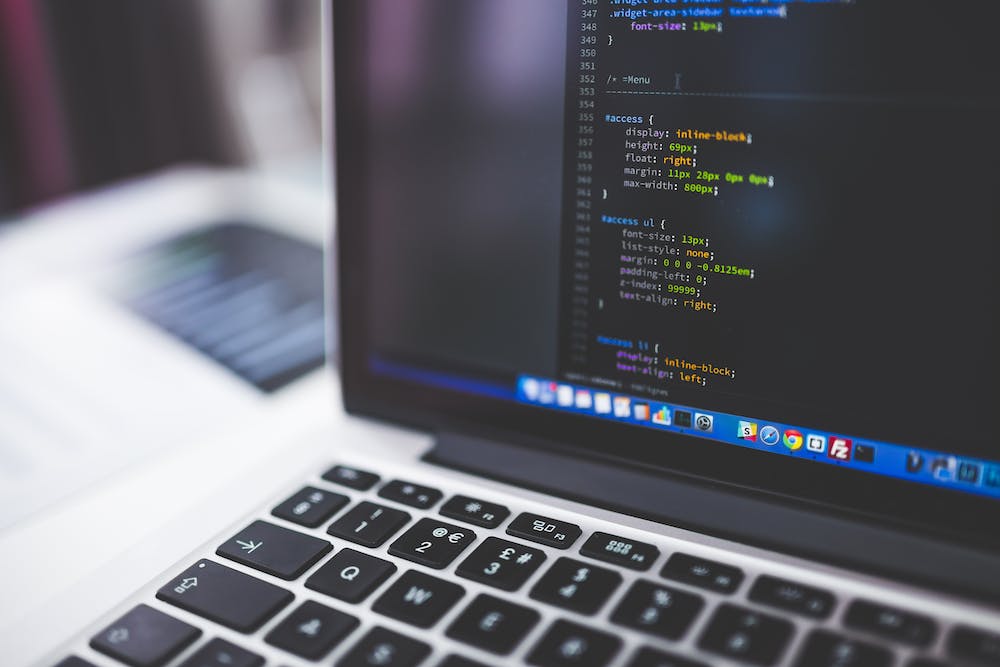
Understanding Unset PHP Function: A Comprehensive Guide
PHP is a widely used web development language that provides powerful functionalities to create dynamic and interactive websites. One of the essential functions in PHP is the unset() function, which allows developers to remove variables, objects, and array elements. In this comprehensive guide, we will explore the various aspects of the unset() function and its usage in PHP programming.
What is the unset() function?
The unset() function is a language construct in PHP that is primarily used to destroy the specified variables and free up the memory they occupy. When a variable is unset, IT is removed from the current scope, and its value becomes undefined. This function can be applied to variables, arrays, and objects. IT is important to note that unset() is not a function but a language construct, which means IT does not return a value.
Usage of the unset() function
The unset() function is quite versatile and can be used in several scenarios. Some common use cases include:
- Removing a Variable: When a variable is no longer needed or contains sensitive information, using unset() allows you to remove IT from the memory and make IT inaccessible. This can help enhance the security of your application.
- Deleting Array Elements: With unset(), you can delete specific elements from an array. This is particularly useful when you want to eliminate certain data from an array without modifying the keys or the order of the remaining elements.
- Destroying Objects: Objects in PHP take up memory space. By using unset() on an object, you can remove IT and free up the resources IT was utilizing.
Examples of using the unset() function:
1. Removing a Variable:
“`php
$myVariable = ‘Sensitive data’;
unset($myVariable);
// $myVariable is now undefined
“`
2. Deleting Array Elements:
“`php
$array = [“apple”, “banana”, “cherry”];
unset($array[1]);
// $array becomes [“apple”, “cherry”]
“`
3. Destroying Objects:
“`php
class MyClass {
// Class definition
}
$obj = new MyClass();
unset($obj);
// $obj is destroyed and no longer accessible
“`
Frequently Asked Questions (FAQs)
Q1. Can unset() be used on constants?
No, the unset() function cannot be used to unset constants. Constants, by their definition, are meant to remain unchanged throughout the execution of a script.
Q2. Is there a way to check if a variable has been unset?
Yes, you can use the isset() function to determine if a variable has been unset or not. The isset() function returns false if the variable is unset, and true otherwise.
Q3. Can the unset() function be used on multi-dimensional arrays?
Yes, the unset() function can be used on multi-dimensional arrays. You need to specify the keys of the elements you want to remove. However, IT is important to note that if you remove an element from an array, the keys of the remaining elements will not be re-ordered or re-indexed.
Conclusion
The unset() function is a powerful tool in PHP that allows developers to efficiently manage memory and remove variables, array elements, and objects. By utilizing this function, developers can enhance the security and performance of their PHP applications. Understanding the various use cases and limitations of unset() is crucial for effective PHP programming.