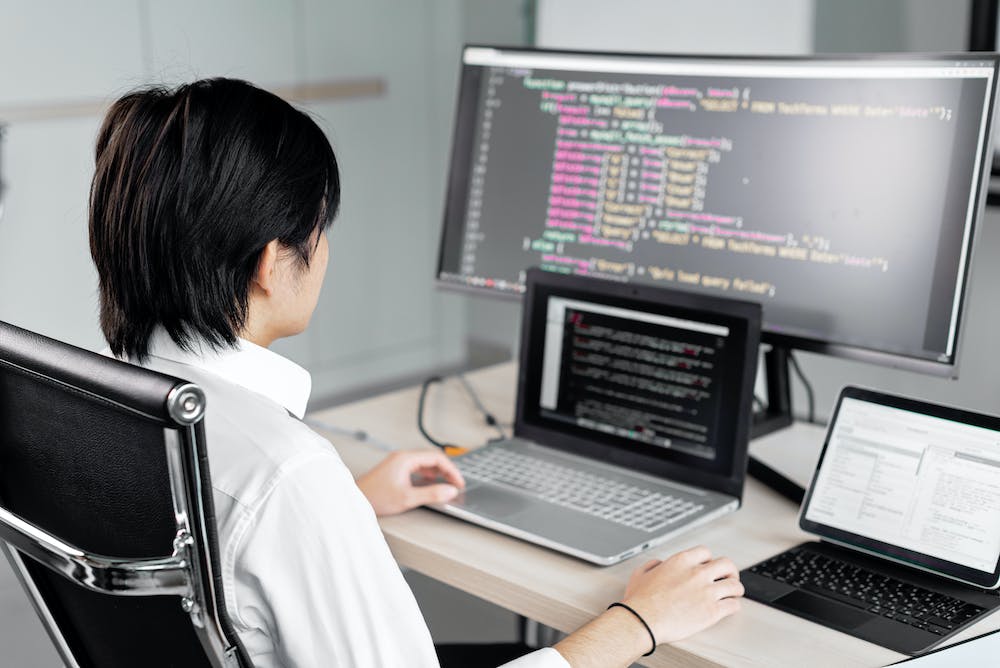
The PHP exit() function is a powerful tool that allows you to terminate the execution of a script and return a specified exit status. IT can be used in various scenarios to handle errors, control program flow, or simply exit a script once a certain condition is met. Understanding how to properly use the exit() function is crucial for any PHP developer, as IT can help ensure the integrity and reliability of your code.
The exit() function in PHP is a language construct rather than a regular function. This means that you can call IT with or without parentheses, depending on your preference. When called, the function immediately stops the execution of the script and sends a specified exit status to the server. This exit status can be used to communicate various outcomes, such as success, failure, or specific error codes.
One of the most common use cases for the exit() function is error handling. When an error occurs in your script, you can use the exit() function to immediately halt the execution and display a user-friendly error message. For example:
if (!$result) {
echo "Error: Failed to retrieve data.";
exit(); // Terminate the script
}
In this example, if the variable $result evaluates to false, the script will display an error message and exit. This prevents any further code from executing and ensures that the user is aware of the failure.
Another use case for the exit() function is controlling program flow based on specific conditions. For instance, you may want to exit a script if a certain condition is met, such as when a user is not logged in:
if (!isset($_SESSION['user'])) {
header("Location: login.php");
exit(); // Terminate the script
}
In this example, if the session variable “user” is not set, the script redirects the user to a login page and exits. This prevents any unauthorized access to restricted pages.
The exit() function can also be used to communicate specific exit statuses to the server. By default, when you call exit() without any arguments, IT returns an exit status of 0, indicating successful termination. However, you can specify a different exit status as an argument. This can be useful in scenarios where you want to differentiate between different types of errors or outcomes:
if ($errorCondition) {
exit(1); // Terminate the script with error status 1
}
In this example, if the variable $errorCondition evaluates to true, the script will exit with an error status of 1, indicating a specific type of error. You can use different exit status codes to differentiate between various error conditions and handle them accordingly.
FAQs
Q: Can exit() be used within a function?
A: Yes, the exit() function can be used within a function to terminate the execution of that function. However, IT will also stop the execution of the entire script if called within the main script.
Q: Does exit() close database connections or release resources?
A: No, the exit() function immediately terminates the script without any further cleanup. IT does not close database connections or release any resources. IT is recommended to handle these tasks separately before calling exit().
Q: Is there a difference between exit() and die()?
A: No, exit() and die() are equivalent and can be used interchangeably. They both serve the same purpose of terminating the script and returning an exit status.
Q: Can the exit status be captured and used by the calling code?
A: Yes, the exit status can be captured and used by the calling code, for example, to determine the success or failure of the executed script. In PHP, you can use the shell_exec() function to capture the exit status of a command executed with exec().
Q: Is IT good practice to use exit() for normal program flow control?
A: No, IT is generally not considered good practice to use exit() for normal program flow control. IT is primarily intended for error handling or exceptional cases. Using IT excessively or inappropriately can make code harder to debug and maintain.
In conclusion, the PHP exit() function is a powerful tool for controlling program flow, handling errors, and terminating script execution. By understanding its usage and following best practices, you can ensure the reliability and integrity of your PHP code.