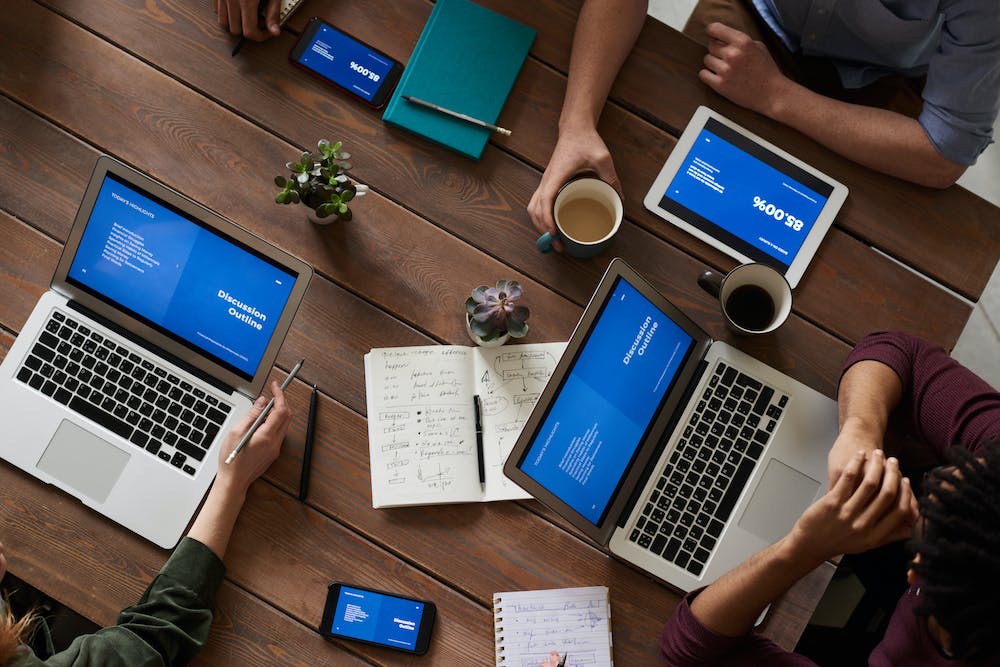
PHP is a powerful programming language that is widely used for web development. One of its key features is its ability to work with arrays, which are essential for storing and manipulating data. Learning how to echo array like a pro can significantly enhance your PHP skills and make you a more efficient developer. In this article, we will explore the fundamentals of echoing arrays in PHP and provide you with tips and examples to help you master this essential skill.
Understanding Arrays in PHP
Before we delve into how to echo arrays in PHP, let’s first understand what arrays are and how they work. In PHP, an array is a data structure that can store multiple values under a single variable name. These values can be of any data type, including strings, integers, or even other arrays. This makes arrays incredibly versatile and useful for organizing and managing data.
There are two main types of arrays in PHP: indexed arrays and associative arrays. Indexed arrays use numeric keys to access elements, while associative arrays use named keys. Let’s take a look at an example of each type of array:
“`php
// Indexed array
$cars = array(“Volvo”, “BMW”, “Toyota”);
// Associative array
$age = array(“Peter”=>”35”, “Ben”=>”37”, “Joe”=>”43”);
?>
“`
Echoing Arrays in PHP
Now that we have a basic understanding of arrays in PHP, let’s learn how to echo them. Echoing an array in PHP involves displaying its elements in a readable format, making IT easier for developers to understand and work with the data. There are several ways to echo arrays in PHP, each with its own advantages and use cases.
Using print_r()
One of the simplest ways to echo an array in PHP is by using the print_r()
function. This function is especially useful for debugging purposes, as it displays the entire contents of an array, including keys and values, in a human-readable format. Here’s an example:
“`php
$cars = array(“Volvo”, “BMW”, “Toyota”);
print_r($cars);
?>
“`
When you run this code, it will output the following:
“`
Array
(
[0] => Volvo
[1] => BMW
[2] => Toyota
)
“`
As you can see, print_r()
provides a clear and concise representation of the array’s contents, making it easy to understand and analyze.
Using var_dump()
Another useful function for echoing arrays in PHP is var_dump()
. This function displays the details of a variable, including its type and value, in a more detailed and technical format. Here’s how you can use it to echo an array:
“`php
$cars = array(“Volvo”, “BMW”, “Toyota”);
var_dump($cars);
?>
“`
Running this code will produce the following output:
“`
array(3) {
[0]=>
string(5) “Volvo”
[1]=>
string(3) “BMW”
[2]=>
string(6) “Toyota”
}
“`
Unlike print_r()
, var_dump()
provides additional information about the array, such as the data type and length of each element. This can be helpful for understanding the inner workings of the array and diagnosing any issues that may arise.
Using foreach Loop
While print_r()
and var_dump()
are great for debugging, they may not always provide the most user-friendly output for displaying arrays in a web page. In such cases, using a foreach
loop can be more effective for echoing arrays in PHP. This allows you to iterate through the elements of the array and display them in a customized format. Here’s an example:
“`php
$cars = array(“Volvo”, “BMW”, “Toyota”);
echo “
- “;
- $car
foreach ($cars as $car) {
echo “
“;
}
echo “
“;
?>
“`
When you run this code, it will generate an unordered list with each car name as a list item:
“`
– Volvo
– BMW
– Toyota
“`
Using a foreach
loop gives you more control over how the array is displayed, allowing you to format the output in a way that suits your specific requirements.
Tips for Echoing Arrays Like a Pro
Now that you have learned the basics of echoing arrays in PHP, here are some additional tips to help you master this skill and take your PHP development to the next level:
Use descriptive variable names
When working with arrays, it’s important to use clear and descriptive variable names that reflect the contents of the array. This makes it easier for you and other developers to understand the purpose of the array and its elements when echoing it.
Handle nested arrays carefully
If your array contains nested arrays, be mindful of how you echo them to avoid cluttering the output. Consider using recursive functions or multi-dimensional foreach
loops to neatly display nested arrays.
Consider the context of the output
Depending on where the array is being echoed, you may need to format the output differently. For example, echoing an array in a web page may require HTML formatting, while echoing in a console application may not.
Leverage PHP’s built-in functions
PHP offers a variety of built-in functions for manipulating and formatting arrays, such as implode()
and json_encode()
. Experiment with these functions to find the most efficient way to echo arrays based on your specific needs.
Conclusion
Learning how to echo arrays in PHP is a fundamental skill for any developer working with PHP. By mastering the techniques outlined in this article, you can enhance your ability to work with and display array data in a clear and efficient manner. Whether you are building web applications, APIs, or command-line tools, the power of echoing arrays in PHP is an essential tool in your development arsenal.
FAQs
Q: Can I use other functions to echo arrays in PHP?
A: Yes, there are several other functions available in PHP for echoing arrays, such as json_encode()
and implode()
. These functions offer different ways to format and display array data, so it’s worth experimenting with them to find the best approach for your specific use case.
Q: How can I echo a specific element from an array?
A: To echo a specific element from an array, you can use the array’s key to access the desired value. For example, if you have an associative array $age
with the keys “Peter”, “Ben”, and “Joe”, you can echo the age of “Peter” like this: echo $age['Peter'];
Q: Is there a limit to the size of arrays that can be echoed in PHP?
A: PHP does not impose a specific limit on the size of arrays that can be echoed. However, echoing large arrays with complex structures may impact performance and readability, so it’s important to consider the context and purpose of the array when echoing it.
By following the tips and techniques outlined in this article, you can unleash the power of PHP and become a pro at echoing arrays, making you a more efficient and skilled developer.