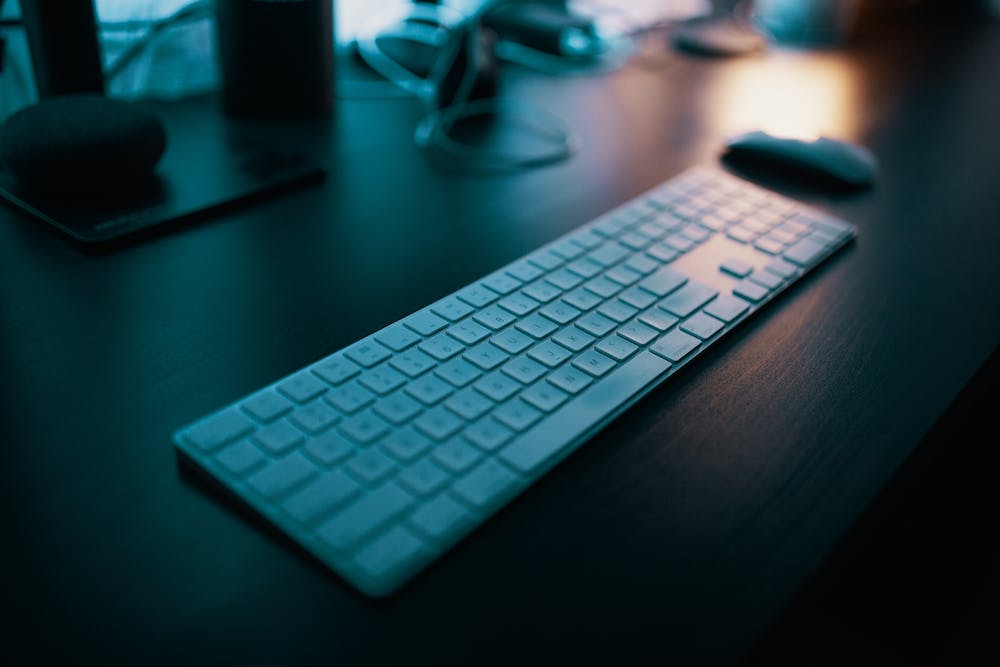
Laravel is a popular PHP framework known for its elegant syntax, expressive code, and powerful features. As a developer, mastering Laravel can significantly boost your development skills and make you more productive. One of the most powerful features of Laravel is the foreach loop, which allows you to iterate through arrays and collections with ease.
What is Foreach in Laravel?
The foreach loop in Laravel is used to iterate through arrays and collections. IT provides a convenient way to loop through each item in an array or collection and perform a certain action. The foreach loop is a powerful tool for developers and can be used in various scenarios to simplify the code and make it more readable.
How to Use Foreach in Laravel
Using the foreach loop in Laravel is quite simple. Here’s a basic example of how to use foreach to loop through an array of items:
“`php
$items = [‘apple’, ‘banana’, ‘orange’];
foreach ($items as $item) {
echo $item;
}
“`
In this example, we have an array of items and we use the foreach loop to iterate through each item and echo it to the screen. This is a basic example, but the foreach loop can be used in more complex scenarios as well.
When working with collections in Laravel, you can also use the foreach loop to iterate through the collection’s items. Here’s an example:
“`php
$users = User::all();
foreach ($users as $user) {
echo $user->name;
}
“`
In this example, we use the foreach loop to iterate through the collection of users and echo each user’s name to the screen. This is just one example of how the foreach loop can be used with collections in Laravel.
Why Use Foreach in Laravel?
There are several reasons why you should use the foreach loop in Laravel. Some of the key benefits include:
- Simplicity: The foreach loop is a simple and readable way to iterate through arrays and collections, making your code more understandable and maintainable.
- Flexibility: The foreach loop can be used with both arrays and collections, giving you the flexibility to work with different types of data structures in Laravel.
- Productivity: Using the foreach loop can help you write code more quickly and efficiently, saving you time and making you more productive as a developer.
Best Practices for Using Foreach in Laravel
While the foreach loop in Laravel is a powerful tool, it’s important to use it properly to avoid common pitfalls. Here are some best practices for using foreach in Laravel:
- Check for Empty Arrays/Collections: Before using a foreach loop, always check if the array or collection is empty to avoid errors.
- Avoid Modifying the Array/Collection: It’s generally not recommended to modify the array or collection within the foreach loop, as it can lead to unexpected results.
- Use Break and Continue Sparingly: While break and continue statements can be used within a foreach loop, they can make the code less readable and should be used sparingly.
Conclusion
The foreach loop in Laravel is a powerful tool for developers, allowing them to iterate through arrays and collections with ease. By mastering the foreach loop, you can boost your development skills and become more productive in Laravel development. Remember to use the foreach loop responsibly and follow best practices to avoid common pitfalls. With the power of foreach in Laravel, you can take your development skills to the next level and write elegant, expressive code.
FAQs
Q: What are the other loop alternatives in Laravel?
A: In addition to foreach, Laravel also supports other loop alternatives such as for and while loops. These loops can be used in different scenarios depending on the specific requirements of your code.
Q: Can foreach be used with associative arrays in Laravel?
A: Yes, foreach can be used with associative arrays in Laravel. When iterating through an associative array, the $key and $value (or any other variable name you choose) can represent the key-value pair for each item in the array.
Q: Is there a performance difference between foreach and other loops in Laravel?
A: In general, there is no significant performance difference between foreach and other loops in Laravel. The choice of loop depends on the specific requirements of your code and the readability of the code.
References
backlink works. “The Power of Foreach in Laravel”. https://www.backlinkworks.com/blog/power-of-foreach-laravel