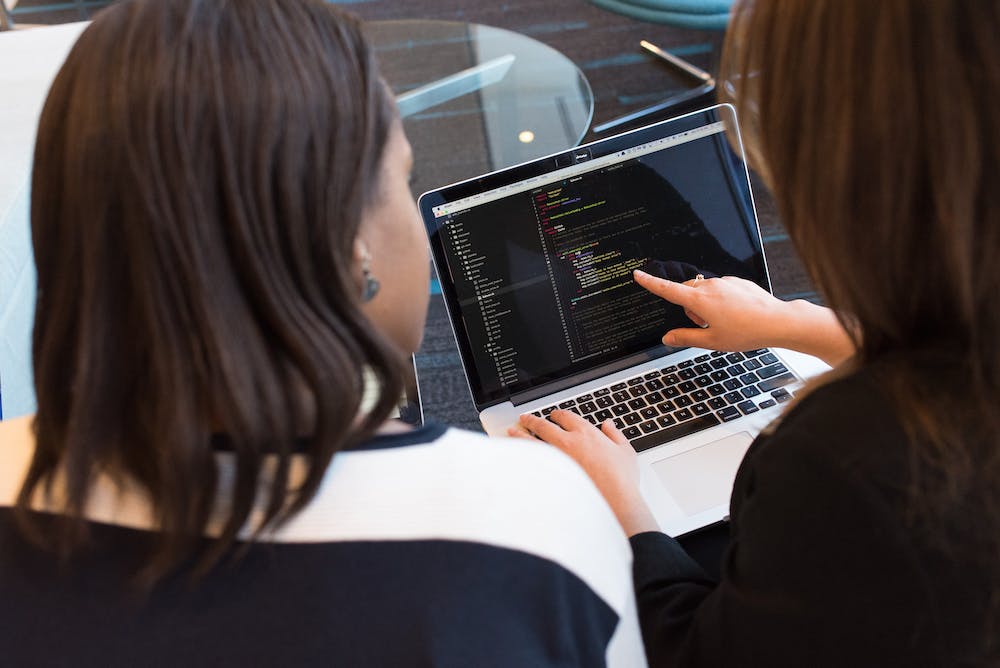
Laravel is a popular PHP framework known for its elegant syntax and powerful features. If you’re a Laravel developer, you’ve probably heard of GraphQL – a query language for your API that provides a more efficient, powerful, and flexible alternative to traditional REST APIs. In this ultimate guide, we’ll take a deep dive into how you can unleash the power of Laravel with GraphQL and take your web development skills to the next level.
What is GraphQL?
Before we dive into how to use GraphQL with Laravel, let’s first understand what GraphQL is all about. GraphQL is a query language for your API, and a runtime for executing those queries with your existing data. IT provides a more efficient, powerful, and flexible alternative to traditional REST APIs. With GraphQL, you can define the shape of the data you need and get exactly what you ask for. This reduces over-fetching and under-fetching of data and gives clients the power to ask for exactly what they need in a single request.
Why Use GraphQL with Laravel?
Now that you have a basic understanding of what GraphQL is, you might be wondering why you should use it with Laravel. There are several reasons why using GraphQL with Laravel can be beneficial:
- Efficient Data Fetching: With GraphQL, clients can specify the exact shape of the data they need, reducing over-fetching and under-fetching of data.
- Agility and Flexibility: GraphQL provides a flexible way to query for data, allowing clients to request exactly what they need without having to change the server-side code.
- Powerful Developer Tools: GraphQL comes with powerful developer tools that make it easy to explore the schema, test queries, and optimize performance.
- Single Endpoint: With GraphQL, you only need a single endpoint for all your data needs, making it easier to manage and consume data from your API.
Getting Started with GraphQL in Laravel
Now that you understand the benefits of using GraphQL with Laravel, let’s explore how you can get started with using GraphQL in your Laravel application. There are several packages available that make it easy to integrate GraphQL into your Laravel application, such as Lighthouse, GraphQL for Laravel, and Nuwave Lighthouse. For the purpose of this guide, we will use Lighthouse, a PHP package that lets you write GraphQL servers in PHP with ease.
Installation
First, you need to install Lighthouse using Composer:
composer require nuwave/lighthouse
Once Lighthouse is installed, you can publish its configuration file using the following command:
php artisan vendor:publish --provider="Nuwave\Lighthouse\LighthouseServiceProvider" --tag="config"
Next, you need to generate the schema and resolvers by running the following command:
php artisan lighthouse:demo
After running these commands, you’ll have a basic GraphQL setup in your Laravel application, and you can start defining your schema and resolvers.
Defining the Schema
The first step in using GraphQL with Laravel is to define your schema. In GraphQL, the schema defines the types of data that can be queried and the relationships between them. You can define your schema using the GraphQL Schema Definition Language (SDL), which provides a simple and expressive way to define your API’s types and the relationships between them.
Here’s an example of a simple schema definition for a blog application:
type Query {
posts: [Post!]!
post(id: ID!): Post
}
type Mutation {
createPost(title: String!, content: String!): Post!
}
type Post {
id: ID!
title: String!
content: String!
}
Once you’ve defined your schema, you can start writing resolvers to handle the queries and mutations defined in your schema.
Writing Resolvers
In GraphQL, a resolver is a function that’s responsible for fetching the data for a particular field in your schema. In Laravel, you can write resolvers as methods on a controller or a dedicated resolver class. Here’s an example of a resolver for the posts
query in the blog application schema:
class PostController
{
public function posts()
{
return Post::all();
}
}
Once you’ve written resolvers for all the queries and mutations in your schema, you can start serving your GraphQL API using Laravel’s built-in routing and controllers.
Optimizing Queries with GraphQL
Now that you have a basic GraphQL setup in your Laravel application, it’s important to optimize your queries to make sure they run efficiently. One way to optimize your GraphQL queries is by using dataloaders, which allow you to batch and cache database queries to prevent N+1 query problems. Another way to optimize your queries is by using GraphQL’s query optimization features, such as query caching and persisted queries, to reduce the overhead of parsing and executing queries on the server.
Conclusion
In this ultimate guide, we’ve explored how you can unleash the power of Laravel with GraphQL. We’ve learned what GraphQL is, why it’s beneficial to use with Laravel, and how to get started with using GraphQL in your Laravel application. We’ve also looked at how to define your schema, write resolvers, and optimize queries to make sure your GraphQL API runs efficiently. By using GraphQL with Laravel, you can take advantage of a more efficient, powerful, and flexible way to query for data in your web applications, and enhance the overall development experience for both developers and clients.
FAQs
Q: What are some popular GraphQL client libraries for consuming GraphQL APIs?
A: Some popular GraphQL client libraries for consuming GraphQL APIs include Apollo Client, Relay, and Urql.
Q: Can you have multiple GraphQL schemas in a single Laravel application?
A: Yes, you can have multiple GraphQL schemas in a single Laravel application by using packages such as Lighthouse, which provide the flexibility to define multiple schemas and resolvers.
Q: How does GraphQL differ from traditional REST APIs?
A: GraphQL provides a more efficient, powerful, and flexible alternative to traditional REST APIs by allowing clients to specify the exact shape of the data they need, reducing over-fetching and under-fetching of data, and providing a single endpoint for all data needs.