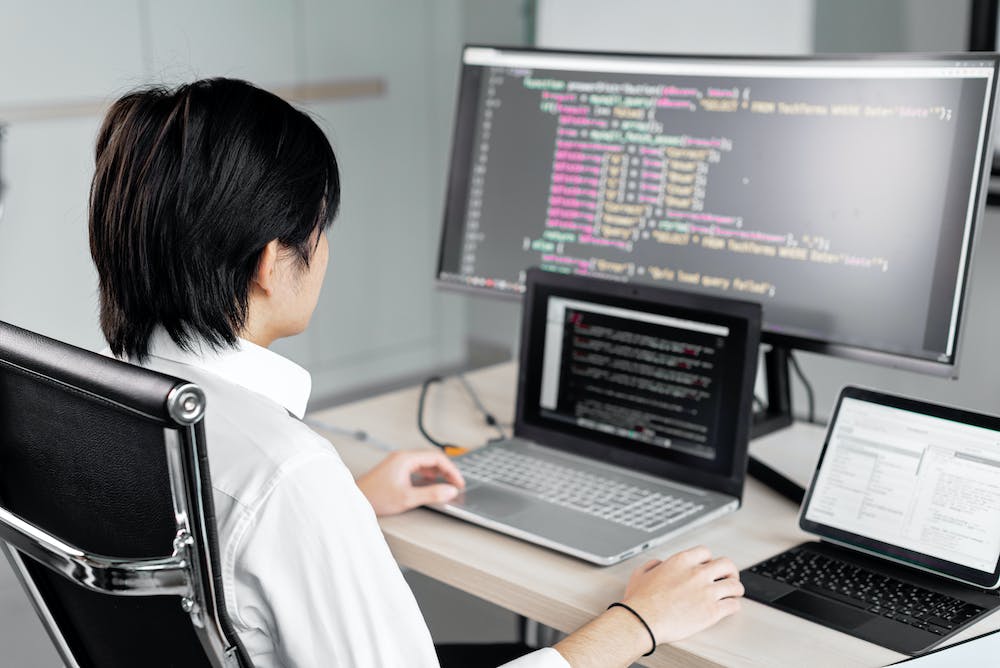
Numpy is a powerful library in Python that is widely used for numerical computations. IT provides support for high-performance multidimensional array objects and tools for working with these arrays. In this article, we will explore five mind-blowing tricks that will help you unleash the power of Numpy code and take your Python programming skills to the next level.
1. Broadcasting
Broadcasting is a powerful feature of Numpy that allows you to perform operations on arrays of different shapes. This can be incredibly useful when working with large datasets or when performing complex calculations. For example, you can add a scalar value to a Numpy array, and the operation will be applied to each element of the array, without the need for explicit looping or iteration.
Here’s an example:
import numpy as np
a = np.array([1, 2, 3, 4])
b = 2
c = a + b
print(c)
The output will be:
[3 4 5 6]
2. Universal Functions
Numpy provides a wide range of universal functions, or ufuncs, that allow you to perform element-wise operations on arrays. These functions are optimized for speed and efficiency, making them ideal for large-scale computations. Some common ufuncs include np.sin
, np.cos
, np.exp
, and np.log
.
Here’s an example:
import numpy as np
a = np.array([1, 2, 3, 4])
b = np.exp(a)
print(b)
The output will be:
[ 2.71828183 7.3890561 20.08553692 54.59815003]
3. Slicing and Indexing
Numpy allows for advanced slicing and indexing of arrays, making it easy to extract and manipulate subsets of data. You can use boolean arrays, integer arrays, and other advanced techniques to create powerful and efficient data manipulation operations.
Here’s an example:
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
b = a[a > 5]
print(b)
The output will be:
[6 7 8 9]
4. Vectorization
Vectorization is the process of converting a scalar operation into a vector operation, allowing for parallelization and increased performance. Numpy’s vectorized operations make it easy to perform complex calculations on large datasets with minimal code and maximum speed.
Here’s an example:
import numpy as np
a = np.array([1, 2, 3, 4])
b = np.array([5, 6, 7, 8])
c = a * b
print(c)
The output will be:
[ 5 12 21 32]
5. Linear Algebra Operations
Numpy provides comprehensive support for linear algebra operations, including matrix multiplication, matrix inversion, eigenvalue decomposition, and more. These operations are essential for many scientific and engineering applications, and Numpy’s efficient implementation makes it the go-to choice for these tasks.
Here’s an example:
import numpy as np
a = np.array([[1, 2], [3, 4]])
b = np.array([[5, 6], [7, 8]])
c = np.dot(a, b)
print(c)
The output will be:
[[19 22]
[43 50]]
Conclusion
Numpy is an essential library for anyone working with numerical data in Python. Its powerful array operations, universal functions, and support for linear algebra make it a versatile tool for a wide range of applications. By utilizing the tricks and techniques discussed in this article, you can take your Numpy coding skills to the next level and unlock the full potential of this amazing library.
FAQs
Q: Can I use Numpy for machine learning applications?
A: Yes, Numpy is widely used in machine learning for data manipulation, preprocessing, and numerical computations.
Q: Is Numpy open source?
A: Yes, Numpy is an open-source library released under a permissive license.
Q: How can I install Numpy?
A: You can install Numpy using pip, the Python package manager. Simply run the command pip install numpy
in your terminal or command prompt.