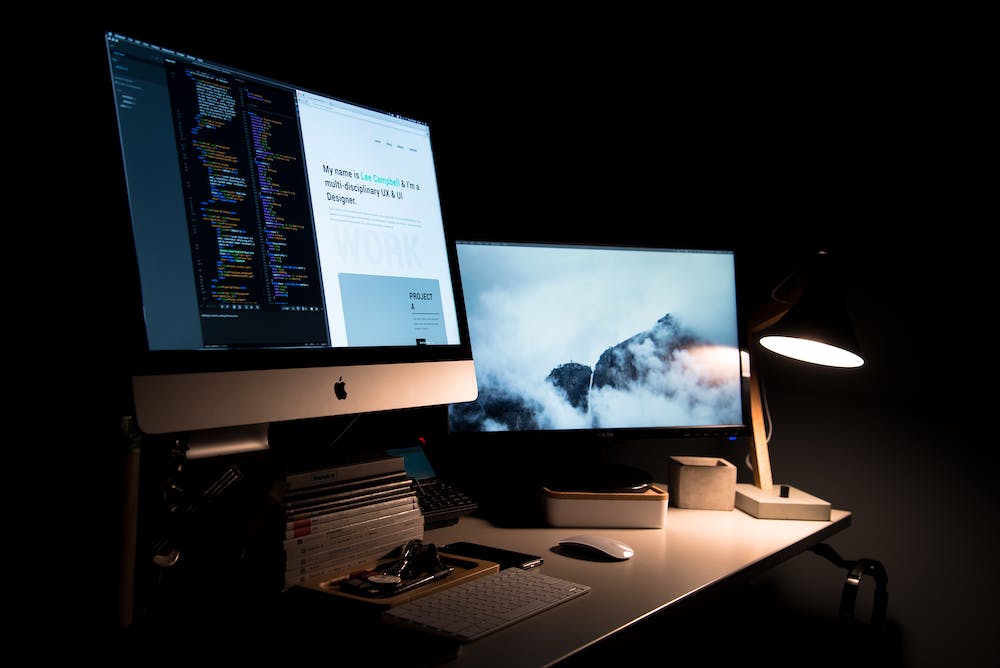
Unleash the Power of ASCII in Python! You Won’t Believe What You Can Do!
Introduction:
ASCII (American Standard Code for Information Interchange) is a character encoding standard that represents text in computers and other devices. IT uses numerical codes to represent characters, allowing computers to understand and display them. In Python, ASCII can be a powerful tool that opens up a world of possibilities for text manipulation, data analysis, and creative coding. In this article, we will explore the power of ASCII in Python and discover some amazing things that you can do with IT!
Understanding ASCII:
Before we dive into the exciting world of ASCII in Python, let’s quickly understand how ASCII works. ASCII assigns a unique code to each character, ranging from 0 to 127. This includes letters, numbers, punctuation marks, and special characters. For example, the ASCII code for the letter ‘A’ is 65, ‘B’ is 66, and so on. These codes can be represented in decimal, binary, or hexadecimal formats.
ASCII Art:
One of the most popular and fun applications of ASCII in Python is ASCII art. ASCII art is a technique that uses characters from the ASCII character set to create images and visual designs. By leveraging the ASCII codes and their corresponding characters, you can create stunning and intricate art pieces using just plain text. Here’s an example:
“`
# ASCII Art Example
print(” _”)
print(” / \ \ “)
print(” / _ \ \ “)
print(” | __/ |”)
print(” \_____/ “)
“`
When you run this code, you will see a simple representation of a house made using ASCII characters. You can go as complex and creative as you want with ASCII art, and IT‘s a fantastic way to showcase your coding skills and creativity.
Text Manipulation Using ASCII:
ASCII can also be used for text manipulation in Python. You can convert characters to their corresponding ASCII codes and perform operations on them. For instance, you can convert a lowercase letter to uppercase by simply subtracting the ASCII code difference between ‘a’ (97) and ‘A’ (65) from its ASCII code. Similarly, you can convert an uppercase letter to lowercase by adding the same ASCII code difference. Here’s an example:
“`python
# Text Manipulation Example
char = ‘a’
ascii_code = ord(char)
uppercase_ascii_code = ascii_code – 32
uppercase_char = chr(uppercase_ascii_code)
print(uppercase_char) # Output: ‘A’
“`
In this example, we convert the lowercase letter ‘a’ to ‘A’ by subtracting 32 from its ASCII code and then converting IT back to a character using `chr()` function. This technique can be useful in various situations where you need to manipulate text or perform case-insensitive operations.
Data Analysis Using ASCII:
ASCII can also be beneficial in data analysis tasks. For instance, you can use ASCII codes to represent categorical data and perform calculations on them. Let’s say you have a dataset with colors represented as strings. You can convert these color names to their ASCII codes and perform numerical operations or comparisons on them. Here’s an example:
“`python
# Data Analysis Example
colors = [‘red’, ‘blue’, ‘green’, ‘yellow’]
ascii_codes = [sum(ord(char) for char in color) for color in colors]
max_color = colors[ascii_codes.index(max(ascii_codes))]
print(max_color) # Output: ‘yellow’
“`
In this example, we calculate the sum of ASCII codes for each color name, and then we find the color with the highest sum. This technique allows you to perform data analysis on categorical data in a unique and creative way.
Conclusion:
ASCII is a powerful tool in Python that opens up countless possibilities for text manipulation, data analysis, and creative coding. From creating stunning ASCII art to manipulating text and performing calculations, ASCII unleashes the creative potential within your Python code. By leveraging the ASCII codes and their corresponding characters, you can create visually appealing designs, manipulate text effortlessly, and analyze categorical data in novel ways. So, don’t underestimate the power of ASCII in Python. IT‘s time to explore and unlock its full potential!
FAQs:
Q1. What are the limitations of ASCII?
A1. ASCII is limited to representing characters in the English language and does not include characters from other languages or symbols.
Q2. Are ASCII codes the same as Unicode?
A2. No, ASCII codes only represent characters in the ASCII character set, while Unicode is a superset of ASCII and includes characters from various languages and symbols.
Q3. Is ASCII case-sensitive?
A3. Yes, ASCII distinguishes between uppercase and lowercase characters, and their codes differ by a specific numerical value.
References:
– Python Documentation: “Built-in Types – String Methods” – https://docs.python.org/3/library/stdtypes.html#str
– Encyclopedia Britannica: “ASCII” – https://www.britannica.com/technology/ASCII