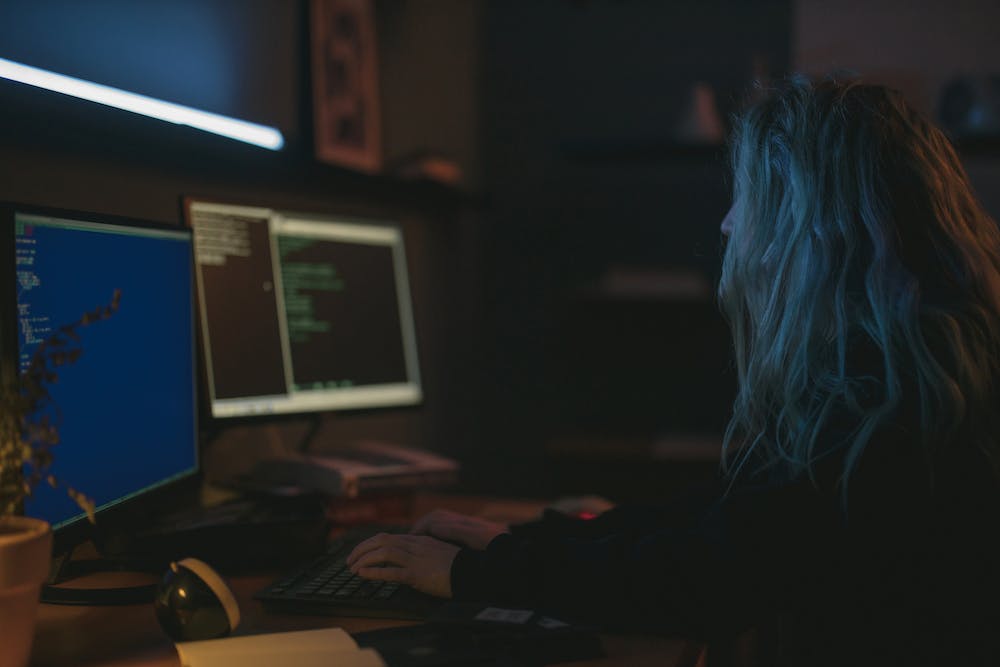
When IT comes to programming, decision-making plays a crucial role in controlling the flow of your code. Python, being one of the most popular programming languages today, offers a variety of tools and techniques to help developers make effective decisions within their programs. In this article, we will explore some mind-blowing Python code that can elevate your decision-making capabilities to new heights and empower you to create sophisticated and intelligent applications.
1. Conditional Statements
One of the fundamental ways to make decisions in Python is by using conditional statements. These statements allow you to perform different actions based on certain conditions. Let’s take a look at an example:
if temperature > 30:
print("IT's a hot day!")
elif temperature <= 30 and temperature > 20:
print("IT's a pleasant day!")
else:
print("IT's a cold day!")
In this code snippet, the temperature variable is checked against different conditions. If the temperature is greater than 30, IT will print “IT‘s a hot day!” If the temperature is between 20 and 30, IT will print “IT‘s a pleasant day!” Otherwise, IT will print “IT‘s a cold day!”
2. Ternary Operator
Python also offers a concise way to make decisions using the ternary operator. IT allows you to assign a value to a variable based on a condition. Here’s an example:
age = 25
is_adult = True if age >= 18 else False
In this example, the variable is_adult is assigned True if the age is greater than or equal to 18, otherwise IT is assigned False. This can be particularly useful in situations where you want to assign a value based on a condition without writing a full if-else statement.
3. Switch Case Using Dictionary
Python lacks a built-in switch case statement like some other programming languages. However, you can mimic its behavior using dictionaries. This technique can be handy when you have multiple cases to consider. Here’s an example:
def perform_operation(operator, num1, num2):
operations = {
'+': num1 + num2,
'-': num1 - num2,
'*': num1 * num2,
'/': num1 / num2,
}
return operations.get(operator, "Invalid operator")
In this function, the perform_operation takes an operator and two numbers as input. IT then checks the operator against the keys in the operations dictionary and returns the corresponding value. If the operator is not found in the dictionary, IT returns “Invalid operator”. This approach allows you to easily handle different cases without cluttering your code with multiple if-else statements.
Conclusion
Python provides several powerful tools for decision-making in your code. From conditional statements and ternary operators to dictionary-based switch cases, you have a wide range of options to choose from. By harnessing the power of these tools, you can make your code more intelligent, flexible, and efficient. Whether you’re building a simple script or a complex application, mastering decision-making in Python is essential.
FAQs
Q: Can I use multiple conditions in a single if statement?
A: Absolutely! Python allows you to use logical operators such as “and” and “or” to combine multiple conditions in a single if statement. For example: if a > 5 and b < 10:
Q: Is IT possible to nest if statements?
A: Yes, you can nest if statements within other if statements to create complex decision-making structures. However, be mindful of maintaining code readability and avoiding excessive nesting.
Q: Are there other approaches to mimic switch case behavior in Python?
A: Yes, apart from using dictionaries, you can also achieve switch case-like behavior by using if-elif-else statements or implementing classes and methods. The choice depends on the specific requirements of your code.
Q: Can I modify the value of a variable inside a ternary operator?
A: No, a ternary operator only allows you to assign a value to a variable based on a condition. If you need to modify the value of a variable, you should use regular if-else statements.
Q: How can I handle default cases in switch case-like dictionary implementations?
A: In the example provided, “Invalid operator” is returned when the operator is not found in the dictionary. You can customize this behavior by providing a default value or using try-except blocks for error handling.
Q: Are there any limitations to using switch case with dictionaries?
A: Switch case implementations using dictionaries work well for cases with constant values. If you require dynamic cases or complex conditions, using if-else statements might be a more suitable option.
Q: Where can I learn more about decision-making in Python?
A: There are several online resources and tutorials available that can help you dive deeper into decision-making techniques in Python. Some recommended sources include the official Python documentation, programming forums, and interactive coding platforms.
Q: Can decision-making techniques in Python be applied to other programming languages?
A: While the specific syntax may differ, the underlying concepts of decision-making are applicable to most programming languages. Understanding decision-making in Python can give you a solid foundation for making effective choices in other languages as well.