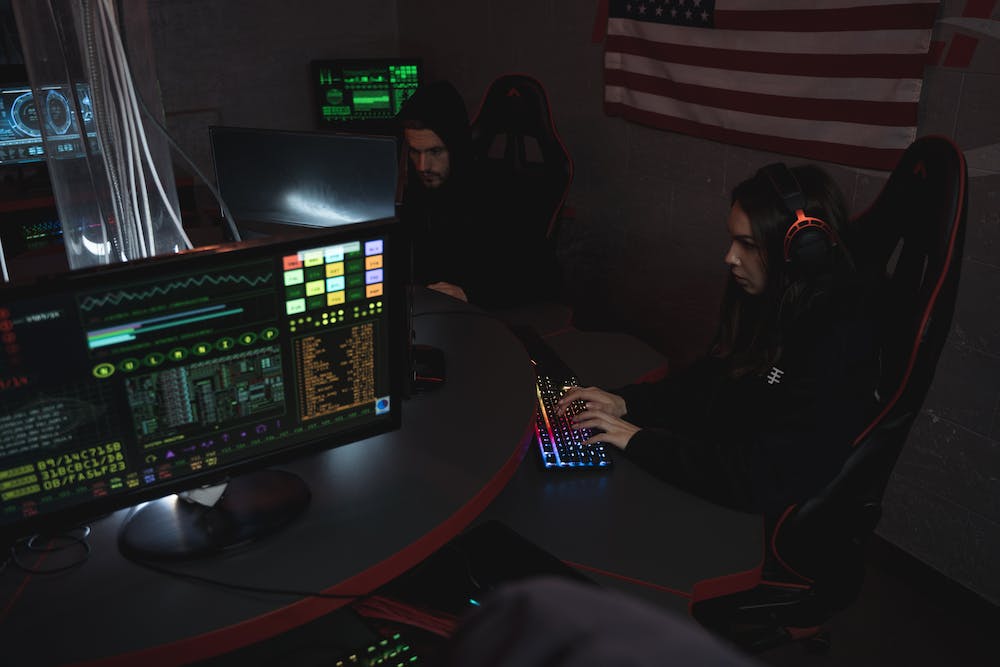
ASCII (American Standard Code for Information Interchange) is a character encoding standard used in computers and communication equipment to represent text and control characters. While IT may seem like a relic of the past, ASCII still plays a significant role in today’s technological landscape, especially in the world of programming and development.
Python, a high-level programming language known for its simplicity and ease of use, provides powerful tools to work with ASCII. In this article, we will explore how Python can unleash the power of ASCII through various code hacks and examples.
Understanding ASCII in Python
Before we delve into the code hacks, let’s first understand the basics of ASCII in Python. In Python, each character is represented by a numeric value according to the ASCII standard. For example, the letter ‘A’ is represented by the ASCII value 65, while the letter ‘a’ is represented by the ASCII value 97.
To work with ASCII in Python, we can use the built-in ord()
and chr()
functions. The ord()
function returns the ASCII value of a character, while the chr()
function returns the character corresponding to a given ASCII value.
Here’s an example of how to use these functions:
“`python
# Using ord() function
print(ord(‘A’)) # Output: 65
# Using chr() function
print(chr(65)) # Output: A
“`
Python Code Hacks for ASCII Manipulation
Now that we have a basic understanding of ASCII in Python, let’s explore some code hacks that can unleash its power:
1. Generating ASCII Table
Python allows us to generate a complete ASCII table using simple code. This can be useful for understanding the ASCII values of different characters and symbols.
“`python
# Generating ASCII table
for i in range(128):
print(f”{i} – {chr(i)}”)
“`
This code snippet will output the complete ASCII table from 0 to 127, along with the corresponding characters.
2. Converting String to ASCII
We can convert a string into its corresponding ASCII values using a list comprehension in Python. For example:
“`python
# Converting string to ASCII
text = “Hello, ASCII!”
ascii_values = [ord(char) for char in text]
print(ascii_values) # Output: [72, 101, 108, 108, 111, 44, 32, 65, 83, 67, 73, 73, 33]
“`
This code snippet will output the ASCII values of each character in the given string.
3. Creating ASCII Art
Python allows us to create ASCII art by mapping numeric values to corresponding characters. This can be used for creating visually appealing text-based graphics.
“`python
# Creating ASCII art
art = “”
for i in range(10):
for j in range(10):
art += chr(65 + (i+j)%26)
art += “\n”
print(art)
“`
This code snippet will create a simple ASCII art using alphabetic characters.
Unleashing the Power of ASCII for Data Encryption
ASCII can also be used for data encryption and encoding in Python. For example, we can use ASCII values to shift characters in a string to create a simple encryption algorithm.
“`python
# Data encryption using ASCII
def encrypt(text, shift):
encrypted_text = “”
for char in text:
if char.isalpha():
shifted = ord(char) + shift
if char.islower():
if shifted > ord(‘z’):
shifted -= 26
elif char.isupper():
if shifted > ord(‘Z’):
shifted -= 26
encrypted_text += chr(shifted)
else:
encrypted_text += char
return encrypted_text
original_text = “Hello, ASCII Encryption!”
shifted_text = encrypt(original_text, 3)
print(shifted_text) # Output: Khoor, DFVVFLH Hqfubsdu!
“`
In this example, we create a simple encryption algorithm by shifting each alphabetic character in the string by a given value. This can be useful for basic data encryption and encoding.
Conclusion
Python provides powerful tools for working with ASCII, allowing developers to unleash its power for various purposes such as data manipulation, encryption, and even creating visual art. By leveraging the built-in functions and code hacks provided by Python, developers can tap into the rich capabilities of ASCII to enhance their programming and development projects.
Whether it’s generating an ASCII table, converting strings to ASCII, creating ASCII art, or implementing data encryption, Python offers a wide range of possibilities for working with ASCII. By experimenting with these code hacks and examples, developers can gain a deeper understanding of ASCII and its potential applications in the world of programming.
FAQs
Q: What are some practical uses of ASCII in Python?
A: Some practical uses of ASCII in Python include data encryption, encoding, parsing, generating ASCII art, and working with text-based data. ASCII can also be used for character manipulation and analysis within strings.
Q: Can ASCII be used for data encoding and compression in Python?
A: Yes, ASCII can be used for data encoding and compression in Python by representing data as ASCII characters and implementing custom encoding algorithms for efficient storage and transmission.
Q: Are there any libraries or modules in Python specifically designed for working with ASCII?
A: While Python provides built-in functions for working with ASCII, there are also third-party libraries and modules such as ‘backlink works‘ that offer additional functionalities and tools for working with ASCII data and characters.