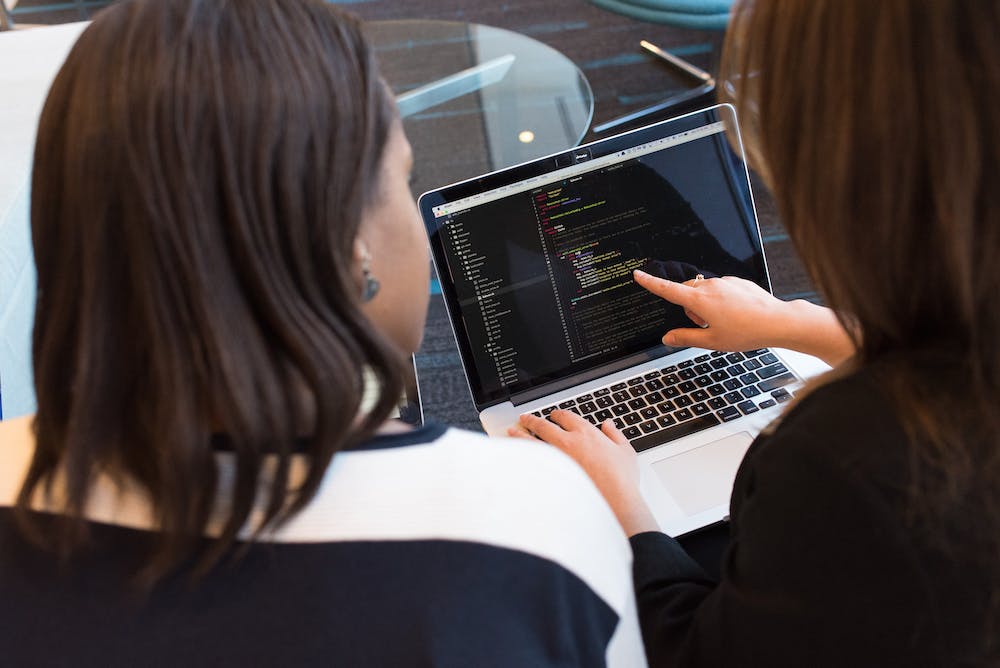
The PHP empty()
function is a useful tool for determining if a variable is considered empty. Whether you are a beginner or an experienced PHP developer, understanding how to use this function effectively is crucial. In this comprehensive guide, we will delve into the intricacies of the empty()
function, covering its basic usage, complex scenarios, and common pitfalls. By the end of this article, you will have a solid understanding of how to utilize this function in your PHP code.
Basic Usage
At its core, the empty()
function is designed to check whether a variable is considered empty. IT does so by considering a variable to be empty if IT is:
- An empty string (
""
), zero (0
), or a string with only whitespace characters (like" "
) - NULL or undefined
- An empty array (
[]
) - A variable that has not been set at all
To use the empty()
function, simply pass the variable you want to check as its parameter. Let’s look at a few examples:
$name = "";
if (empty($name)) {
echo "The name is empty.";
}
$count = 0;
if (empty($count)) {
echo "The count is empty.";
}
$numbers = [];
if (empty($numbers)) {
echo "The array is empty.";
}
$undefinedVariable;
if (empty($undefinedVariable)) {
echo "The variable is empty.";
}
In the above code snippet, the empty()
function is used to check if the $name
, $count
, $numbers
, and $undefinedVariable
variables are empty. If they are empty, the corresponding message is printed.
Complex Scenarios
While the empty()
function is straightforward in basic scenarios, IT becomes more nuanced in some complex cases. For instance, IT might not behave as you expect when your variable contains certain values.
One specific case to be aware of is when your variable contains the string “0”. Although IT is technically a non-empty string, the empty()
function considers IT to be empty due to its zero-like nature. Let’s take a look at an example:
$value = "0";
if (empty($value)) {
echo "The value is empty.";
} else {
echo "The value is not empty.";
}
In this case, the output will be “The value is empty.” This behavior can be surprising if you are expecting “The value is not empty.” Instead of using empty()
, you may consider using the isset()
function to check if a variable is set, which does not consider “0” as empty.
Another scenario where you need to be cautious is with variables that contain whitespace characters. As mentioned earlier, a variable with only whitespace characters is considered empty. Here’s an example that showcases this:
$whitespace = " ";
if (empty($whitespace)) {
echo "The whitespace variable is empty.";
} else {
echo "The whitespace variable is not empty.";
}
In this case, the output will be “The whitespace variable is empty.” Even though the $whitespace
variable contains spaces, IT is considered empty due to the absence of other non-whitespace characters.
Common Pitfalls
There are a few common pitfalls that developers sometimes encounter when using the empty()
function.
The first one is attempting to use empty()
with a non-existent variable directly. Consider the following code:
if (empty($nonExistentVariable)) {
echo "The variable is empty.";
} else {
echo "The variable is not empty.";
}
If you run this code, you will receive an error similar to “Undefined variable: nonExistentVariable“. To avoid this, ensure that the variable you are checking with empty()
exists or use isset()
for such scenarios.
Another common pitfall is mistakenly using the empty()
function on non-variable entities, such as function calls or expressions. In such cases, PHP will raise a warning because empty()
expects a variable parameter. Here’s an example to illustrate this:
if (empty(getName())) {
echo "The name is empty.";
}
function getName() {
return "John Doe";
}
Executing this code will result in a “Warning: empty() expects parameter 1 to be variable” warning. Ensure that you pass a variable to empty()
instead of a function call or expression.
FAQs
Q: What’s the difference between empty()
and isset()
?
A: While empty()
checks if a variable is empty, isset()
determines if a variable is set and not NULL. In other words, isset()
will return false
if the variable is either NULL or not set, whereas empty()
will return true
in those cases. Additionally, empty()
considers an empty string, 0, an empty array, and a variable that has not been set as empty, while isset()
does not.
Q: Is there an opposite to the empty()
function?
A: Yes, the opposite of the empty()
function is the !empty()
expression. IT returns true
if the variable is not empty, allowing you to perform further actions based on that condition.
Q: How is empty()
useful in form validation?
A: The empty()
function is commonly used in form validation to check if required fields are filled out. By running empty()
on the submitted form input, you can easily determine if the user has left a required field empty. If the field is empty, you can display an error message and prevent the form from being submitted until all required fields are filled.
Q: Can empty()
be used with objects?
A: No, empty()
cannot be used directly with objects. IT only works with variables. To check if an object is empty, you can use other methods like checking if IT has any properties or if IT meets specific criteria.
Now that you have a comprehensive understanding of the PHP empty()
function, you can confidently utilize IT to check the emptiness of variables in your PHP applications. Remember to consider complex scenarios, avoid common pitfalls, and choose the appropriate function for your specific use cases.