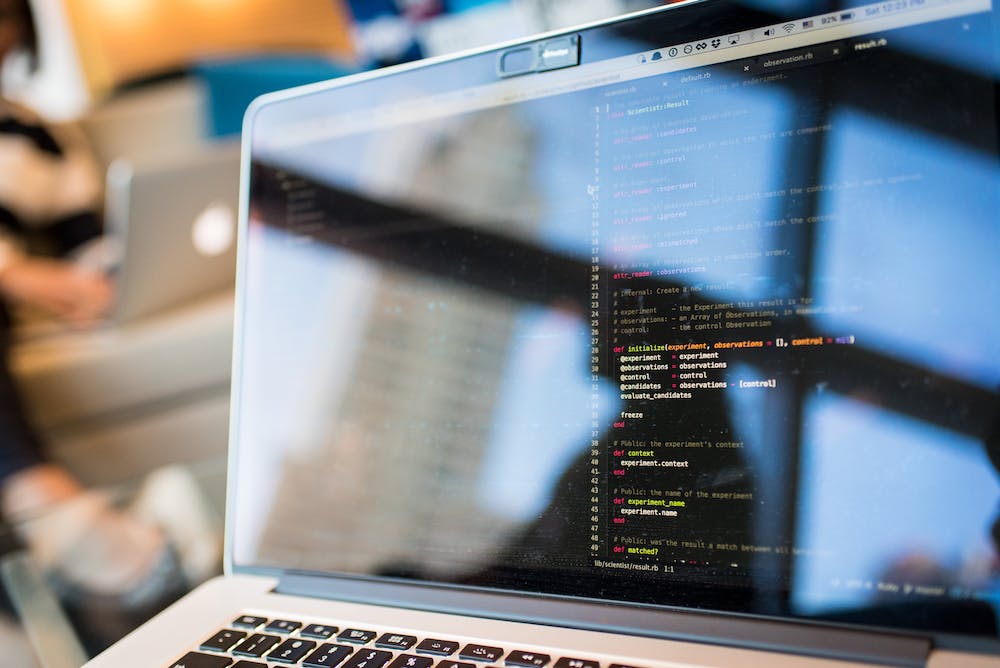
Introduction
File Transfer Protocol (FTP) is a standard network protocol used to transfer files between a client and a server on a computer network. IT is commonly used in web development for transferring files to and from a web server. PHP, being a powerful and popular server-side scripting language, provides built-in functions and libraries to interact with FTP servers.
Why PHP FTP?
PHP FTP allows web developers to automate file transfer tasks, manage remote files, and build powerful web applications that require seamless integration with FTP servers. Some of the key reasons to utilize PHP FTP in your web development projects are:
- Automated File Transfer: With PHP FTP, you can write scripts to automatically transfer files between different servers, saving time and effort.
- Remote File Management: PHP FTP provides functions to perform various operations on remote files, such as uploading, downloading, deleting, renaming, and changing permissions.
- Integration with Web Applications: PHP FTP can be integrated into your web applications to allow users to upload files, manage their files on the server, or synchronize data between different platforms.
- Background Processing: PHP FTP allows asynchronous processing, enabling background file transfers or batch processing without interrupting the main application flow.
Getting Started with PHP FTP
To start using PHP FTP, you need to ensure that the FTP extension is enabled in your PHP configuration. Most hosting providers have this extension enabled by default, but if you are running PHP on your local machine, you may need to enable IT manually by uncommenting the corresponding line in the php.ini file.
Once the FTP extension is enabled, you can establish connections, authenticate with the FTP server, and perform various file transfer operations using the PHP FTP functions.
Connection Establishment
To establish a connection with an FTP server, you can use the ftp_connect()
function. IT takes the server address as a parameter and returns a connection resource that can be used for subsequent operations.
<?php
$ftpServer = 'ftp.example.com';
$ftpConnection = ftp_connect($ftpServer);
if ($ftpConnection) {
echo 'Connected to the FTP server!';
} else {
echo 'Failed to connect to the FTP server.';
}
ftp_close($ftpConnection);
?>
Authentication
Once connected, you need to authenticate with the FTP server using your credentials. The ftp_login()
function is used for this purpose. IT takes the FTP connection resource, username, and password as parameters.
<?php
$ftpServer = 'ftp.example.com';
$ftpConnection = ftp_connect($ftpServer);
$ftpUsername = 'your-username';
$ftpPassword = 'your-password';
if (ftp_login($ftpConnection, $ftpUsername, $ftpPassword)) {
echo 'Authenticated successfully!';
} else {
echo 'Authentication failed.';
}
ftp_close($ftpConnection);
?>
File Transfer Operations
Once authenticated, you can perform various file transfer operations using the FTP connection resource and the PHP FTP functions. Here are a few examples:
- Uploading a file: Use the
ftp_put()
function to upload a local file to the FTP server. IT takes the FTP connection resource, destination path on the server, and source path of the local file as parameters. - Downloading a file: Use the
ftp_get()
function to download a file from the FTP server. IT takes the FTP connection resource, local file path for saving, and remote file path on the server as parameters. - Deleting a file: Use the
ftp_delete()
function to delete a file on the FTP server. IT takes the FTP connection resource and the file path on the server as parameters. - Renaming a file: Use the
ftp_rename()
function to rename a file on the FTP server. IT takes the FTP connection resource, current file path, and new file path as parameters. - Changing file permissions: Use the
ftp_chmod()
function to change file permissions on the FTP server. IT takes the FTP connection resource and the file path on the server along with the new permissions as parameters.
Conclusion
PHP FTP is a powerful tool for web developers, allowing seamless integration with FTP servers and enabling automation of file transfer tasks. By leveraging PHP FTP functions, you can easily manage remote files, build robust web applications, and enhance your development efficiency. Understanding how to establish connections, authenticate, and perform file transfer operations using PHP FTP can greatly benefit your web development projects.
FAQs
Q: Can PHP FTP be used for secure file transfers?
A: Yes, PHP FTP supports secure file transfers using FTP over TLS (FTPS) or Secure File Transfer Protocol (SFTP). You need to enable the appropriate extension and specify the secure connection parameters in your PHP scripts.
Q: Are there any alternatives to PHP FTP?
A: Yes, there are alternative libraries and packages available for handling FTP operations in PHP, such as phpseclib and cURL. These libraries provide additional features and capabilities beyond the built-in FTP functions in PHP.
Q: Can PHP FTP handle large file transfers?
A: Yes, PHP FTP can handle large file transfers. However, you may need to configure your PHP settings and server configurations to accommodate larger file sizes and longer execution times.
Q: Are there any security considerations when using PHP FTP?
A: When working with PHP FTP, IT is essential to ensure that your FTP server is properly secured and that your PHP scripts handle user authentication and input validation carefully to prevent unauthorized access and potential security vulnerabilities.