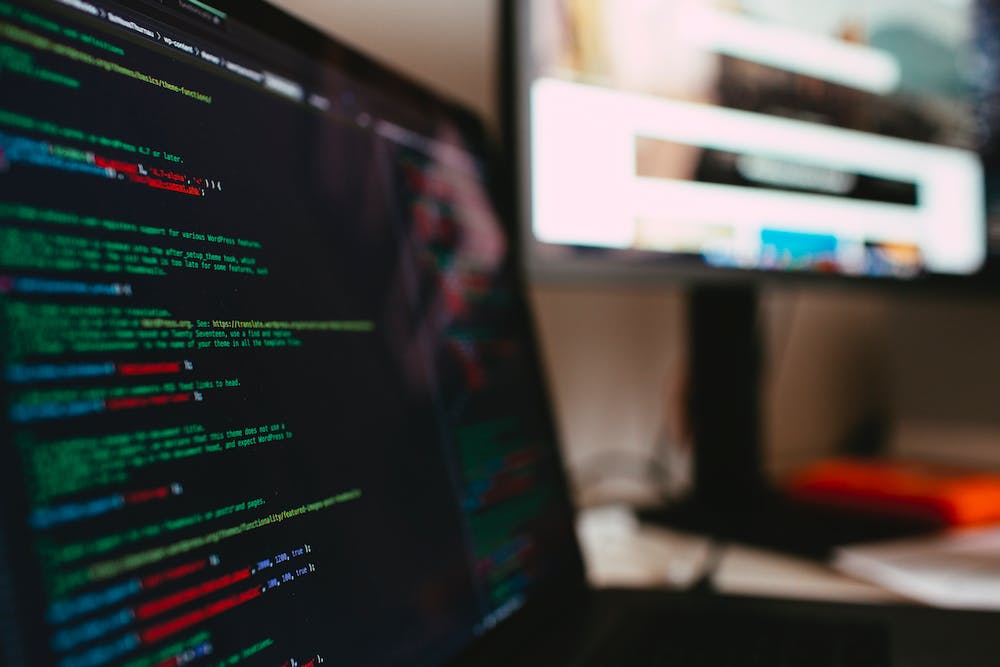
Introduction
PHP is a powerful scripting language that is widely used for web development. One of its core strengths lies in its ability to interact with databases, allowing developers to store and retrieve data efficiently. In this article, we will explore how to insert data into databases using PHP, providing you with a simple yet effective hack to get you started!
Understanding Databases
Before diving into the PHP implementation, IT‘s essential to understand the concept of databases. A database is a structured collection of data organized and accessed through a software system. IT allows for efficient storage, retrieval, and manipulation of information.
Common database management systems (DBMS) include MySQL, PostgreSQL, and SQLite. These systems provide SQL (Structured Query Language) to interact with the databases. PHP offers built-in support for various DBMS and provides functions to execute SQL queries programmatically.
Preparing the Database
Firstly, you need to set up your database using the chosen DBMS. For this example, let’s consider MySQL. Use a tool like phpMyAdmin or the MySQL command line to create a new database. For demonstration purposes, let’s call IT “employees”. Inside the database, create a table named “users” with columns such as “id,” “name,” and “email.”
Connecting to the Database
Using PHP, establishing a connection to the database is the first step. To connect to a MySQL database, you can use the built-in function called mysqli_connect(). Here’s an example:
$host = "localhost"; // Database host
$username = "your_username"; // Database username
$password = "your_password"; // Database password
$database = "employees"; // Database name
$connection = mysqli_connect($host, $username, $password, $database);
if (!$connection) {
die("Connection failed: " . mysqli_connect_error());
}
Inserting Data into the Database
After establishing a successful connection, you can proceed with inserting data into the database. The key function for this task is mysqli_query(). Here’s an example:
$name = $_POST['name']; // get the value of 'name' from a form, for example
$email = $_POST['email']; // get the value of 'email' from a form, for example
$query = "INSERT INTO users (name, email) VALUES ('$name', '$email')";
if (mysqli_query($connection, $query)) {
echo "Data inserted successfully!";
} else {
echo "Error inserting data: " . mysqli_error($connection);
}
In the above code snippet, we use the POST method to retrieve the values entered by the user in a form, but you can modify IT to suit your needs. The SQL query contains the INSERT statement, specifying the table and column names where the data should be inserted.
Closing the Connection
After executing the necessary database operations, IT‘s crucial to close the connection to free up resources. Use the mysqli_close() function to close the connection, as shown below:
mysqli_close($connection);
Conclusion
In this article, we have explored how to insert data into databases using the power of PHP. Through a step-by-step guide, we covered essential concepts such as connecting to the database, preparing the database, inserting data, and closing the connection. By mastering these techniques, you are now equipped to harness the capabilities of PHP for efficient data management.
FAQs
1. Is PHP the only way to interact with databases?
No, there are other programming languages like Python, Java, and .NET that can also interact with databases. However, PHP is particularly popular for web development due to its simple syntax and extensive libraries.
2. Are there any security concerns when inserting data into databases using PHP?
Yes, there are security considerations that need to be addressed. IT is essential to sanitize and validate user input to prevent SQL injection attacks. PHP provides functions like mysqli_real_escape_string() and prepared statements to mitigate these risks.
3. Can I insert multiple rows of data in a single query?
Yes, IT is possible to insert multiple rows of data in a single query. Instead of executing multiple INSERT statements, you can use the INSERT INTO…VALUES syntax and specify multiple sets of values within parentheses.
4. What other database operations can be performed using PHP?
PHP offers various database operations, including data retrieval (SELECT), data modification (UPDATE), data deletion (DELETE), and more. The power of PHP combined with SQL allows you to perform a wide range of operations on your database.